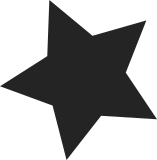
The code cleanups fix up W=1 compiler warnings and some unnecessary checks. The new Kconfig option, defaulting to N, allows the rarely used eCryptfs kernel to userspace communication channel to be compiled out. This may be the first step in it being eventually removed. -----BEGIN PGP SIGNATURE----- Version: GnuPG v1.4.12 (GNU/Linux) iQIcBAABCgAGBQJRN+Z7AAoJENaSAD2qAscKr00P/0/sNgen9e5zqe1q+CAj6hW0 ynzWY/ZNk905hU6tmYb/rHwt7DfaSmrZuzypZP9sGbu+q9RITLl65Hm9HGEJuvJA fK0UHejcAMQmf+AGZiiMs0SB4B4z+eAzUQTWZsX22C1u+3zyI5xLs1NBquKwDyeq 5sNbcmzQYn4w04xag3yYVQEow0NeIjjuCUc8gNUPctDQldN9DdFTdwFTar5lvC0s V4qPWqa61mS9xtegryWAw4DNKjUIrZZFFupWPqRYDVYK8N+RQRBL1RWGVRFCJ17j Ho8yi2onPFGt2y/kW6MwsC41wWFk0Mxsfxf/ZaBMm3lpfYM8UbGQJ6+V9wQWOokU kioUcTI0WvK999mRLxUNkXuVuNDv0OUysgtALy5bevfneWrfXxoSKq+MPbyNfC7+ mo2BCIyHLXn7BYhzPTU+XfksPfMneYUi5LWf4Km5XYXlZ8rwk3IKvJQFyVThEv8+ peVvwSwblUHaoQLnFhEVeu4olHO6AdVQtwr53HPgpMPaZj2/vaWQNA4+bu5HZHTG wqBmdo4DH4jgd9D8xiMZMIJTik8j9aUmpntc4eR7RJEKSice4+X1fUXL4n4N4NfD FkYjWCUZI6nkFUGhGDCokCjzZ3GTEzbe+4pNi3ycTnywcOXFSoq2Kx+tNzE4zXBs FlWGJYrCub9UOLwoYV2C =XwgS -----END PGP SIGNATURE----- Merge tag 'ecryptfs-3.9-rc2-fixes' of git://git.kernel.org/pub/scm/linux/kernel/git/tyhicks/ecryptfs Pull ecryptfs fixes from Tyler Hicks: "Minor code cleanups and new Kconfig option to disable /dev/ecryptfs The code cleanups fix up W=1 compiler warnings and some unnecessary checks. The new Kconfig option, defaulting to N, allows the rarely used eCryptfs kernel to userspace communication channel to be compiled out. This may be the first step in it being eventually removed." Hmm. I'm not sure whether these should be called "fixes", and it probably should have gone in the merge window. But I'll let it slide. * tag 'ecryptfs-3.9-rc2-fixes' of git://git.kernel.org/pub/scm/linux/kernel/git/tyhicks/ecryptfs: eCryptfs: allow userspace messaging to be disabled eCryptfs: Fix redundant error check on ecryptfs_find_daemon_by_euid() ecryptfs: ecryptfs_msg_ctx_alloc_to_free(): remove kfree() redundant null check eCryptfs: decrypt_pki_encrypted_session_key(): remove kfree() redundant null check eCryptfs: remove unneeded checks in virt_to_scatterlist() eCryptfs: Fix -Wmissing-prototypes warnings eCryptfs: Fix -Wunused-but-set-variable warnings eCryptfs: initialize payload_len in keystore.c
374 lines
11 KiB
C
374 lines
11 KiB
C
/**
|
|
* eCryptfs: Linux filesystem encryption layer
|
|
*
|
|
* Copyright (C) 1997-2004 Erez Zadok
|
|
* Copyright (C) 2001-2004 Stony Brook University
|
|
* Copyright (C) 2004-2007 International Business Machines Corp.
