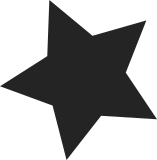
In this ioctl interface, processing the command starts from properties of the command and fetching the appropriate user objects before calling the handler. Parsing and validation is done according to a specifier declared by the driver's code. In the driver, all supported objects are declared. These objects are separated to different object namepsaces. Dividing objects to namespaces is done at initialization by using the higher bits of the object ids. This initialization can mix objects declared in different places to one parsing tree using in this ioctl interface. For each object we list all supported methods. Similarly to objects, methods are separated to method namespaces too. Namespacing is done similarly to the objects case. This could be used in order to add methods to an existing object. Each method has a specific handler, which could be either a default handler or a driver specific handler. Along with the handler, a bunch of attributes are specified as well. Similarly to objects and method, attributes are namespaced and hashed by their ids at initialization too. All supported attributes are subject to automatic fetching and validation. These attributes include the command, response and the method's related objects' ids. When these entities (objects, methods and attributes) are used, the high bits of the entities ids are used in order to calculate the hash bucket index. Then, these high bits are masked out in order to have a zero based index. Since we use these high bits for both bucketing and namespacing, we get a compact representation and O(1) array access. This is mandatory for efficient dispatching. Each attribute has a type (PTR_IN, PTR_OUT, IDR and FD) and a length. Attributes could be validated through some attributes, like: (*) Minimum size / Exact size (*) Fops for FD (*) Object type for IDR If an IDR/fd attribute is specified, the kernel also states the object type and the required access (NEW, WRITE, READ or DESTROY). All uobject/fd management is done automatically by the infrastructure, meaning - the infrastructure will fail concurrent commands that at least one of them requires concurrent access (WRITE/DESTROY), synchronize actions with device removals (dissociate context events) and take care of reference counting (increase/decrease) for concurrent actions invocation. The reference counts on the actual kernel objects shall be handled by the handlers. objects +--------+ | | | | methods +--------+ | | ns method method_spec +-----+ |len | +--------+ +------+[d]+-------+ +----------------+[d]+------------+ |attr1+-> |type | | object +> |method+-> | spec +-> + attr_buckets +-> |default_chain+--> +-----+ |idr_type| +--------+ +------+ |handler| | | +------------+ |attr2| |access | | | | | +-------+ +----------------+ |driver chain| +-----+ +--------+ | | | | +------------+ | | +------+ | | | | | | | | | | | | | | | | | | | | +--------+ [d] = Hash ids to groups using the high order bits The right types table is also chosen by using the high bits from the ids. Currently we have either default or driver specific groups. Once validation and object fetching (or creation) completed, we call the handler: int (*handler)(struct ib_device *ib_dev, struct ib_uverbs_file *ufile, struct uverbs_attr_bundle *ctx); ctx bundles attributes of different namespaces. Each element there is an array of attributes which corresponds to one namespaces of attributes. For example, in the usually used case: ctx core +----------------------------+ +------------+ | core: +---> | valid | +----------------------------+ | cmd_attr | | driver: | +------------+ |----------------------------+--+ | valid | | | cmd_attr | | +------------+ | | valid | | | obj_attr | | +------------+ | | drivers | +------------+ +> | valid | | cmd_attr | +------------+ | valid | | cmd_attr | +------------+ | valid | | obj_attr | +------------+ Signed-off-by: Matan Barak <matanb@mellanox.com> Reviewed-by: Yishai Hadas <yishaih@mellanox.com> Signed-off-by: Doug Ledford <dledford@redhat.com>
121 lines
5.2 KiB
C
121 lines
5.2 KiB
C
/*
|
|
* Copyright (c) 2005 Topspin Communications. All rights reserved.
|
|
* Copyright (c) 2005, 2006 Cisco Systems. All rights reserved.
|
|
* Copyright (c) 2005-2017 Mellanox Technologies. All rights reserved.
|
|
* Copyright (c) 2005 Voltaire, Inc. All rights reserved.
|
|
* Copyright (c) 2005 PathScale, Inc. All rights reserved.
|
|
*
|
|
* This software is available to you under a choice of one of two
|
|
* licenses. You may choose to be licensed under the terms of the GNU
|
|
* General Public License (GPL) Version 2, available from the file
|
|
* COPYING in the main directory of this source tree, or the
|
|
* OpenIB.org BSD license below:
|
|
*
|
|
* Redistribution and use in source and binary forms, with or
|
|
* without modification, are permitted provided that the following
|
|
* conditions are met:
|
|
*
|
|
* - Redistributions of source code must retain the above
|
|
* copyright notice, this list of conditions and the following
|
|
* disclaimer.
|
|
*
|
|
* - Redistributions in binary form must reproduce the above
|
|
* copyright notice, this list of conditions and the following
|
|
* disclaimer in the documentation and/or other materials
|
|
* provided with the distribution.
