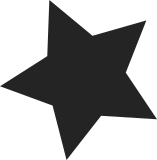
Patch series "mm: remove quicklist page table caches". A while ago Nicholas proposed to remove quicklist page table caches [1]. I've rebased his patch on the curren upstream and switched ia64 and sh to use generic versions of PTE allocation. [1] https://lore.kernel.org/linux-mm/20190711030339.20892-1-npiggin@gmail.com This patch (of 3): Remove page table allocator "quicklists". These have been around for a long time, but have not got much traction in the last decade and are only used on ia64 and sh architectures. The numbers in the initial commit look interesting but probably don't apply anymore. If anybody wants to resurrect this it's in the git history, but it's unhelpful to have this code and divergent allocator behaviour for minor archs. Also it might be better to instead make more general improvements to page allocator if this is still so slow. Link: http://lkml.kernel.org/r/1565250728-21721-2-git-send-email-rppt@linux.ibm.com Signed-off-by: Nicholas Piggin <npiggin@gmail.com> Signed-off-by: Mike Rapoport <rppt@linux.ibm.com> Cc: Tony Luck <tony.luck@intel.com> Cc: Yoshinori Sato <ysato@users.sourceforge.jp> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
128 lines
3.6 KiB
C
128 lines
3.6 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _ASM_PGALLOC_H
|
|
#define _ASM_PGALLOC_H
|
|
|
|
#include <linux/gfp.h>
|
|
#include <linux/mm.h>
|
|
#include <linux/threads.h>
|
|
#include <asm/processor.h>
|
|
#include <asm/fixmap.h>
|
|
|
|
#include <asm/cache.h>
|
|
|
|
#include <asm-generic/pgalloc.h> /* for pte_{alloc,free}_one */
|
|
|
|
/* Allocate the top level pgd (page directory)
|
|
*
|
|
* Here (for 64 bit kernels) we implement a Hybrid L2/L3 scheme: we
|
|
* allocate the first pmd adjacent to the pgd. This means that we can
|
|
* subtract a constant offset to get to it. The pmd and pgd sizes are
|
|
* arranged so that a single pmd covers 4GB (giving a full 64-bit
|
|
* process access to 8TB) so our lookups are effectively L2 for the
|
|
* first 4GB of the kernel (i.e. for all ILP32 processes and all the
|
|
* kernel for machines with under 4GB of memory) */
|
|
static inline pgd_t *pgd_alloc(struct mm_struct *mm)
|
|
{
|
|
pgd_t *pgd = (pgd_t *)__get_free_pages(GFP_KERNEL,
|
|
PGD_ALLOC_ORDER);
|
|
pgd_t *actual_pgd = pgd;
|
|
|
|
if (likely(pgd != NULL)) {
|
|
memset(pgd, 0, PAGE_SIZE<<PGD_ALLOC_ORDER);
|
|
#if CONFIG_PGTABLE_LEVELS == 3
|
|
actual_pgd += PTRS_PER_PGD;
|
|
/* Populate first pmd with allocated memory. We mark it
|
|
* with PxD_FLAG_ATTACHED as a signal to the system that this
|
|
* pmd entry may not be cleared. */
|
|
__pgd_val_set(*actual_pgd, (PxD_FLAG_PRESENT |
|
|
PxD_FLAG_VALID |
|
|
PxD_FLAG_ATTACHED)
|
|
+ (__u32)(__pa((unsigned long)pgd) >> PxD_VALUE_SHIFT));
|
|
/* The first pmd entry also is marked with PxD_FLAG_ATTACHED as
|
|
* a signal that this pmd may not be freed */
|
|
__pgd_val_set(*pgd, PxD_FLAG_ATTACHED);
|
|
#endif
|
|
}
|
|
spin_lock_init(pgd_spinlock(actual_pgd));
|
|
return actual_pgd;
|
|
}
|
|
|
|
static inline void pgd_free(struct mm_struct *mm, pgd_t *pgd)
|
|
{
|
|
#if CONFIG_PGTABLE_LEVELS == 3
|
|
pgd -= PTRS_PER_PGD;
|
|
#endif
|
|
free_pages((unsigned long)pgd, PGD_ALLOC_ORDER);
|
|
}
|
|
|
|
#if CONFIG_PGTABLE_LEVELS == 3
|
|
|
|
/* Three Level Page Table Support for pmd's */
|
|
|
|
static inline void pgd_populate(struct mm_struct *mm, pgd_t *pgd, pmd_t *pmd)
|
|
{
|
|
__pgd_val_set(*pgd, (PxD_FLAG_PRESENT | PxD_FLAG_VALID) +
|
|
(__u32)(__pa((unsigned long)pmd) >> PxD_VALUE_SHIFT));
|
|
}
|
|
|
|
static inline pmd_t *pmd_alloc_one(struct mm_struct *mm, unsigned long address)
|
|
{
|
|
pmd_t *pmd = (pmd_t *)__get_free_pages(GFP_KERNEL, PMD_ORDER);
|
|
if (pmd)
|
|
memset(pmd, 0, PAGE_SIZE<<PMD_ORDER);
|
|
return pmd;
|
|
}
|
|
|
|
static inline void pmd_free(struct mm_struct *mm, pmd_t *pmd)
|
|
{
|
|
if (pmd_flag(*pmd) & PxD_FLAG_ATTACHED) {
|
|
/*
|
|
* This is the permanent pmd attached to the pgd;
|
|
* cannot free it.
|
|
* Increment the counter to compensate for the decrement
|
|
* done by generic mm code.
|
|
*/
|
|
mm_inc_nr_pmds(mm);
|
|
return;
|
|
}
|
|
free_pages((unsigned long)pmd, PMD_ORDER);
|
|
}
|
|
|
|
#else
|
|
|
|
/* Two Level Page Table Support for pmd's */
|
|
|
|
/*
|
|
* allocating and freeing a pmd is trivial: the 1-entry pmd is
|
|
* inside the pgd, so has no extra memory associated with it.
|
|
*/
|
|
|
|
#define pmd_alloc_one(mm, addr) ({ BUG(); ((pmd_t *)2); })
|
|
#define pmd_free(mm, x) do { } while (0)
|
|
#define pgd_populate(mm, pmd, pte) BUG()
|
|
|
|
#endif
|
|
|
|
static inline void
|
|
pmd_populate_kernel(struct mm_struct *mm, pmd_t *pmd, pte_t *pte)
|
|
{
|
|
#if CONFIG_PGTABLE_LEVELS == 3
|
|
/* preserve the gateway marker if this is the beginning of
|
|
* the permanent pmd */
|
|
if(pmd_flag(*pmd) & PxD_FLAG_ATTACHED)
|
|
__pmd_val_set(*pmd, (PxD_FLAG_PRESENT |
|
|
PxD_FLAG_VALID |
|
|
PxD_FLAG_ATTACHED)
|
|
+ (__u32)(__pa((unsigned long)pte) >> PxD_VALUE_SHIFT));
|
|
else
|
|
#endif
|
|
__pmd_val_set(*pmd, (PxD_FLAG_PRESENT | PxD_FLAG_VALID)
|
|
+ (__u32)(__pa((unsigned long)pte) >> PxD_VALUE_SHIFT));
|
|
}
|
|
|
|
#define pmd_populate(mm, pmd, pte_page) \
|
|
pmd_populate_kernel(mm, pmd, page_address(pte_page))
|
|
#define pmd_pgtable(pmd) pmd_page(pmd)
|
|
|
|
#endif
|