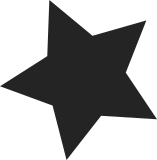
If the RAMOOPS_FLAG_FTRACE_PER_CPU flag is passed to ramoops pdata, split the ftrace space into multiple zones depending on the number of CPUs. This speeds up the performance of function tracing by about 280% in my tests as we avoid the locking. The trade off being lesser space available per CPU. Let the ramoops user decide which option they want based on pdata flag. Signed-off-by: Joel Fernandes <joelaf@google.com> [kees: added max_ftrace_cnt to track size, added DT logic and docs] Signed-off-by: Kees Cook <keescook@chromium.org>
105 lines
2.9 KiB
C
105 lines
2.9 KiB
C
/*
|
|
* Copyright (C) 2010 Marco Stornelli <marco.stornelli@gmail.com>
|
|
* Copyright (C) 2011 Kees Cook <keescook@chromium.org>
|
|
* Copyright (C) 2011 Google, Inc.
|
|
*
|
|
* This software is licensed under the terms of the GNU General Public
|
|
* License version 2, as published by the Free Software Foundation, and
|
|
* may be copied, distributed, and modified under those terms.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
*/
|
|
|
|
#ifndef __LINUX_PSTORE_RAM_H__
|
|
#define __LINUX_PSTORE_RAM_H__
|
|
|
|
#include <linux/compiler.h>
|
|
#include <linux/device.h>
|
|
#include <linux/init.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/list.h>
|
|
#include <linux/types.h>
|
|
|
|
/*
|
|
* Choose whether access to the RAM zone requires locking or not. If a zone
|
|
* can be written to from different CPUs like with ftrace for example, then
|
|
* PRZ_FLAG_NO_LOCK is used. For all other cases, locking is required.
|
|
*/
|
|
#define PRZ_FLAG_NO_LOCK BIT(0)
|
|
|
|
struct persistent_ram_buffer;
|
|
struct rs_control;
|
|
|
|
struct persistent_ram_ecc_info {
|
|
int block_size;
|
|
int ecc_size;
|
|
int symsize;
|
|
int poly;
|
|
};
|
|
|
|
struct persistent_ram_zone {
|
|
phys_addr_t paddr;
|
|
size_t size;
|
|
void *vaddr;
|
|
struct persistent_ram_buffer *buffer;
|
|
size_t buffer_size;
|
|
u32 flags;
|
|
raw_spinlock_t buffer_lock;
|
|
|
|
/* ECC correction */
|
|
char *par_buffer;
|
|
char *par_header;
|
|
struct rs_control *rs_decoder;
|
|
int corrected_bytes;
|
|
int bad_blocks;
|
|
struct persistent_ram_ecc_info ecc_info;
|
|
|
|
char *old_log;
|
|
size_t old_log_size;
|
|
};
|
|
|
|
struct persistent_ram_zone *persistent_ram_new(phys_addr_t start, size_t size,
|
|
u32 sig, struct persistent_ram_ecc_info *ecc_info,
|
|
unsigned int memtype, u32 flags);
|
|
void persistent_ram_free(struct persistent_ram_zone *prz);
|
|
void persistent_ram_zap(struct persistent_ram_zone *prz);
|
|
|
|
int persistent_ram_write(struct persistent_ram_zone *prz, const void *s,
|
|
unsigned int count);
|
|
int persistent_ram_write_user(struct persistent_ram_zone *prz,
|
|
const void __user *s, unsigned int count);
|
|
|
|
void persistent_ram_save_old(struct persistent_ram_zone *prz);
|
|
size_t persistent_ram_old_size(struct persistent_ram_zone *prz);
|
|
void *persistent_ram_old(struct persistent_ram_zone *prz);
|
|
void persistent_ram_free_old(struct persistent_ram_zone *prz);
|
|
ssize_t persistent_ram_ecc_string(struct persistent_ram_zone *prz,
|
|
char *str, size_t len);
|
|
|
|
/*
|
|
* Ramoops platform data
|
|
* @mem_size memory size for ramoops
|
|
* @mem_address physical memory address to contain ramoops
|
|
*/
|
|
|
|
#define RAMOOPS_FLAG_FTRACE_PER_CPU BIT(0)
|
|
|
|
struct ramoops_platform_data {
|
|
unsigned long mem_size;
|
|
phys_addr_t mem_address;
|
|
unsigned int mem_type;
|
|
unsigned long record_size;
|
|
unsigned long console_size;
|
|
unsigned long ftrace_size;
|
|
unsigned long pmsg_size;
|
|
int dump_oops;
|
|
u32 flags;
|
|
struct persistent_ram_ecc_info ecc_info;
|
|
};
|
|
|
|
#endif
|