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
|
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
|
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
|
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS
|
|
* BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN
|
|
* ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
|
|
* CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
|
* SOFTWARE.
|
|
*/
|
|
|
|
#ifndef RDMA_CORE_H
|
|
#define RDMA_CORE_H
|
|
|
|
#include <linux/idr.h>
|
|
#include <rdma/uverbs_types.h>
|
|
#include <rdma/uverbs_ioctl.h>
|
|
#include <rdma/ib_verbs.h>
|
|
#include <linux/mutex.h>
|
|
|
|
int uverbs_ns_idx(u16 *id, unsigned int ns_count);
|
|
const struct uverbs_object_spec *uverbs_get_object(const struct ib_device *ibdev,
|
|
uint16_t object);
|
|
const struct uverbs_method_spec *uverbs_get_method(const struct uverbs_object_spec *object,
|
|
uint16_t method);
|
|
/*
|
|
* These functions initialize the context and cleanups its uobjects.
|
|
* The context has a list of objects which is protected by a mutex
|
|
* on the context. initialize_ucontext should be called when we create
|
|
* a context.
|
|
* cleanup_ucontext removes all uobjects from the context and puts them.
|
|
*/
|
|
void uverbs_cleanup_ucontext(struct ib_ucontext *ucontext, bool device_removed);
|
|
void uverbs_initialize_ucontext(struct ib_ucontext *ucontext);
|
|
|
|
/*
|
|
* uverbs_uobject_get is called in order to increase the reference count on
|
|
* an uobject. This is useful when a handler wants to keep the uobject's memory
|
|
* alive, regardless if this uobject is still alive in the context's objects
|
|
* repository. Objects are put via uverbs_uobject_put.
|
|
*/
|
|
void uverbs_uobject_get(struct ib_uobject *uobject);
|
|
|
|
/*
|
|
* In order to indicate we no longer needs this uobject, uverbs_uobject_put
|
|
* is called. When the reference count is decreased, the uobject is freed.
|
|
* For example, this is used when attaching a completion channel to a CQ.
|
|
*/
|
|
void uverbs_uobject_put(struct ib_uobject *uobject);
|
|
|
|
/* Indicate this fd is no longer used by this consumer, but its memory isn't
|
|
* necessarily released yet. When the last reference is put, we release the
|
|
* memory. After this call is executed, calling uverbs_uobject_get isn't
|
|
* allowed.
|
|
* This must be called from the release file_operations of the file!
|
|
*/
|
|
void uverbs_close_fd(struct file *f);
|
|
|
|
/*
|
|
* Get an ib_uobject that corresponds to the given id from ucontext, assuming
|
|
* the object is from the given type. Lock it to the required access when
|
|
* applicable.
|
|
* This function could create (access == NEW), destroy (access == DESTROY)
|
|
* or unlock (access == READ || access == WRITE) objects if required.
|
|
* The action will be finalized only when uverbs_finalize_object or
|
|
* uverbs_finalize_objects are called.
|
|
*/
|
|
struct ib_uobject *uverbs_get_uobject_from_context(const struct uverbs_obj_type *type_attrs,
|
|
struct ib_ucontext *ucontext,
|
|
enum uverbs_obj_access access,
|
|
int id);
|
|
int uverbs_finalize_object(struct ib_uobject *uobj,
|
|
enum uverbs_obj_access access,
|
|
bool commit);
|
|
/*
|
|
* Note that certain finalize stages could return a status:
|
|
* (a) alloc_commit could return a failure if the object is committed at the
|
|
* same time when the context is destroyed.
|
|
* (b) remove_commit could fail if the object wasn't destroyed successfully.
|
|
* Since multiple objects could be finalized in one transaction, it is very NOT
|
|
* recommended to have several finalize actions which have side effects.
|
|
* For example, it's NOT recommended to have a certain action which has both
|
|
* a commit action and a destroy action or two destroy objects in the same
|
|
* action. The rule of thumb is to have one destroy or commit action with
|
|
* multiple lookups.
|
|
* The first non zero return value of finalize_object is returned from this
|
|
* function. For example, this could happen when we couldn't destroy an
|
|
* object.
|
|
*/
|
|
int uverbs_finalize_objects(struct uverbs_attr_bundle *attrs_bundle,
|
|
struct uverbs_attr_spec_hash * const *spec_hash,
|
|
size_t num,
|
|
bool commit);
|
|
|
|
#endif /* RDMA_CORE_H */
|