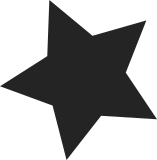
Currently only CPU devices use the transition latency and the OPPs populated in the SCPI driver. scpi-cpufreq has logic to handle these. However, even GPU and other users of SCPI DVFS will need the same logic. In order to avoid duplication, this patch adds support to get DVFS transition latency and add all the OPPs to the device using OPP library helper functions. The helper functions added here can be used for any device whose DVFS are managed by SCPI. Also, we also have incorrect dependency on the cluster identifier for the CPUs. It's fundamentally wrong as the domain id need not match the cluster id. This patch gets rid of that dependency by making use of the clock bindings which are already in place. Signed-off-by: Sudeep Holla <sudeep.holla@arm.com>
85 lines
2.6 KiB
C
85 lines
2.6 KiB
C
/*
|
|
* SCPI Message Protocol driver header
|
|
*
|
|
* Copyright (C) 2014 ARM Ltd.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms and conditions of the GNU General Public License,
|
|
* version 2, as published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope it will be useful, but WITHOUT
|
|
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
|
|
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for
|
|
* more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along with
|
|
* this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
#include <linux/types.h>
|
|
|
|
struct scpi_opp {
|
|
u32 freq;
|
|
u32 m_volt;
|
|
} __packed;
|
|
|
|
struct scpi_dvfs_info {
|
|
unsigned int count;
|
|
unsigned int latency; /* in nanoseconds */
|
|
struct scpi_opp *opps;
|
|
};
|
|
|
|
enum scpi_sensor_class {
|
|
TEMPERATURE,
|
|
VOLTAGE,
|
|
CURRENT,
|
|
POWER,
|
|
ENERGY,
|
|
};
|
|
|
|
struct scpi_sensor_info {
|
|
u16 sensor_id;
|
|
u8 class;
|
|
u8 trigger_type;
|
|
char name[20];
|
|
} __packed;
|
|
|
|
/**
|
|
* struct scpi_ops - represents the various operations provided
|
|
* by SCP through SCPI message protocol
|
|
* @get_version: returns the major and minor revision on the SCPI
|
|
* message protocol
|
|
* @clk_get_range: gets clock range limit(min - max in Hz)
|
|
* @clk_get_val: gets clock value(in Hz)
|
|
* @clk_set_val: sets the clock value, setting to 0 will disable the
|
|
* clock (if supported)
|
|
* @dvfs_get_idx: gets the Operating Point of the given power domain.
|
|
* OPP is an index to the list return by @dvfs_get_info
|
|
* @dvfs_set_idx: sets the Operating Point of the given power domain.
|
|
* OPP is an index to the list return by @dvfs_get_info
|
|
* @dvfs_get_info: returns the DVFS capabilities of the given power
|
|
* domain. It includes the OPP list and the latency information
|
|
*/
|
|
struct scpi_ops {
|
|
u32 (*get_version)(void);
|
|
int (*clk_get_range)(u16, unsigned long *, unsigned long *);
|
|
unsigned long (*clk_get_val)(u16);
|
|
int (*clk_set_val)(u16, unsigned long);
|
|
int (*dvfs_get_idx)(u8);
|
|
int (*dvfs_set_idx)(u8, u8);
|
|
struct scpi_dvfs_info *(*dvfs_get_info)(u8);
|
|
int (*device_domain_id)(struct device *);
|
|
int (*get_transition_latency)(struct device *);
|
|
int (*add_opps_to_device)(struct device *);
|
|
int (*sensor_get_capability)(u16 *sensors);
|
|
int (*sensor_get_info)(u16 sensor_id, struct scpi_sensor_info *);
|
|
int (*sensor_get_value)(u16, u64 *);
|
|
int (*device_get_power_state)(u16);
|
|
int (*device_set_power_state)(u16, u8);
|
|
};
|
|
|
|
#if IS_REACHABLE(CONFIG_ARM_SCPI_PROTOCOL)
|
|
struct scpi_ops *get_scpi_ops(void);
|
|
#else
|
|
static inline struct scpi_ops *get_scpi_ops(void) { return NULL; }
|
|
#endif
|