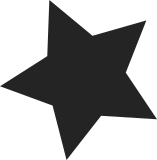
Export the context state: whether we run in user / kernel from the context tracking subsystem point of view. This is going to be used by the generic virtual cputime accounting subsystem that is needed to implement the full dynticks. Signed-off-by: Frederic Weisbecker <fweisbec@gmail.com> Cc: Andrew Morton <akpm@linux-foundation.org> Cc: Ingo Molnar <mingo@kernel.org> Cc: Li Zhong <zhong@linux.vnet.ibm.com> Cc: Namhyung Kim <namhyung.kim@lge.com> Cc: Paul E. McKenney <paulmck@linux.vnet.ibm.com> Cc: Paul Gortmaker <paul.gortmaker@windriver.com> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Steven Rostedt <rostedt@goodmis.org> Cc: Thomas Gleixner <tglx@linutronix.de>
70 lines
1.8 KiB
C
70 lines
1.8 KiB
C
#include <linux/context_tracking.h>
|
|
#include <linux/rcupdate.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/hardirq.h>
|
|
|
|
|
|
DEFINE_PER_CPU(struct context_tracking, context_tracking) = {
|
|
#ifdef CONFIG_CONTEXT_TRACKING_FORCE
|
|
.active = true,
|
|
#endif
|
|
};
|
|
|
|
void user_enter(void)
|
|
{
|
|
unsigned long flags;
|
|
|
|
/*
|
|
* Some contexts may involve an exception occuring in an irq,
|
|
* leading to that nesting:
|
|
* rcu_irq_enter() rcu_user_exit() rcu_user_exit() rcu_irq_exit()
|
|
* This would mess up the dyntick_nesting count though. And rcu_irq_*()
|
|
* helpers are enough to protect RCU uses inside the exception. So
|
|
* just return immediately if we detect we are in an IRQ.
|
|
*/
|
|
if (in_interrupt())
|
|
return;
|
|
|
|
WARN_ON_ONCE(!current->mm);
|
|
|
|
local_irq_save(flags);
|
|
if (__this_cpu_read(context_tracking.active) &&
|
|
__this_cpu_read(context_tracking.state) != IN_USER) {
|
|
__this_cpu_write(context_tracking.state, IN_USER);
|
|
rcu_user_enter();
|
|
}
|
|
local_irq_restore(flags);
|
|
}
|
|
|
|
void user_exit(void)
|
|
{
|
|
unsigned long flags;
|
|
|
|
/*
|
|
* Some contexts may involve an exception occuring in an irq,
|
|
* leading to that nesting:
|
|
* rcu_irq_enter() rcu_user_exit() rcu_user_exit() rcu_irq_exit()
|
|
* This would mess up the dyntick_nesting count though. And rcu_irq_*()
|
|
* helpers are enough to protect RCU uses inside the exception. So
|
|
* just return immediately if we detect we are in an IRQ.
|
|
*/
|
|
if (in_interrupt())
|
|
return;
|
|
|
|
local_irq_save(flags);
|
|
if (__this_cpu_read(context_tracking.state) == IN_USER) {
|
|
__this_cpu_write(context_tracking.state, IN_KERNEL);
|
|
rcu_user_exit();
|
|
}
|
|
local_irq_restore(flags);
|
|
}
|
|
|
|
void context_tracking_task_switch(struct task_struct *prev,
|
|
struct task_struct *next)
|
|
{
|
|
if (__this_cpu_read(context_tracking.active)) {
|
|
clear_tsk_thread_flag(prev, TIF_NOHZ);
|
|
set_tsk_thread_flag(next, TIF_NOHZ);
|
|
}
|
|
}
|