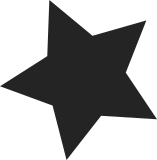
Since commit 7b11304
("dma: mxs-dma: Report correct residue for cyclic DMA")
the mxs dmaengine driver has support for residue reporting. So there is no need
to specify the SND_DMAENGINE_PCM_FLAG_NO_RESIDUE flag anymore. This allows a
finer grained resolution for the PCM pointer as well as avoids the race
condition that can occur with the period counting that is used when the
dmaengine driver does not support residue reporting.
Signed-off-by: Lars-Peter Clausen <lars@metafoo.de>
Tested-by: Shawn Guo <shawn.guo@linaro.org>
Signed-off-by: Mark Brown <broonie@linaro.org>
60 lines
1.8 KiB
C
60 lines
1.8 KiB
C
/*
|
|
* Copyright (C) 2011 Freescale Semiconductor, Inc. All Rights Reserved.
|
|
*
|
|
* Based on sound/soc/imx/imx-pcm-dma-mx2.c
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
|
*/
|
|
|
|
#include <linux/device.h>
|
|
#include <linux/init.h>
|
|
#include <linux/module.h>
|
|
|
|
#include <sound/core.h>
|
|
#include <sound/pcm.h>
|
|
#include <sound/soc.h>
|
|
#include <sound/dmaengine_pcm.h>
|
|
|
|
#include "mxs-pcm.h"
|
|
|
|
static const struct snd_pcm_hardware snd_mxs_hardware = {
|
|
.info = SNDRV_PCM_INFO_MMAP |
|
|
SNDRV_PCM_INFO_MMAP_VALID |
|
|
SNDRV_PCM_INFO_PAUSE |
|
|
SNDRV_PCM_INFO_RESUME |
|
|
SNDRV_PCM_INFO_INTERLEAVED |
|
|
SNDRV_PCM_INFO_HALF_DUPLEX,
|
|
.period_bytes_min = 32,
|
|
.period_bytes_max = 8192,
|
|
.periods_min = 1,
|
|
.periods_max = 52,
|
|
.buffer_bytes_max = 64 * 1024,
|
|
.fifo_size = 32,
|
|
};
|
|
|
|
static const struct snd_dmaengine_pcm_config mxs_dmaengine_pcm_config = {
|
|
.pcm_hardware = &snd_mxs_hardware,
|
|
.prealloc_buffer_size = 64 * 1024,
|
|
};
|
|
|
|
int mxs_pcm_platform_register(struct device *dev)
|
|
{
|
|
return devm_snd_dmaengine_pcm_register(dev, &mxs_dmaengine_pcm_config,
|
|
SND_DMAENGINE_PCM_FLAG_HALF_DUPLEX);
|
|
}
|
|
EXPORT_SYMBOL_GPL(mxs_pcm_platform_register);
|
|
|
|
MODULE_LICENSE("GPL");
|