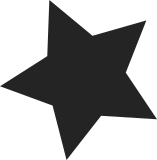
This reverts merge0f75ef6a9c
(and thus effectively commits7a1ade8475
("keys: Provide KEYCTL_GRANT_PERMISSION")2e12256b9a
("keys: Replace uid/gid/perm permissions checking with an ACL") that the merge brought in). It turns out that it breaks booting with an encrypted volume, and Eric biggers reports that it also breaks the fscrypt tests [1] and loading of in-kernel X.509 certificates [2]. The root cause of all the breakage is likely the same, but David Howells is off email so rather than try to work it out it's getting reverted in order to not impact the rest of the merge window. [1] https://lore.kernel.org/lkml/20190710011559.GA7973@sol.localdomain/ [2] https://lore.kernel.org/lkml/20190710013225.GB7973@sol.localdomain/ Link: https://lore.kernel.org/lkml/CAHk-=wjxoeMJfeBahnWH=9zShKp2bsVy527vo3_y8HfOdhwAAw@mail.gmail.com/ Reported-by: Eric Biggers <ebiggers@kernel.org> Cc: David Howells <dhowells@redhat.com> Cc: James Morris <jmorris@namei.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
107 lines
2.7 KiB
C
107 lines
2.7 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/* Key permission checking
|
|
*
|
|
* Copyright (C) 2005 Red Hat, Inc. All Rights Reserved.
|
|
* Written by David Howells (dhowells@redhat.com)
|
|
*/
|
|
|
|
#include <linux/export.h>
|
|
#include <linux/security.h>
|
|
#include "internal.h"
|
|
|
|
/**
|
|
* key_task_permission - Check a key can be used
|
|
* @key_ref: The key to check.
|
|
* @cred: The credentials to use.
|
|
* @perm: The permissions to check for.
|
|
*
|
|
* Check to see whether permission is granted to use a key in the desired way,
|
|
* but permit the security modules to override.
|
|
*
|
|
* The caller must hold either a ref on cred or must hold the RCU readlock.
|
|
*
|
|
* Returns 0 if successful, -EACCES if access is denied based on the
|
|
* permissions bits or the LSM check.
|
|
*/
|
|
int key_task_permission(const key_ref_t key_ref, const struct cred *cred,
|
|
unsigned perm)
|
|
{
|
|
struct key *key;
|
|
key_perm_t kperm;
|
|
int ret;
|
|
|
|
key = key_ref_to_ptr(key_ref);
|
|
|
|
/* use the second 8-bits of permissions for keys the caller owns */
|
|
if (uid_eq(key->uid, cred->fsuid)) {
|
|
kperm = key->perm >> 16;
|
|
goto use_these_perms;
|
|
}
|
|
|
|
/* use the third 8-bits of permissions for keys the caller has a group
|
|
* membership in common with */
|
|
if (gid_valid(key->gid) && key->perm & KEY_GRP_ALL) {
|
|
if (gid_eq(key->gid, cred->fsgid)) {
|
|
kperm = key->perm >> 8;
|
|
goto use_these_perms;
|
|
}
|
|
|
|
ret = groups_search(cred->group_info, key->gid);
|
|
if (ret) {
|
|
kperm = key->perm >> 8;
|
|
goto use_these_perms;
|
|
}
|
|
}
|
|
|
|
/* otherwise use the least-significant 8-bits */
|
|
kperm = key->perm;
|
|
|
|
use_these_perms:
|
|
|
|
/* use the top 8-bits of permissions for keys the caller possesses
|
|
* - possessor permissions are additive with other permissions
|
|
*/
|
|
if (is_key_possessed(key_ref))
|
|
kperm |= key->perm >> 24;
|
|
|
|
kperm = kperm & perm & KEY_NEED_ALL;
|
|
|
|
if (kperm != perm)
|
|
return -EACCES;
|
|
|
|
/* let LSM be the final arbiter */
|
|
return security_key_permission(key_ref, cred, perm);
|
|
}
|
|
EXPORT_SYMBOL(key_task_permission);
|
|
|
|
/**
|
|
* key_validate - Validate a key.
|
|
* @key: The key to be validated.
|
|
*
|
|
* Check that a key is valid, returning 0 if the key is okay, -ENOKEY if the
|
|
* key is invalidated, -EKEYREVOKED if the key's type has been removed or if
|
|
* the key has been revoked or -EKEYEXPIRED if the key has expired.
|
|
*/
|
|
int key_validate(const struct key *key)
|
|
{
|
|
unsigned long flags = READ_ONCE(key->flags);
|
|
time64_t expiry = READ_ONCE(key->expiry);
|
|
|
|
if (flags & (1 << KEY_FLAG_INVALIDATED))
|
|
return -ENOKEY;
|
|
|
|
/* check it's still accessible */
|
|
if (flags & ((1 << KEY_FLAG_REVOKED) |
|
|
(1 << KEY_FLAG_DEAD)))
|
|
return -EKEYREVOKED;
|
|
|
|
/* check it hasn't expired */
|
|
if (expiry) {
|
|
if (ktime_get_real_seconds() >= expiry)
|
|
return -EKEYEXPIRED;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
EXPORT_SYMBOL(key_validate);
|