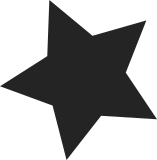
Christopher reported a regression where he was unable to unmount a NFS filesystem where the root had gone stale. The problem is that d_revalidate handles the root of the filesystem differently from other dentries, but d_weak_revalidate does not. We could simply fix this by making d_weak_revalidate return success on IS_ROOT dentries, but there are cases where we do want to revalidate the root of the fs. A umount is really a special case. We generally aren't interested in anything but the dentry and vfsmount that's attached at that point. If the inode turns out to be stale we just don't care since the intent is to stop using it anyway. Try to handle this situation better by treating umount as a special case in the lookup code. Have it resolve the parent using normal means, and then do a lookup of the final dentry without revalidating it. In most cases, the final lookup will come out of the dcache, but the case where there's a trailing symlink or !LAST_NORM entry on the end complicates things a bit. Cc: Neil Brown <neilb@suse.de> Reported-by: Christopher T Vogan <cvogan@us.ibm.com> Signed-off-by: Jeff Layton <jlayton@redhat.com> Signed-off-by: Al Viro <viro@zeniv.linux.org.uk>
120 lines
3.5 KiB
C
120 lines
3.5 KiB
C
#ifndef _LINUX_NAMEI_H
|
|
#define _LINUX_NAMEI_H
|
|
|
|
#include <linux/dcache.h>
|
|
#include <linux/errno.h>
|
|
#include <linux/linkage.h>
|
|
#include <linux/path.h>
|
|
|
|
struct vfsmount;
|
|
|
|
enum { MAX_NESTED_LINKS = 8 };
|
|
|
|
struct nameidata {
|
|
struct path path;
|
|
struct qstr last;
|
|
struct path root;
|
|
struct inode *inode; /* path.dentry.d_inode */
|
|
unsigned int flags;
|
|
unsigned seq;
|
|
int last_type;
|
|
unsigned depth;
|
|
char *saved_names[MAX_NESTED_LINKS + 1];
|
|
};
|
|
|
|
/*
|
|
* Type of the last component on LOOKUP_PARENT
|
|
*/
|
|
enum {LAST_NORM, LAST_ROOT, LAST_DOT, LAST_DOTDOT, LAST_BIND};
|
|
|
|
/*
|
|
* The bitmask for a lookup event:
|
|
* - follow links at the end
|
|
* - require a directory
|
|
* - ending slashes ok even for nonexistent files
|
|
* - internal "there are more path components" flag
|
|
* - dentry cache is untrusted; force a real lookup
|
|
* - suppress terminal automount
|
|
*/
|
|
#define LOOKUP_FOLLOW 0x0001
|
|
#define LOOKUP_DIRECTORY 0x0002
|
|
#define LOOKUP_AUTOMOUNT 0x0004
|
|
|
|
#define LOOKUP_PARENT 0x0010
|
|
#define LOOKUP_REVAL 0x0020
|
|
#define LOOKUP_RCU 0x0040
|
|
|
|
/*
|
|
* Intent data
|
|
*/
|
|
#define LOOKUP_OPEN 0x0100
|
|
#define LOOKUP_CREATE 0x0200
|
|
#define LOOKUP_EXCL 0x0400
|
|
#define LOOKUP_RENAME_TARGET 0x0800
|
|
|
|
#define LOOKUP_JUMPED 0x1000
|
|
#define LOOKUP_ROOT 0x2000
|
|
#define LOOKUP_EMPTY 0x4000
|
|
|
|
extern int user_path_at(int, const char __user *, unsigned, struct path *);
|
|
extern int user_path_at_empty(int, const char __user *, unsigned, struct path *, int *empty);
|
|
extern int user_path_umountat(int, const char __user *, unsigned int, struct path *);
|
|
|
|
#define user_path(name, path) user_path_at(AT_FDCWD, name, LOOKUP_FOLLOW, path)
|
|
#define user_lpath(name, path) user_path_at(AT_FDCWD, name, 0, path)
|
|
#define user_path_dir(name, path) \
|
|
user_path_at(AT_FDCWD, name, LOOKUP_FOLLOW | LOOKUP_DIRECTORY, path)
|
|
|
|
extern int kern_path(const char *, unsigned, struct path *);
|
|
|
|
extern struct dentry *kern_path_create(int, const char *, struct path *, unsigned int);
|
|
extern struct dentry *user_path_create(int, const char __user *, struct path *, unsigned int);
|
|
extern void done_path_create(struct path *, struct dentry *);
|
|
extern struct dentry *kern_path_locked(const char *, struct path *);
|
|
extern int vfs_path_lookup(struct dentry *, struct vfsmount *,
|
|
const char *, unsigned int, struct path *);
|
|
|
|
extern struct dentry *lookup_one_len(const char *, struct dentry *, int);
|
|
|
|
extern int follow_down_one(struct path *);
|
|
extern int follow_down(struct path *);
|
|
extern int follow_up(struct path *);
|
|
|
|
extern struct dentry *lock_rename(struct dentry *, struct dentry *);
|
|
extern void unlock_rename(struct dentry *, struct dentry *);
|
|
|
|
extern void nd_jump_link(struct nameidata *nd, struct path *path);
|
|
|
|
static inline void nd_set_link(struct nameidata *nd, char *path)
|
|
{
|
|
nd->saved_names[nd->depth] = path;
|
|
}
|
|
|
|
static inline char *nd_get_link(struct nameidata *nd)
|
|
{
|
|
return nd->saved_names[nd->depth];
|
|
}
|
|
|
|
static inline void nd_terminate_link(void *name, size_t len, size_t maxlen)
|
|
{
|
|
((char *) name)[min(len, maxlen)] = '\0';
|
|
}
|
|
|
|
/**
|
|
* retry_estale - determine whether the caller should retry an operation
|
|
* @error: the error that would currently be returned
|
|
* @flags: flags being used for next lookup attempt
|
|
*
|
|
* Check to see if the error code was -ESTALE, and then determine whether
|
|
* to retry the call based on whether "flags" already has LOOKUP_REVAL set.
|
|
*
|
|
* Returns true if the caller should try the operation again.
|
|
*/
|
|
static inline bool
|
|
retry_estale(const long error, const unsigned int flags)
|
|
{
|
|
return error == -ESTALE && !(flags & LOOKUP_REVAL);
|
|
}
|
|
|
|
#endif /* _LINUX_NAMEI_H */
|