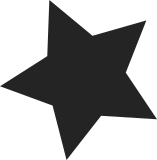
After running SetPageUptodate, preceeding stores to the page contents to actually bring it uptodate may not be ordered with the store to set the page uptodate. Therefore, another CPU which checks PageUptodate is true, then reads the page contents can get stale data. Fix this by having an smp_wmb before SetPageUptodate, and smp_rmb after PageUptodate. Many places that test PageUptodate, do so with the page locked, and this would be enough to ensure memory ordering in those places if SetPageUptodate were only called while the page is locked. Unfortunately that is not always the case for some filesystems, but it could be an idea for the future. Also bring the handling of anonymous page uptodateness in line with that of file backed page management, by marking anon pages as uptodate when they _are_ uptodate, rather than when our implementation requires that they be marked as such. Doing allows us to get rid of the smp_wmb's in the page copying functions, which were especially added for anonymous pages for an analogous memory ordering problem. Both file and anonymous pages are handled with the same barriers. FAQ: Q. Why not do this in flush_dcache_page? A. Firstly, flush_dcache_page handles only one side (the smb side) of the ordering protocol; we'd still need smp_rmb somewhere. Secondly, hiding away memory barriers in a completely unrelated function is nasty; at least in the PageUptodate macros, they are located together with (half) the operations involved in the ordering. Thirdly, the smp_wmb is only required when first bringing the page uptodate, wheras flush_dcache_page should be called each time it is written to through the kernel mapping. It is logically the wrong place to put it. Q. Why does this increase my text size / reduce my performance / etc. A. Because it is adding the necessary instructions to eliminate the data-race. Q. Can it be improved? A. Yes, eg. if you were to create a rule that all SetPageUptodate operations run under the page lock, we could avoid the smp_rmb places where PageUptodate is queried under the page lock. Requires audit of all filesystems and at least some would need reworking. That's great you're interested, I'm eagerly awaiting your patches. Signed-off-by: Nick Piggin <npiggin@suse.de> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
142 lines
3.4 KiB
C
142 lines
3.4 KiB
C
/*
|
|
* linux/mm/page_io.c
|
|
*
|
|
* Copyright (C) 1991, 1992, 1993, 1994 Linus Torvalds
|
|
*
|
|
* Swap reorganised 29.12.95,
|
|
* Asynchronous swapping added 30.12.95. Stephen Tweedie
|
|
* Removed race in async swapping. 14.4.1996. Bruno Haible
|
|
* Add swap of shared pages through the page cache. 20.2.1998. Stephen Tweedie
|
|
* Always use brw_page, life becomes simpler. 12 May 1998 Eric Biederman
|
|
*/
|
|
|
|
#include <linux/mm.h>
|
|
#include <linux/kernel_stat.h>
|
|
#include <linux/pagemap.h>
|
|
#include <linux/swap.h>
|
|
#include <linux/bio.h>
|
|
#include <linux/swapops.h>
|
|
#include <linux/writeback.h>
|
|
#include <asm/pgtable.h>
|
|
|
|
static struct bio *get_swap_bio(gfp_t gfp_flags, pgoff_t index,
|
|
struct page *page, bio_end_io_t end_io)
|
|
{
|
|
struct bio *bio;
|
|
|
|
bio = bio_alloc(gfp_flags, 1);
|
|
if (bio) {
|
|
struct swap_info_struct *sis;
|
|
swp_entry_t entry = { .val = index, };
|
|
|
|
sis = get_swap_info_struct(swp_type(entry));
|
|
bio->bi_sector = map_swap_page(sis, swp_offset(entry)) *
|
|
(PAGE_SIZE >> 9);
|
|
bio->bi_bdev = sis->bdev;
|
|
bio->bi_io_vec[0].bv_page = page;
|
|
bio->bi_io_vec[0].bv_len = PAGE_SIZE;
|
|
bio->bi_io_vec[0].bv_offset = 0;
|
|
bio->bi_vcnt = 1;
|
|
bio->bi_idx = 0;
|
|
bio->bi_size = PAGE_SIZE;
|
|
bio->bi_end_io = end_io;
|
|
}
|
|
return bio;
|
|
}
|
|
|
|
static void end_swap_bio_write(struct bio *bio, int err)
|
|
{
|
|
const int uptodate = test_bit(BIO_UPTODATE, &bio->bi_flags);
|
|
struct page *page = bio->bi_io_vec[0].bv_page;
|
|
|
|
if (!uptodate) {
|
|
SetPageError(page);
|
|
/*
|
|
* We failed to write the page out to swap-space.