|
|
* Author(s): Michael A. Halcrow <mhalcrow@us.ibm.com>
|
|
* Michael C. Thompson <mcthomps@us.ibm.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of the
|
|
* License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but
|
|
* WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA
|
|
* 02111-1307, USA.
|
|
*/
|
|
|
|
#include <linux/file.h>
|
|
#include <linux/poll.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/mount.h>
|
|
#include <linux/pagemap.h>
|
|
#include <linux/security.h>
|
|
#include <linux/compat.h>
|
|
#include <linux/fs_stack.h>
|
|
#include "ecryptfs_kernel.h"
|
|
|
|
/**
|
|
* ecryptfs_read_update_atime
|
|
*
|
|
* generic_file_read updates the atime of upper layer inode. But, it
|
|
* doesn't give us a chance to update the atime of the lower layer
|
|
* inode. This function is a wrapper to generic_file_read. It
|
|
* updates the atime of the lower level inode if generic_file_read
|
|
* returns without any errors. This is to be used only for file reads.
|
|
* The function to be used for directory reads is ecryptfs_read.
|
|
*/
|
|
static ssize_t ecryptfs_read_update_atime(struct kiocb *iocb,
|
|
const struct iovec *iov,
|
|
unsigned long nr_segs, loff_t pos)
|
|
{
|
|
ssize_t rc;
|
|
struct path lower;
|
|
struct file *file = iocb->ki_filp;
|
|
|
|
rc = generic_file_aio_read(iocb, iov, nr_segs, pos);
|
|
/*
|
|
* Even though this is a async interface, we need to wait
|
|
* for IO to finish to update atime
|
|
*/
|
|
if (-EIOCBQUEUED == rc)
|
|
rc = wait_on_sync_kiocb(iocb);
|
|
if (rc >= 0) {
|
|
lower.dentry = ecryptfs_dentry_to_lower(file->f_path.dentry);
|
|
lower.mnt = ecryptfs_dentry_to_lower_mnt(file->f_path.dentry);
|
|
touch_atime(&lower);
|
|
}
|
|
return rc;
|
|
}
|
|
|
|
struct ecryptfs_getdents_callback {
|
|
void *dirent;
|
|
struct dentry *dentry;
|
|
filldir_t filldir;
|
|
int filldir_called;
|
|
int entries_written;
|
|
};
|
|
|
|
/* Inspired by generic filldir in fs/readdir.c */
|
|
static int
|
|
ecryptfs_filldir(void *dirent, const char *lower_name, int lower_namelen,
|
|
loff_t offset, u64 ino, unsigned int d_type)
|
|
{
|
|
struct ecryptfs_getdents_callback *buf =
|
|
(struct ecryptfs_getdents_callback *)dirent;
|
|
size_t name_size;
|
|
char *name;
|
|
int rc;
|
|
|
|
buf->filldir_called++;
|
|
rc = ecryptfs_decode_and_decrypt_filename(&name, &name_size,
|
|
buf->dentry, lower_name,
|
|
lower_namelen);
|
|
if (rc) {
|
|
printk(KERN_ERR "%s: Error attempting to decode and decrypt "
|
|
"filename [%s]; rc = [%d]\n", __func__, lower_name,
