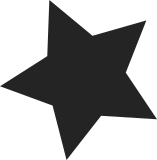
Remove the file name from the comment at top of many files. In most cases the file name was wrong anyway, so it's rather pointless. Also unify the IBM copyright statement. We did have a lot of sightly different statements and wanted to change them one after another whenever a file gets touched. However that never happened. Instead people start to take the old/"wrong" statements to use as a template for new files. So unify all of them in one go. Signed-off-by: Heiko Carstens <heiko.carstens@de.ibm.com>
73 lines
1.5 KiB
C
73 lines
1.5 KiB
C
/*
|
|
* Copyright IBM Corp. 2006, 2010
|
|
* Author(s): Martin Schwidefsky <schwidefsky@de.ibm.com>
|
|
*/
|
|
|
|
#ifndef __ASM_IRQFLAGS_H
|
|
#define __ASM_IRQFLAGS_H
|
|
|
|
#include <linux/types.h>
|
|
|
|
/* store then OR system mask. */
|
|
#define __arch_local_irq_stosm(__or) \
|
|
({ \
|
|
unsigned long __mask; \
|
|
asm volatile( \
|
|
" stosm %0,%1" \
|
|
: "=Q" (__mask) : "i" (__or) : "memory"); \
|
|
__mask; \
|
|
})
|
|
|
|
/* store then AND system mask. */
|
|
#define __arch_local_irq_stnsm(__and) \
|
|
({ \
|
|
unsigned long __mask; \
|
|
asm volatile( \
|
|
" stnsm %0,%1" \
|
|
: "=Q" (__mask) : "i" (__and) : "memory"); \
|
|
__mask; \
|
|
})
|
|
|
|
/* set system mask. */
|
|
static inline notrace void __arch_local_irq_ssm(unsigned long flags)
|
|
{
|
|
asm volatile("ssm %0" : : "Q" (flags) : "memory");
|
|
}
|
|
|
|
static inline notrace unsigned long arch_local_save_flags(void)
|
|
{
|
|
return __arch_local_irq_stosm(0x00);
|
|
}
|
|
|
|
static inline notrace unsigned long arch_local_irq_save(void)
|
|
{
|
|
return __arch_local_irq_stnsm(0xfc);
|
|
}
|
|
|
|
static inline notrace void arch_local_irq_disable(void)
|
|
{
|
|
arch_local_irq_save();
|
|
}
|
|
|
|
static inline notrace void arch_local_irq_enable(void)
|
|
{
|
|
__arch_local_irq_stosm(0x03);
|
|
}
|
|
|
|
static inline notrace void arch_local_irq_restore(unsigned long flags)
|
|
{
|
|
__arch_local_irq_ssm(flags);
|
|
}
|
|
|
|
static inline notrace bool arch_irqs_disabled_flags(unsigned long flags)
|
|
{
|
|
return !(flags & (3UL << (BITS_PER_LONG - 8)));
|
|
}
|
|
|
|
static inline notrace bool arch_irqs_disabled(void)
|
|
{
|
|
return arch_irqs_disabled_flags(arch_local_save_flags());
|
|
}
|
|
|
|
#endif /* __ASM_IRQFLAGS_H */
|