|
|
* Re-dirty the page in order to avoid it being reclaimed.
|
|
* Also print a dire warning that things will go BAD (tm)
|
|
* very quickly.
|
|
*
|
|
* Also clear PG_reclaim to avoid rotate_reclaimable_page()
|
|
*/
|
|
set_page_dirty(page);
|
|
printk(KERN_ALERT "Write-error on swap-device (%u:%u:%Lu)\n",
|
|
imajor(bio->bi_bdev->bd_inode),
|
|
iminor(bio->bi_bdev->bd_inode),
|
|
(unsigned long long)bio->bi_sector);
|
|
ClearPageReclaim(page);
|
|
}
|
|
end_page_writeback(page);
|
|
bio_put(bio);
|
|
}
|
|
|
|
void end_swap_bio_read(struct bio *bio, int err)
|
|
{
|
|
const int uptodate = test_bit(BIO_UPTODATE, &bio->bi_flags);
|
|
struct page *page = bio->bi_io_vec[0].bv_page;
|
|
|
|
if (!uptodate) {
|
|
SetPageError(page);
|
|
ClearPageUptodate(page);
|
|
printk(KERN_ALERT "Read-error on swap-device (%u:%u:%Lu)\n",
|
|
imajor(bio->bi_bdev->bd_inode),
|
|
iminor(bio->bi_bdev->bd_inode),
|
|
(unsigned long long)bio->bi_sector);
|
|
} else {
|
|
SetPageUptodate(page);
|
|
}
|
|
unlock_page(page);
|
|
bio_put(bio);
|
|
}
|
|
|
|
/*
|
|
* We may have stale swap cache pages in memory: notice
|
|
* them here and get rid of the unnecessary final write.
|
|
*/
|
|
int swap_writepage(struct page *page, struct writeback_control *wbc)
|
|
{
|
|
struct bio *bio;
|
|
int ret = 0, rw = WRITE;
|
|
|
|
if (remove_exclusive_swap_page(page)) {
|
|
unlock_page(page);
|
|
goto out;
|
|
}
|
|
bio = get_swap_bio(GFP_NOIO, page_private(page), page,
|
|
end_swap_bio_write);
|
|
if (bio == NULL) {
|
|
set_page_dirty(page);
|
|
unlock_page(page);
|
|
ret = -ENOMEM;
|
|
goto out;
|
|
}
|
|
if (wbc->sync_mode == WB_SYNC_ALL)
|
|
rw |= (1 << BIO_RW_SYNC);
|
|
count_vm_event(PSWPOUT);
|
|
set_page_writeback(page);
|
|
unlock_page(page);
|
|
submit_bio(rw, bio);
|
|
out:
|
|
return ret;
|
|
}
|
|
|
|
int swap_readpage(struct file *file, struct page *page)
|
|
{
|
|
struct bio *bio;
|
|
int ret = 0;
|
|
|
|
BUG_ON(!PageLocked(page));
|
|
BUG_ON(PageUptodate(page));
|
|
bio = get_swap_bio(GFP_KERNEL, page_private(page), page,
|
|
end_swap_bio_read);
|
|
if (bio == NULL) {
|
|
unlock_page(page);
|
|
ret = -ENOMEM;
|
|
goto out;
|
|
}
|
|
count_vm_event(PSWPIN);
|
|
submit_bio(READ, bio);
|
|
out:
|
|
return ret;
|
|
}
|