|
|
rc);
|
|
goto out;
|
|
}
|
|
rc = buf->filldir(buf->dirent, name, name_size, offset, ino, d_type);
|
|
kfree(name);
|
|
if (rc >= 0)
|
|
buf->entries_written++;
|
|
out:
|
|
return rc;
|
|
}
|
|
|
|
/**
|
|
* ecryptfs_readdir
|
|
* @file: The eCryptfs directory file
|
|
* @dirent: Directory entry handle
|
|
* @filldir: The filldir callback function
|
|
*/
|
|
static int ecryptfs_readdir(struct file *file, void *dirent, filldir_t filldir)
|
|
{
|
|
int rc;
|
|
struct file *lower_file;
|
|
struct inode *inode;
|
|
struct ecryptfs_getdents_callback buf;
|
|
|
|
lower_file = ecryptfs_file_to_lower(file);
|
|
lower_file->f_pos = file->f_pos;
|
|
inode = file_inode(file);
|
|
memset(&buf, 0, sizeof(buf));
|
|
buf.dirent = dirent;
|
|
buf.dentry = file->f_path.dentry;
|
|
buf.filldir = filldir;
|
|
buf.filldir_called = 0;
|
|
buf.entries_written = 0;
|
|
rc = vfs_readdir(lower_file, ecryptfs_filldir, (void *)&buf);
|
|
file->f_pos = lower_file->f_pos;
|
|
if (rc < 0)
|
|
goto out;
|
|
if (buf.filldir_called && !buf.entries_written)
|
|
goto out;
|
|
if (rc >= 0)
|
|
fsstack_copy_attr_atime(inode,
|
|
file_inode(lower_file));
|
|
out:
|
|
return rc;
|
|
}
|
|
|
|
struct kmem_cache *ecryptfs_file_info_cache;
|
|
|
|
static int read_or_initialize_metadata(struct dentry *dentry)
|
|
{
|
|
struct inode *inode = dentry->d_inode;
|
|
struct ecryptfs_mount_crypt_stat *mount_crypt_stat;
|
|
struct ecryptfs_crypt_stat *crypt_stat;
|
|
int rc;
|
|
|
|
crypt_stat = &ecryptfs_inode_to_private(inode)->crypt_stat;
|
|
mount_crypt_stat = &ecryptfs_superblock_to_private(
|
|
inode->i_sb)->mount_crypt_stat;
|
|
mutex_lock(&crypt_stat->cs_mutex);
|
|
|
|
if (crypt_stat->flags & ECRYPTFS_POLICY_APPLIED &&
|
|
crypt_stat->flags & ECRYPTFS_KEY_VALID) {
|
|
rc = 0;
|
|
goto out;
|
|
}
|
|
|
|
rc = ecryptfs_read_metadata(dentry);
|
|
if (!rc)
|
|
goto out;
|
|
|
|
if (mount_crypt_stat->flags & ECRYPTFS_PLAINTEXT_PASSTHROUGH_ENABLED) {
|
|
crypt_stat->flags &= ~(ECRYPTFS_I_SIZE_INITIALIZED
|
|
| ECRYPTFS_ENCRYPTED);
|
|
rc = 0;
|
|
goto out;
|
|
}
|
|
|
|
if (!(mount_crypt_stat->flags & ECRYPTFS_XATTR_METADATA_ENABLED) &&
|
|
!i_size_read(ecryptfs_inode_to_lower(inode))) {
|
|
rc = ecryptfs_initialize_file(dentry, inode);
|
|
if (!rc)
|
|
goto out;
|
|
}
|
|
|
|
rc = -EIO;
|
|
out:
|
|
mutex_unlock(&crypt_stat->cs_mutex);
|
|
return rc;
|
|
}
|
|
|
|
/**
|
|
* ecryptfs_open
|
|
* @inode: inode speciying file to open
|
|
* @file: Structure to return filled in
|
|
*
|
|
* Opens the file specified by inode.
