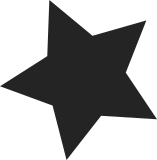
PAGE_CACHE_{SIZE,SHIFT,MASK,ALIGN} macros were introduced *long* time ago with promise that one day it will be possible to implement page cache with bigger chunks than PAGE_SIZE. This promise never materialized. And unlikely will. We have many places where PAGE_CACHE_SIZE assumed to be equal to PAGE_SIZE. And it's constant source of confusion on whether PAGE_CACHE_* or PAGE_* constant should be used in a particular case, especially on the border between fs and mm. Global switching to PAGE_CACHE_SIZE != PAGE_SIZE would cause to much breakage to be doable. Let's stop pretending that pages in page cache are special. They are not. The changes are pretty straight-forward: - <foo> << (PAGE_CACHE_SHIFT - PAGE_SHIFT) -> <foo>; - <foo> >> (PAGE_CACHE_SHIFT - PAGE_SHIFT) -> <foo>; - PAGE_CACHE_{SIZE,SHIFT,MASK,ALIGN} -> PAGE_{SIZE,SHIFT,MASK,ALIGN}; - page_cache_get() -> get_page(); - page_cache_release() -> put_page(); This patch contains automated changes generated with coccinelle using script below. For some reason, coccinelle doesn't patch header files. I've called spatch for them manually. The only adjustment after coccinelle is revert of changes to PAGE_CAHCE_ALIGN definition: we are going to drop it later. There are few places in the code where coccinelle didn't reach. I'll fix them manually in a separate patch. Comments and documentation also will be addressed with the separate patch. virtual patch @@ expression E; @@ - E << (PAGE_CACHE_SHIFT - PAGE_SHIFT) + E @@ expression E; @@ - E >> (PAGE_CACHE_SHIFT - PAGE_SHIFT) + E @@ @@ - PAGE_CACHE_SHIFT + PAGE_SHIFT @@ @@ - PAGE_CACHE_SIZE + PAGE_SIZE @@ @@ - PAGE_CACHE_MASK + PAGE_MASK @@ expression E; @@ - PAGE_CACHE_ALIGN(E) + PAGE_ALIGN(E) @@ expression E; @@ - page_cache_get(E) + get_page(E) @@ expression E; @@ - page_cache_release(E) + put_page(E) Signed-off-by: Kirill A. Shutemov <kirill.shutemov@linux.intel.com> Acked-by: Michal Hocko <mhocko@suse.com> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
176 lines
4.6 KiB
C
176 lines
4.6 KiB
C
/* -*- mode: c; c-basic-offset: 8; -*-
|
|
* vim: noexpandtab sw=8 ts=8 sts=0:
|
|
*
|
|
* mount.c - operations for initializing and mounting configfs.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public
|
|
* License along with this program; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 021110-1307, USA.
|
|
*
|
|
* Based on sysfs:
|
|
* sysfs is Copyright (C) 2001, 2002, 2003 Patrick Mochel
|
|
*
|
|
* configfs Copyright (C) 2005 Oracle. All rights reserved.
|
|
*/
|
|
|
|
#include <linux/fs.h>
|
|
#include <linux/module.h>
|
|
#include <linux/mount.h>
|
|
#include <linux/pagemap.h>
|
|
#include <linux/init.h>
|
|
#include <linux/slab.h>
|
|
|
|
#include <linux/configfs.h>
|
|
#include "configfs_internal.h"
|
|
|
|
/* Random magic number */
|
|
#define CONFIGFS_MAGIC 0x62656570
|
|
|
|
static struct vfsmount *configfs_mount = NULL;
|
|
struct kmem_cache *configfs_dir_cachep;
|
|
static int configfs_mnt_count = 0;
|
|
|
|
static const struct super_operations configfs_ops = {
|
|
.statfs = simple_statfs,
|
|
.drop_inode = generic_delete_inode,
|
|
};
|
|
|
|
static struct config_group configfs_root_group = {
|
|
.cg_item = {
|
|
.ci_namebuf = "root",
|
|
.ci_name = configfs_root_group.cg_item.ci_namebuf,
|
|
},
|
|
};
|
|
|
|
