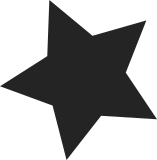
The first column (columns in the near future) are for the per line event overhead(s), that only appear when they are not zero. To clearly separate it, add back a solid vertical line, with just one colour, not influenced by the per line overheads. Then have the addr/offset column, then optionally the dynamic (static in the future) jump->target arrows, if 'j' enables it. Then the instructions. Requested-by: Peter Zijlstra <peterz@infradead.org> Cc: David Ahern <dsahern@gmail.com> Cc: Frederic Weisbecker <fweisbec@gmail.com> Cc: Mike Galbraith <efault@gmx.de> Cc: Namhyung Kim <namhyung@gmail.com> Cc: Paul Mackerras <paulus@samba.org> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Stephane Eranian <eranian@google.com> Link: http://lkml.kernel.org/n/tip-r415t4sps0oyr9y8kd9j7clz@git.kernel.org Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com>
73 lines
2.9 KiB
C
73 lines
2.9 KiB
C
#ifndef _PERF_UI_BROWSER_H_
|
|
#define _PERF_UI_BROWSER_H_ 1
|
|
|
|
#include <stdbool.h>
|
|
#include <sys/types.h>
|
|
#include "../types.h"
|
|
|
|
#define HE_COLORSET_TOP 50
|
|
#define HE_COLORSET_MEDIUM 51
|
|
#define HE_COLORSET_NORMAL 52
|
|
#define HE_COLORSET_SELECTED 53
|
|
#define HE_COLORSET_CODE 54
|
|
#define HE_COLORSET_ADDR 55
|
|
|
|
struct ui_browser {
|
|
u64 index, top_idx;
|
|
void *top, *entries;
|
|
u16 y, x, width, height;
|
|
int current_color;
|
|
void *priv;
|
|
const char *title;
|
|
char *helpline;
|
|
unsigned int (*refresh)(struct ui_browser *self);
|
|
void (*write)(struct ui_browser *self, void *entry, int row);
|
|
void (*seek)(struct ui_browser *self, off_t offset, int whence);
|
|
bool (*filter)(struct ui_browser *self, void *entry);
|
|
u32 nr_entries;
|
|
bool navkeypressed;
|
|
bool use_navkeypressed;
|
|
};
|
|
|
|
int ui_browser__set_color(struct ui_browser *browser, int color);
|
|
void ui_browser__set_percent_color(struct ui_browser *self,
|
|
double percent, bool current);
|
|
bool ui_browser__is_current_entry(struct ui_browser *self, unsigned row);
|
|
void ui_browser__refresh_dimensions(struct ui_browser *self);
|
|
void ui_browser__reset_index(struct ui_browser *self);
|
|
|
|
void ui_browser__gotorc(struct ui_browser *self, int y, int x);
|
|
void ui_browser__write_graph(struct ui_browser *browser, int graph);
|
|
void __ui_browser__line_arrow(struct ui_browser *browser, unsigned int column,
|
|
u64 start, u64 end);
|
|
void __ui_browser__show_title(struct ui_browser *browser, const char *title);
|
|
void ui_browser__show_title(struct ui_browser *browser, const char *title);
|
|
int ui_browser__show(struct ui_browser *self, const char *title,
|
|
const char *helpline, ...);
|
|
void ui_browser__hide(struct ui_browser *self);
|
|
int ui_browser__refresh(struct ui_browser *self);
|
|
int ui_browser__run(struct ui_browser *browser, int delay_secs);
|
|
void ui_browser__update_nr_entries(struct ui_browser *browser, u32 nr_entries);
|
|
void ui_browser__handle_resize(struct ui_browser *browser);
|
|
void __ui_browser__vline(struct ui_browser *browser, unsigned int column,
|
|
u16 start, u16 end);
|
|
|
|
int ui_browser__warning(struct ui_browser *browser, int timeout,
|
|
const char *format, ...);
|
|
int ui_browser__help_window(struct ui_browser *browser, const char *text);
|
|
bool ui_browser__dialog_yesno(struct ui_browser *browser, const char *text);
|
|
int ui_browser__input_window(const char *title, const char *text, char *input,
|
|
const char *exit_msg, int delay_sec);
|
|
|
|
void ui_browser__argv_seek(struct ui_browser *browser, off_t offset, int whence);
|
|
unsigned int ui_browser__argv_refresh(struct ui_browser *browser);
|
|
|
|
void ui_browser__rb_tree_seek(struct ui_browser *self, off_t offset, int whence);
|
|
unsigned int ui_browser__rb_tree_refresh(struct ui_browser *self);
|
|
|
|
void ui_browser__list_head_seek(struct ui_browser *self, off_t offset, int whence);
|
|
unsigned int ui_browser__list_head_refresh(struct ui_browser *self);
|
|
|
|
void ui_browser__init(void);
|
|
#endif /* _PERF_UI_BROWSER_H_ */
|