|
|
*
|
|
* Returns zero on success; non-zero otherwise
|
|
*/
|
|
static int ecryptfs_open(struct inode *inode, struct file *file)
|
|
{
|
|
int rc = 0;
|
|
struct ecryptfs_crypt_stat *crypt_stat = NULL;
|
|
struct ecryptfs_mount_crypt_stat *mount_crypt_stat;
|
|
struct dentry *ecryptfs_dentry = file->f_path.dentry;
|
|
/* Private value of ecryptfs_dentry allocated in
|
|
* ecryptfs_lookup() */
|
|
struct ecryptfs_file_info *file_info;
|
|
|
|
mount_crypt_stat = &ecryptfs_superblock_to_private(
|
|
ecryptfs_dentry->d_sb)->mount_crypt_stat;
|
|
if ((mount_crypt_stat->flags & ECRYPTFS_ENCRYPTED_VIEW_ENABLED)
|
|
&& ((file->f_flags & O_WRONLY) || (file->f_flags & O_RDWR)
|
|
|| (file->f_flags & O_CREAT) || (file->f_flags & O_TRUNC)
|
|
|| (file->f_flags & O_APPEND))) {
|
|
printk(KERN_WARNING "Mount has encrypted view enabled; "
|
|
"files may only be read\n");
|
|
rc = -EPERM;
|
|
goto out;
|
|
}
|
|
/* Released in ecryptfs_release or end of function if failure */
|
|
file_info = kmem_cache_zalloc(ecryptfs_file_info_cache, GFP_KERNEL);
|
|
ecryptfs_set_file_private(file, file_info);
|
|
if (!file_info) {
|
|
ecryptfs_printk(KERN_ERR,
|
|
"Error attempting to allocate memory\n");
|
|
rc = -ENOMEM;
|
|
goto out;
|
|
}
|
|
crypt_stat = &ecryptfs_inode_to_private(inode)->crypt_stat;
|
|
mutex_lock(&crypt_stat->cs_mutex);
|
|
if (!(crypt_stat->flags & ECRYPTFS_POLICY_APPLIED)) {
|
|
ecryptfs_printk(KERN_DEBUG, "Setting flags for stat...\n");
|
|
/* Policy code enabled in future release */
|
|
crypt_stat->flags |= (ECRYPTFS_POLICY_APPLIED
|
|
| ECRYPTFS_ENCRYPTED);
|
|
}
|
|
mutex_unlock(&crypt_stat->cs_mutex);
|
|
rc = ecryptfs_get_lower_file(ecryptfs_dentry, inode);
|
|
if (rc) {
|
|
printk(KERN_ERR "%s: Error attempting to initialize "
|
|
"the lower file for the dentry with name "
|
|
"[%s]; rc = [%d]\n", __func__,
|
|
ecryptfs_dentry->d_name.name, rc);
|
|
goto out_free;
|
|
}
|
|
if ((ecryptfs_inode_to_private(inode)->lower_file->f_flags & O_ACCMODE)
|
|
== O_RDONLY && (file->f_flags & O_ACCMODE) != O_RDONLY) {
|
|
rc = -EPERM;
|
|
printk(KERN_WARNING "%s: Lower file is RO; eCryptfs "
|
|
"file must hence be opened RO\n", __func__);
|
|
goto out_put;
|
|
}
|
|
ecryptfs_set_file_lower(
|
|
file, ecryptfs_inode_to_private(inode)->lower_file);
|
|
if (S_ISDIR(ecryptfs_dentry->d_inode->i_mode)) {
|
|
ecryptfs_printk(KERN_DEBUG, "This is a directory\n");
|
|
mutex_lock(&crypt_stat->cs_mutex);
|
|
crypt_stat->flags &= ~(ECRYPTFS_ENCRYPTED);
|
|
mutex_unlock(&crypt_stat->cs_mutex);
|
|
rc = 0;
|
|
goto out;
|
|
}
|
|
rc = read_or_initialize_metadata(ecryptfs_dentry);
|
|
if (rc)
|
|
goto out_put;
|
|
ecryptfs_printk(KERN_DEBUG, "inode w/ addr = [0x%p], i_ino = "
|
|
"[0x%.16lx] size: [0x%.16llx]\n", inode, inode->i_ino,
|
|
(unsigned long long)i_size_read(inode));
|
|
goto out;
|
|
out_put:
|
|
ecryptfs_put_lower_file(inode);
|
|
out_free:
|
|
kmem_cache_free(ecryptfs_file_info_cache,
|
|
ecryptfs_file_to_private(file));
|
|
out:
|
|
return rc;
|
|
}
|
|
|
|
