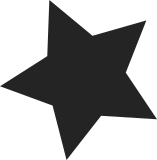
Currently various places in the VFS call vfs_dq_init directly. This means we tie the quota code into the VFS. Get rid of that and make the filesystem responsible for the initialization. For most metadata operations this is a straight forward move into the methods, but for truncate and open it's a bit more complicated. For truncate we currently only call vfs_dq_init for the sys_truncate case because open already takes care of it for ftruncate and open(O_TRUNC) - the new code causes an additional vfs_dq_init for those which is harmless. For open the initialization is moved from do_filp_open into the open method, which means it happens slightly earlier now, and only for regular files. The latter is fine because we don't need to initialize it for operations on special files, and we already do it as part of the namespace operations for directories. Add a dquot_file_open helper that filesystems that support generic quotas can use to fill in ->open. Signed-off-by: Christoph Hellwig <hch@lst.de> Signed-off-by: Jan Kara <jack@suse.cz>
108 lines
2.7 KiB
C
108 lines
2.7 KiB
C
/*
|
|
* linux/fs/ext2/file.c
|
|
*
|
|
* Copyright (C) 1992, 1993, 1994, 1995
|
|
* Remy Card (card@masi.ibp.fr)
|
|
* Laboratoire MASI - Institut Blaise Pascal
|
|
* Universite Pierre et Marie Curie (Paris VI)
|
|
*
|
|
* from
|
|
*
|
|
* linux/fs/minix/file.c
|
|
*
|
|
* Copyright (C) 1991, 1992 Linus Torvalds
|
|
*
|
|
* ext2 fs regular file handling primitives
|
|
*
|
|
* 64-bit file support on 64-bit platforms by Jakub Jelinek
|
|
* (jj@sunsite.ms.mff.cuni.cz)
|
|
*/
|
|
|
|
#include <linux/time.h>
|
|
#include <linux/pagemap.h>
|
|
#include "ext2.h"
|
|
#include "xattr.h"
|
|
#include "acl.h"
|
|
|
|
/*
|
|
* Called when filp is released. This happens when all file descriptors
|
|
* for a single struct file are closed. Note that different open() calls
|
|
* for the same file yield different struct file structures.
|
|
*/
|
|
static int ext2_release_file (struct inode * inode, struct file * filp)
|
|
{
|
|
if (filp->f_mode & FMODE_WRITE) {
|
|
mutex_lock(&EXT2_I(inode)->truncate_mutex);
|
|
ext2_discard_reservation(inode);
|
|
mutex_unlock(&EXT2_I(inode)->truncate_mutex);
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
int ext2_fsync(struct file *file, struct dentry *dentry, int datasync)
|
|
{
|
|
int ret;
|
|
struct super_block *sb = dentry->d_inode->i_sb;
|
|
struct address_space *mapping = sb->s_bdev->bd_inode->i_mapping;
|
|
|
|
ret = simple_fsync(file, dentry, datasync);
|
|
if (ret == -EIO || test_and_clear_bit(AS_EIO, &mapping->flags)) {
|
|
/* We don't really know where the IO error happened... */
|
|
ext2_error(sb, __func__,
|
|
"detected IO error when writing metadata buffers");
|
|
ret = -EIO;
|
|
}
|
|
return ret;
|
|
}
|
|
|
|
/*
|
|
* We have mostly NULL's here: the current defaults are ok for
|
|
* the ext2 filesystem.
|
|
*/
|
|
const struct file_operations ext2_file_operations = {
|
|
.llseek = generic_file_llseek,
|
|
.read = do_sync_read,
|
|
.write = do_sync_write,
|
|
.aio_read = generic_file_aio_read,
|
|
.aio_write = generic_file_aio_write,
|
|
.unlocked_ioctl = ext2_ioctl,
|
|
#ifdef CONFIG_COMPAT
|
|
.compat_ioctl = ext2_compat_ioctl,
|
|
#endif
|
|
.mmap = generic_file_mmap,
|
|
.open = dquot_file_open,
|
|
.release = ext2_release_file,
|
|
.fsync = ext2_fsync,
|
|
.splice_read = generic_file_splice_read,
|
|
.splice_write = generic_file_splice_write,
|
|
};
|
|
|
|
#ifdef CONFIG_EXT2_FS_XIP
|
|
const struct file_operations ext2_xip_file_operations = {
|
|
.llseek = generic_file_llseek,
|
|
.read = xip_file_read,
|
|
.write = xip_file_write,
|
|
.unlocked_ioctl = ext2_ioctl,
|
|
#ifdef CONFIG_COMPAT
|
|
.compat_ioctl = ext2_compat_ioctl,
|
|
#endif
|
|
.mmap = xip_file_mmap,
|
|
.open = dquot_file_open,
|
|
.release = ext2_release_file,
|
|
.fsync = ext2_fsync,
|
|
};
|
|
#endif
|
|
|
|
const struct inode_operations ext2_file_inode_operations = {
|
|
.truncate = ext2_truncate,
|
|
#ifdef CONFIG_EXT2_FS_XATTR
|
|
.setxattr = generic_setxattr,
|
|
.getxattr = generic_getxattr,
|
|
.listxattr = ext2_listxattr,
|
|
.removexattr = generic_removexattr,
|
|
#endif
|
|
.setattr = ext2_setattr,
|
|
.check_acl = ext2_check_acl,
|
|
.fiemap = ext2_fiemap,
|
|
};
|