int configfs_is_root(struct config_item *item)
|
|
{
|
|
return item == &configfs_root_group.cg_item;
|
|
}
|
|
|
|
static struct configfs_dirent configfs_root = {
|
|
.s_sibling = LIST_HEAD_INIT(configfs_root.s_sibling),
|
|
.s_children = LIST_HEAD_INIT(configfs_root.s_children),
|
|
.s_element = &configfs_root_group.cg_item,
|
|
.s_type = CONFIGFS_ROOT,
|
|
.s_iattr = NULL,
|
|
};
|
|
|
|
static int configfs_fill_super(struct super_block *sb, void *data, int silent)
|
|
{
|
|
struct inode *inode;
|
|
struct dentry *root;
|
|
|
|
sb->s_blocksize = PAGE_SIZE;
|
|
sb->s_blocksize_bits = PAGE_SHIFT;
|
|
sb->s_magic = CONFIGFS_MAGIC;
|
|
sb->s_op = &configfs_ops;
|
|
sb->s_time_gran = 1;
|
|
|
|
inode = configfs_new_inode(S_IFDIR | S_IRWXU | S_IRUGO | S_IXUGO,
|
|
&configfs_root, sb);
|
|
if (inode) {
|
|
inode->i_op = &configfs_root_inode_operations;
|
|
inode->i_fop = &configfs_dir_operations;
|
|
/* directory inodes start off with i_nlink == 2 (for "." entry) */
|
|
inc_nlink(inode);
|
|
} else {
|
|
pr_debug("could not get root inode\n");
|
|
return -ENOMEM;
|
|
}
|
|
|
|
root = d_make_root(inode);
|
|
if (!root) {
|
|
pr_debug("%s: could not get root dentry!\n",__func__);
|
|
return -ENOMEM;
|
|
}
|
|
config_group_init(&configfs_root_group);
|
|
configfs_root_group.cg_item.ci_dentry = root;
|
|
root->d_fsdata = &configfs_root;
|
|
sb->s_root = root;
|
|
sb->s_d_op = &configfs_dentry_ops; /* the rest get that */
|
|
return 0;
|
|
}
|
|
|
|
static struct dentry *configfs_do_mount(struct file_system_type *fs_type,
|
|
int flags, const char *dev_name, void *data)
|
|
{
|
|
return mount_single(fs_type, flags, data, configfs_fill_super);
|
|
}
|
|
|
|
static struct file_system_type configfs_fs_type = {
|
|
.owner = THIS_MODULE,
|
|
.name = "configfs",
|
|
.mount = configfs_do_mount,
|
|
.kill_sb = kill_litter_super,
|
|
};
|
|
MODULE_ALIAS_FS("configfs");
|
|
|
|
struct dentry *configfs_pin_fs(void)
|
|
{
|
|
int err = simple_pin_fs(&configfs_fs_type, &configfs_mount,
|
|
&configfs_mnt_count);
|
|
return err ? ERR_PTR(err) : configfs_mount->mnt_root;
|
|
}
|
|
|
|
void configfs_release_fs(void)
|
|
{
|
|
simple_release_fs(&configfs_mount, &configfs_mnt_count);
|
|
}
|
|
|
|
|
|
static int __init configfs_init(void)
|
|
{
|
|
int err = -ENOMEM;
|
|
|
|
configfs_dir_cachep = kmem_cache_create("configfs_dir_cache",
|
|
sizeof(struct configfs_dirent),
|
|
0, 0, NULL);
|
|
if (!configfs_dir_cachep)
|
|
goto out;
|
|
|
|
err = sysfs_create_mount_point(kernel_kobj, "config");
|
|
if (err)
|
|
goto out2;
|
|
|
|
err = register_filesystem(&configfs_fs_type);
|
|
if (err)
|
|
goto out3;
|
|
|
|
return 0;
|
|
out3:
|
|
pr_err("Unable to register filesystem!\n");
|
|
sysfs_remove_mount_point(kernel_kobj, "config");
|
|
out2:
|
|
kmem_cache_destroy(configfs_dir_cachep);
|
|
configfs_dir_cachep = NULL;
|
|
out:
|
|
return err;
|
|
}
|
|
|
|
static void __exit configfs_exit(void)
|
|
{
|
|
unregister_filesystem(&configfs_fs_type);
|
|
sysfs_remove_mount_point(kernel_kobj, "config");
|
|
kmem_cache_destroy(configfs_dir_cachep);
|
|
configfs_dir_cachep = NULL;
|
|
}
|
|
|
|
MODULE_AUTHOR("Oracle");
|
|
MODULE_LICENSE("GPL");
|
|
MODULE_VERSION("0.0.2");
|
|
MODULE_DESCRIPTION("Simple RAM filesystem for user driven kernel subsystem configuration.");
|
|
|
|
core_initcall(configfs_init);
|
|
module_exit(configfs_exit);
|