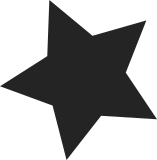
This patch (as995) cleans up the remains of the former NO_AUTOSUSPEND quirk. Since autosuspend is disabled by default, we will let userspace worry about which devices can safely be suspended. Thus the lengthy series of quirk entries is no longer needed, and neither is the quirk ID. I suppose someone might eventually run across a hub that can't be suspended; let's ignore the possibility for now. The patch also cleans up the hasty way in which autosuspend gets disabled. Setting udev->autosuspend_delay to -1 wasn't quite right, because the value is always supposed to be a multiple of HZ. It's better to leave the delay value alone and set autosuspend_disabled, which is what the quirk routine used to do. Signed-off-by: Alan Stern <stern@rowland.harvard.edu> Cc: stable <stable@kernel.org> Signed-off-by: Greg Kroah-Hartman <gregkh@suse.de>
83 lines
2.3 KiB
C
83 lines
2.3 KiB
C
/*
|
|
* USB device quirk handling logic and table
|
|
*
|
|
* Copyright (c) 2007 Oliver Neukum
|
|
* Copyright (c) 2007 Greg Kroah-Hartman <gregkh@suse.de>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License as published by the Free
|
|
* Software Foundation, version 2.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#include <linux/usb.h>
|
|
#include <linux/usb/quirks.h>
|
|
#include "usb.h"
|
|
|
|
/* List of quirky USB devices. Please keep this list ordered by:
|
|
* 1) Vendor ID
|
|
* 2) Product ID
|
|
* 3) Class ID
|
|
*
|
|
* as we want specific devices to be overridden first, and only after that, any
|
|
* class specific quirks.
|
|
*
|
|
* Right now the logic aborts if it finds a valid device in the table, we might
|
|
* want to change that in the future if it turns out that a whole class of
|
|
* devices is broken...
|
|
*/
|
|
static const struct usb_device_id usb_quirk_list[] = {
|
|
/* CBM - Flash disk */
|
|
{ USB_DEVICE(0x0204, 0x6025), .driver_info = USB_QUIRK_RESET_RESUME },
|
|
/* HP 5300/5370C scanner */
|
|
{ USB_DEVICE(0x03f0, 0x0701), .driver_info = USB_QUIRK_STRING_FETCH_255 },
|
|
|
|
/* INTEL VALUE SSD */
|
|
{ USB_DEVICE(0x8086, 0xf1a5), .driver_info = USB_QUIRK_RESET_RESUME },
|
|
|
|
/* M-Systems Flash Disk Pioneers */
|
|
{ USB_DEVICE(0x08ec, 0x1000), .driver_info = USB_QUIRK_RESET_RESUME },
|
|
|
|
/* Philips PSC805 audio device */
|
|
{ USB_DEVICE(0x0471, 0x0155), .driver_info = USB_QUIRK_RESET_RESUME },
|
|
|
|
/* SKYMEDI USB_DRIVE */
|
|
{ USB_DEVICE(0x1516, 0x8628), .driver_info = USB_QUIRK_RESET_RESUME },
|
|
|
|
{ } /* terminating entry must be last */
|
|
};
|
|
|
|
static const struct usb_device_id *find_id(struct usb_device *udev)
|
|
{
|
|
const struct usb_device_id *id = usb_quirk_list;
|
|
|
|
for (; id->idVendor || id->bDeviceClass || id->bInterfaceClass ||
|
|
id->driver_info; id++) {
|
|
if (usb_match_device(udev, id))
|
|
return id;
|
|
}
|
|
return NULL;
|
|
}
|
|
|
|
/*
|
|
* Detect any quirks the device has, and do any housekeeping for it if needed.
|
|
*/
|
|
void usb_detect_quirks(struct usb_device *udev)
|
|
{
|
|
const struct usb_device_id *id = usb_quirk_list;
|
|
|
|
id = find_id(udev);
|
|
if (id)
|
|
udev->quirks = (u32)(id->driver_info);
|
|
if (udev->quirks)
|
|
dev_dbg(&udev->dev, "USB quirks for this device: %x\n",
|
|
udev->quirks);
|
|
|
|
/* By default, disable autosuspend for all non-hubs */
|
|
#ifdef CONFIG_USB_SUSPEND
|
|
if (udev->descriptor.bDeviceClass != USB_CLASS_HUB)
|
|
udev->autosuspend_disabled = 1;
|
|
#endif
|
|
}
|