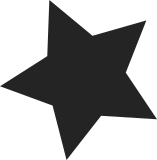
Based on 1 normalized pattern(s): this program is free software you can redistribute it and or modify it under the terms of the gnu general public license as published by the free software foundation either version 2 of the license or at your option any later version extracted by the scancode license scanner the SPDX license identifier GPL-2.0-or-later has been chosen to replace the boilerplate/reference in 3029 file(s). Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Reviewed-by: Allison Randal <allison@lohutok.net> Cc: linux-spdx@vger.kernel.org Link: https://lkml.kernel.org/r/20190527070032.746973796@linutronix.de Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
118 lines
2.7 KiB
C
118 lines
2.7 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/*
|
|
* Driver for generic Bluetooth SCO link
|
|
* Copyright 2011 Lars-Peter Clausen <lars@metafoo.de>
|
|
*/
|
|
|
|
#include <linux/init.h>
|
|
#include <linux/module.h>
|
|
#include <linux/platform_device.h>
|
|
|
|
#include <sound/soc.h>
|
|
|
|
static const struct snd_soc_dapm_widget bt_sco_widgets[] = {
|
|
SND_SOC_DAPM_INPUT("RX"),
|
|
SND_SOC_DAPM_OUTPUT("TX"),
|
|
};
|
|
|
|
static const struct snd_soc_dapm_route bt_sco_routes[] = {
|
|
{ "Capture", NULL, "RX" },
|
|
{ "TX", NULL, "Playback" },
|
|
};
|
|
|
|
static struct snd_soc_dai_driver bt_sco_dai[] = {
|
|
{
|
|
.name = "bt-sco-pcm",
|
|
.playback = {
|
|
.stream_name = "Playback",
|
|
.channels_min = 1,
|
|
.channels_max = 1,
|
|
.rates = SNDRV_PCM_RATE_8000,
|
|
.formats = SNDRV_PCM_FMTBIT_S16_LE,
|
|
},
|
|
.capture = {
|
|
.stream_name = "Capture",
|
|
.channels_min = 1,
|
|
.channels_max = 1,
|
|
.rates = SNDRV_PCM_RATE_8000,
|
|
.formats = SNDRV_PCM_FMTBIT_S16_LE,
|
|
},
|
|
},
|
|
{
|
|
.name = "bt-sco-pcm-wb",
|
|
.playback = {
|
|
.stream_name = "Playback",
|
|
.channels_min = 1,
|
|
.channels_max = 1,
|
|
.rates = SNDRV_PCM_RATE_8000 | SNDRV_PCM_RATE_16000,
|
|
.formats = SNDRV_PCM_FMTBIT_S16_LE,
|
|
},
|
|
.capture = {
|
|
.stream_name = "Capture",
|
|
.channels_min = 1,
|
|
.channels_max = 1,
|
|
.rates = SNDRV_PCM_RATE_8000 | SNDRV_PCM_RATE_16000,
|
|
.formats = SNDRV_PCM_FMTBIT_S16_LE,
|
|
},
|
|
}
|
|
};
|
|
|
|
static const struct snd_soc_component_driver soc_component_dev_bt_sco = {
|
|
.dapm_widgets = bt_sco_widgets,
|
|
.num_dapm_widgets = ARRAY_SIZE(bt_sco_widgets),
|
|
.dapm_routes = bt_sco_routes,
|
|
.num_dapm_routes = ARRAY_SIZE(bt_sco_routes),
|
|
.idle_bias_on = 1,
|
|
.use_pmdown_time = 1,
|
|
.endianness = 1,
|
|
.non_legacy_dai_naming = 1,
|
|
};
|
|
|
|
static int bt_sco_probe(struct platform_device *pdev)
|
|
{
|
|
return devm_snd_soc_register_component(&pdev->dev,
|
|
&soc_component_dev_bt_sco,
|
|
bt_sco_dai, ARRAY_SIZE(bt_sco_dai));
|
|
}
|
|
|
|
static int bt_sco_remove(struct platform_device *pdev)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static const struct platform_device_id bt_sco_driver_ids[] = {
|
|
{
|
|
.name = "dfbmcs320",
|
|
},
|
|
{
|
|
.name = "bt-sco",
|
|
},
|
|
{},
|
|
};
|
|
MODULE_DEVICE_TABLE(platform, bt_sco_driver_ids);
|
|
|
|
#if defined(CONFIG_OF)
|
|
static const struct of_device_id bt_sco_codec_of_match[] = {
|
|
{ .compatible = "delta,dfbmcs320", },
|
|
{ .compatible = "linux,bt-sco", },
|
|
{},
|
|
};
|
|
MODULE_DEVICE_TABLE(of, bt_sco_codec_of_match);
|
|
#endif
|
|
|
|
static struct platform_driver bt_sco_driver = {
|
|
.driver = {
|
|
.name = "bt-sco",
|
|
.of_match_table = of_match_ptr(bt_sco_codec_of_match),
|
|
},
|
|
.probe = bt_sco_probe,
|
|
.remove = bt_sco_remove,
|
|
.id_table = bt_sco_driver_ids,
|
|
};
|
|
|
|
module_platform_driver(bt_sco_driver);
|
|
|
|
MODULE_AUTHOR("Lars-Peter Clausen <lars@metafoo.de>");
|
|
MODULE_DESCRIPTION("ASoC generic bluetooth sco link driver");
|
|
MODULE_LICENSE("GPL");
|