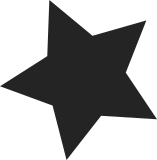
This patch converts IPsec to use the new HMAC template. The names of existing simple digest algorithms may still be used to refer to their HMAC composites. The same structure can be used by other MACs such as AES-XCBC-MAC. This patch also switches from the digest interface to hash. Signed-off-by: Herbert Xu <herbert@gondor.apana.org.au> Signed-off-by: David S. Miller <davem@davemloft.net>
65 lines
1.7 KiB
C
65 lines
1.7 KiB
C
#ifndef _NET_ESP_H
|
|
#define _NET_ESP_H
|
|
|
|
#include <linux/crypto.h>
|
|
#include <net/xfrm.h>
|
|
#include <asm/scatterlist.h>
|
|
|
|
#define ESP_NUM_FAST_SG 4
|
|
|
|
struct esp_data
|
|
{
|
|
struct scatterlist sgbuf[ESP_NUM_FAST_SG];
|
|
|
|
/* Confidentiality */
|
|
struct {
|
|
u8 *key; /* Key */
|
|
int key_len; /* Key length */
|
|
u8 *ivec; /* ivec buffer */
|
|
/* ivlen is offset from enc_data, where encrypted data start.
|
|
* It is logically different of crypto_tfm_alg_ivsize(tfm).
|
|
* We assume that it is either zero (no ivec), or
|
|
* >= crypto_tfm_alg_ivsize(tfm). */
|
|
int ivlen;
|
|
int padlen; /* 0..255 */
|
|
struct crypto_blkcipher *tfm; /* crypto handle */
|
|
} conf;
|
|
|
|
/* Integrity. It is active when icv_full_len != 0 */
|
|
struct {
|
|
u8 *key; /* Key */
|
|
int key_len; /* Length of the key */
|
|
u8 *work_icv;
|
|
int icv_full_len;
|
|
int icv_trunc_len;
|
|
void (*icv)(struct esp_data*,
|
|
struct sk_buff *skb,
|
|
int offset, int len, u8 *icv);
|
|
struct crypto_hash *tfm;
|
|
} auth;
|
|
};
|
|
|
|
extern int skb_to_sgvec(struct sk_buff *skb, struct scatterlist *sg, int offset, int len);
|
|
extern int skb_cow_data(struct sk_buff *skb, int tailbits, struct sk_buff **trailer);
|
|
extern void *pskb_put(struct sk_buff *skb, struct sk_buff *tail, int len);
|
|
|
|
static inline int esp_mac_digest(struct esp_data *esp, struct sk_buff *skb,
|
|
int offset, int len)
|
|
{
|
|
struct hash_desc desc;
|
|
int err;
|
|
|
|
desc.tfm = esp->auth.tfm;
|
|
desc.flags = 0;
|
|
|
|
err = crypto_hash_init(&desc);
|
|
if (unlikely(err))
|
|
return err;
|
|
err = skb_icv_walk(skb, &desc, offset, len, crypto_hash_update);
|
|
if (unlikely(err))
|
|
return err;
|
|
return crypto_hash_final(&desc, esp->auth.work_icv);
|
|
}
|
|
|
|
#endif
|