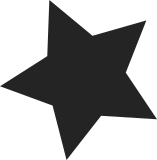
Sometimes it's useful to let the user, while doing performance research, know what in the IEEE754 exceptions has caused many times of FP emulation when running a specific application. This patch adds 5 more files to /sys/kernel/debug/mips/fpuemustats/, whose filenames begin with "ieee754". These stats are in addition to the existing cp1ops, cp1xops, errors, loads and stores, which may not be useful in understanding the reasons of ieee754 exceptions. [ralf@linux-mips.org: Fixed reject due to other changes to the kernel FP assist software.] Signed-off-by: Deng-Cheng Zhu <dengcheng.zhu@imgtec.com> Cc: linux-mips@linux-mips.org Cc: Steven.Hill@imgtec.com Cc: james.hogan@imgtec.com Patchwork: http://patchwork.linux-mips.org/patch/7044/ Signed-off-by: Ralf Baechle <ralf@linux-mips.org>
68 lines
1.5 KiB
C
68 lines
1.5 KiB
C
#include <linux/cpumask.h>
|
|
#include <linux/debugfs.h>
|
|
#include <linux/fs.h>
|
|
#include <linux/init.h>
|
|
#include <linux/percpu.h>
|
|
#include <linux/types.h>
|
|
#include <asm/fpu_emulator.h>
|
|
#include <asm/local.h>
|
|
|
|
DEFINE_PER_CPU(struct mips_fpu_emulator_stats, fpuemustats);
|
|
|
|
static int fpuemu_stat_get(void *data, u64 *val)
|
|
{
|
|
int cpu;
|
|
unsigned long sum = 0;
|
|
|
|
for_each_online_cpu(cpu) {
|
|
struct mips_fpu_emulator_stats *ps;
|
|
local_t *pv;
|
|
|
|
ps = &per_cpu(fpuemustats, cpu);
|
|
pv = (void *)ps + (unsigned long)data;
|
|
sum += local_read(pv);
|
|
}
|
|
*val = sum;
|
|
return 0;
|
|
}
|
|
DEFINE_SIMPLE_ATTRIBUTE(fops_fpuemu_stat, fpuemu_stat_get, NULL, "%llu\n");
|
|
|
|
extern struct dentry *mips_debugfs_dir;
|
|
static int __init debugfs_fpuemu(void)
|
|
{
|
|
struct dentry *d, *dir;
|
|
|
|
if (!mips_debugfs_dir)
|
|
return -ENODEV;
|
|
dir = debugfs_create_dir("fpuemustats", mips_debugfs_dir);
|
|
if (!dir)
|
|
return -ENOMEM;
|
|
|
|
#define FPU_EMU_STAT_OFFSET(m) \
|
|
offsetof(struct mips_fpu_emulator_stats, m)
|
|
|
|
#define FPU_STAT_CREATE(m) \
|
|
do { \
|
|
d = debugfs_create_file(#m , S_IRUGO, dir, \
|
|
(void *)FPU_EMU_STAT_OFFSET(m), \
|
|
&fops_fpuemu_stat); \
|
|
if (!d) \
|
|
return -ENOMEM; \
|
|
} while (0)
|
|
|
|
FPU_STAT_CREATE(emulated);
|
|
FPU_STAT_CREATE(loads);
|
|
FPU_STAT_CREATE(stores);
|
|
FPU_STAT_CREATE(cp1ops);
|
|
FPU_STAT_CREATE(cp1xops);
|
|
FPU_STAT_CREATE(errors);
|
|
FPU_STAT_CREATE(ieee754_inexact);
|
|
FPU_STAT_CREATE(ieee754_underflow);
|
|
FPU_STAT_CREATE(ieee754_overflow);
|
|
FPU_STAT_CREATE(ieee754_zerodiv);
|
|
FPU_STAT_CREATE(ieee754_invalidop);
|
|
|
|
return 0;
|
|
}
|
|
__initcall(debugfs_fpuemu);
|