static int ecryptfs_flush(struct file *file, fl_owner_t td)
|
|
{
|
|
struct file *lower_file = ecryptfs_file_to_lower(file);
|
|
|
|
if (lower_file->f_op && lower_file->f_op->flush) {
|
|
filemap_write_and_wait(file->f_mapping);
|
|
return lower_file->f_op->flush(lower_file, td);
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int ecryptfs_release(struct inode *inode, struct file *file)
|
|
{
|
|
ecryptfs_put_lower_file(inode);
|
|
kmem_cache_free(ecryptfs_file_info_cache,
|
|
ecryptfs_file_to_private(file));
|
|
return 0;
|
|
}
|
|
|
|
static int
|
|
ecryptfs_fsync(struct file *file, loff_t start, loff_t end, int datasync)
|
|
{
|
|
return vfs_fsync(ecryptfs_file_to_lower(file), datasync);
|
|
}
|
|
|
|
static int ecryptfs_fasync(int fd, struct file *file, int flag)
|
|
{
|
|
int rc = 0;
|
|
struct file *lower_file = NULL;
|
|
|
|
lower_file = ecryptfs_file_to_lower(file);
|
|
if (lower_file->f_op && lower_file->f_op->fasync)
|
|
rc = lower_file->f_op->fasync(fd, lower_file, flag);
|
|
return rc;
|
|
}
|
|
|
|
static long
|
|
ecryptfs_unlocked_ioctl(struct file *file, unsigned int cmd, unsigned long arg)
|
|
{
|
|
struct file *lower_file = NULL;
|
|
long rc = -ENOTTY;
|
|
|
|
if (ecryptfs_file_to_private(file))
|
|
lower_file = ecryptfs_file_to_lower(file);
|
|
if (lower_file && lower_file->f_op && lower_file->f_op->unlocked_ioctl)
|
|
rc = lower_file->f_op->unlocked_ioctl(lower_file, cmd, arg);
|
|
return rc;
|
|
}
|
|
|
|
#ifdef CONFIG_COMPAT
|
|
static long
|
|
ecryptfs_compat_ioctl(struct file *file, unsigned int cmd, unsigned long arg)
|
|
{
|
|
struct file *lower_file = NULL;
|
|
long rc = -ENOIOCTLCMD;
|
|
|
|
if (ecryptfs_file_to_private(file))
|
|
lower_file = ecryptfs_file_to_lower(file);
|
|
if (lower_file && lower_file->f_op && lower_file->f_op->compat_ioctl)
|
|
rc = lower_file->f_op->compat_ioctl(lower_file, cmd, arg);
|
|
return rc;
|
|
}
|
|
#endif
|
|
|
|
const struct file_operations ecryptfs_dir_fops = {
|
|
.readdir = ecryptfs_readdir,
|
|
.read = generic_read_dir,
|
|
.unlocked_ioctl = ecryptfs_unlocked_ioctl,
|
|
#ifdef CONFIG_COMPAT
|
|
.compat_ioctl = ecryptfs_compat_ioctl,
|
|
#endif
|
|
.open = ecryptfs_open,
|
|
.flush = ecryptfs_flush,
|
|
.release = ecryptfs_release,
|
|
.fsync = ecryptfs_fsync,
|
|
.fasync = ecryptfs_fasync,
|
|
.splice_read = generic_file_splice_read,
|
|
.llseek = default_llseek,
|
|
};
|
|
|
|
const struct file_operations ecryptfs_main_fops = {
|
|
.llseek = generic_file_llseek,
|
|
.read = do_sync_read,
|
|
.aio_read = ecryptfs_read_update_atime,
|
|
.write = do_sync_write,
|
|
.aio_write = generic_file_aio_write,
|
|
.readdir = ecryptfs_readdir,
|
|
.unlocked_ioctl = ecryptfs_unlocked_ioctl,
|
|
#ifdef CONFIG_COMPAT
|
|
.compat_ioctl = ecryptfs_compat_ioctl,
|
|
#endif
|
|
.mmap = generic_file_mmap,
|
|
.open = ecryptfs_open,
|
|
.flush = ecryptfs_flush,
|
|
.release = ecryptfs_release,
|
|
.fsync = ecryptfs_fsync,
|
|
.fasync = ecryptfs_fasync,
|
|
.splice_read = generic_file_splice_read,
|
|
};
|