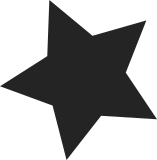
Per SD specification physical layer v4.0, section 3.9.4, it says "UHS-I supports only 4-bit mode. Host shall select 4-bit mode by ACMD6. However mmc_sd_init_uhs_card() still go ahead to initialize the cards anyway, whether card or host won't support 4-bit mode. This breaks the platforms which could support UHS-I mode but on some certain boards only support 1-bit mode with a UHS-I card inserted, as all the tuning process is broken due to this. Alternatively, we should check the return value from mmc_set_bus_width() to see if host could finish the request to switch the bus width on its side. But that needs more thing to do than this patch that just bails out early to try high speed mode if 4-bit mode isn't available for whatever reason. And this patch could also fix the same problem for sdio since R4_18V_PRESENT won't be set for ocr when mmc_sdio_init_card() finds mmc_host_uhs() is false. Note that this patch doesn't keep the checking of card->scr.sda_spec3 and comparing card->scr.bus_widths with SD_SCR_BUS_WIDTH_4 within mmc_sd_init_uhs_card() since if the sd cards response with SD_ROCR_S18A, it definitely supports UHS-I mode, which implicitly means these checkings are always true. Signed-off-by: Shawn Lin <shawn.lin@rock-chips.com> Signed-off-by: Ulf Hansson <ulf.hansson@linaro.org>
85 lines
2 KiB
C
85 lines
2 KiB
C
/*
|
|
* linux/drivers/mmc/core/host.h
|
|
*
|
|
* Copyright (C) 2003 Russell King, All Rights Reserved.
|
|
* Copyright 2007 Pierre Ossman
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
#ifndef _MMC_CORE_HOST_H
|
|
#define _MMC_CORE_HOST_H
|
|
|
|
#include <linux/mmc/host.h>
|
|
|
|
int mmc_register_host_class(void);
|
|
void mmc_unregister_host_class(void);
|
|
|
|
void mmc_retune_enable(struct mmc_host *host);
|
|
void mmc_retune_disable(struct mmc_host *host);
|
|
void mmc_retune_hold(struct mmc_host *host);
|
|
void mmc_retune_release(struct mmc_host *host);
|
|
int mmc_retune(struct mmc_host *host);
|
|
void mmc_retune_pause(struct mmc_host *host);
|
|
void mmc_retune_unpause(struct mmc_host *host);
|
|
|
|
static inline void mmc_retune_hold_now(struct mmc_host *host)
|
|
{
|
|
host->retune_now = 0;
|
|
host->hold_retune += 1;
|
|
}
|
|
|
|
static inline void mmc_retune_recheck(struct mmc_host *host)
|
|
{
|
|
if (host->hold_retune <= 1)
|
|
host->retune_now = 1;
|
|
}
|
|
|
|
static inline int mmc_host_cmd23(struct mmc_host *host)
|
|
{
|
|
return host->caps & MMC_CAP_CMD23;
|
|
}
|
|
|
|
static inline bool mmc_host_done_complete(struct mmc_host *host)
|
|
{
|
|
return host->caps & MMC_CAP_DONE_COMPLETE;
|
|
}
|
|
|
|
static inline int mmc_boot_partition_access(struct mmc_host *host)
|
|
{
|
|
return !(host->caps2 & MMC_CAP2_BOOTPART_NOACC);
|
|
}
|
|
|
|
static inline int mmc_host_uhs(struct mmc_host *host)
|
|
{
|
|
return host->caps &
|
|
(MMC_CAP_UHS_SDR12 | MMC_CAP_UHS_SDR25 |
|
|
MMC_CAP_UHS_SDR50 | MMC_CAP_UHS_SDR104 |
|
|
MMC_CAP_UHS_DDR50) &&
|
|
host->caps & MMC_CAP_4_BIT_DATA;
|
|
}
|
|
|
|
static inline bool mmc_card_hs200(struct mmc_card *card)
|
|
{
|
|
return card->host->ios.timing == MMC_TIMING_MMC_HS200;
|
|
}
|
|
|
|
static inline bool mmc_card_ddr52(struct mmc_card *card)
|
|
{
|
|
return card->host->ios.timing == MMC_TIMING_MMC_DDR52;
|
|
}
|
|
|
|
static inline bool mmc_card_hs400(struct mmc_card *card)
|
|
{
|
|
return card->host->ios.timing == MMC_TIMING_MMC_HS400;
|
|
}
|
|
|
|
static inline bool mmc_card_hs400es(struct mmc_card *card)
|
|
{
|
|
return card->host->ios.enhanced_strobe;
|
|
}
|
|
|
|
#endif
|
|
|