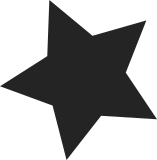
This patch adds an iterator function for the idr data structure. Compared to just iterating through the idr with an integer and idr_find, this iterator is (almost, but not quite) linear in the number of elements, as opposed to the number of integers in the range covered by the idr. This makes a difference for sparse idrs, but more importantly, it's a nicer way to iterate through the elements. The drm subsystem is moving to idr for tracking contexts and drawables, and with this change, we can use the idr exclusively for tracking these resources. [akpm@linux-foundation.org: fix comment] Signed-off-by: Kristian Hoegsberg <krh@redhat.com> Cc: Tejun Heo <htejun@gmail.com> Cc: Dave Airlie <airlied@linux.ie> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
118 lines
3.3 KiB
C
118 lines
3.3 KiB
C
/*
|
|
* include/linux/idr.h
|
|
*
|
|
* 2002-10-18 written by Jim Houston jim.houston@ccur.com
|
|
* Copyright (C) 2002 by Concurrent Computer Corporation
|
|
* Distributed under the GNU GPL license version 2.
|
|
*
|
|
* Small id to pointer translation service avoiding fixed sized
|
|
* tables.
|
|
*/
|
|
|
|
#ifndef __IDR_H__
|
|
#define __IDR_H__
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/bitops.h>
|
|
|
|
#if BITS_PER_LONG == 32
|
|
# define IDR_BITS 5
|
|
# define IDR_FULL 0xfffffffful
|
|
/* We can only use two of the bits in the top level because there is
|
|
only one possible bit in the top level (5 bits * 7 levels = 35
|
|
bits, but you only use 31 bits in the id). */
|
|
# define TOP_LEVEL_FULL (IDR_FULL >> 30)
|
|
#elif BITS_PER_LONG == 64
|
|
# define IDR_BITS 6
|
|
# define IDR_FULL 0xfffffffffffffffful
|
|
/* We can only use two of the bits in the top level because there is
|
|
only one possible bit in the top level (6 bits * 6 levels = 36
|
|
bits, but you only use 31 bits in the id). */
|
|
# define TOP_LEVEL_FULL (IDR_FULL >> 62)
|
|
#else
|
|
# error "BITS_PER_LONG is not 32 or 64"
|
|
#endif
|
|
|
|
#define IDR_SIZE (1 << IDR_BITS)
|
|
#define IDR_MASK ((1 << IDR_BITS)-1)
|
|
|
|
#define MAX_ID_SHIFT (sizeof(int)*8 - 1)
|
|
#define MAX_ID_BIT (1U << MAX_ID_SHIFT)
|
|
#define MAX_ID_MASK (MAX_ID_BIT - 1)
|
|
|
|
/* Leave the possibility of an incomplete final layer */
|
|
#define MAX_LEVEL (MAX_ID_SHIFT + IDR_BITS - 1) / IDR_BITS
|
|
|
|
/* Number of id_layer structs to leave in free list */
|
|
#define IDR_FREE_MAX MAX_LEVEL + MAX_LEVEL
|
|
|
|
struct idr_layer {
|
|
unsigned long bitmap; /* A zero bit means "space here" */
|
|
struct idr_layer *ary[1<<IDR_BITS];
|
|
int count; /* When zero, we can release it */
|
|
};
|
|
|
|
struct idr {
|
|
struct idr_layer *top;
|
|
struct idr_layer *id_free;
|
|
int layers;
|
|
int id_free_cnt;
|
|
spinlock_t lock;
|
|
};
|
|
|
|
#define IDR_INIT(name) \
|
|
{ \
|
|
.top = NULL, \
|
|
.id_free = NULL, \
|
|
.layers = 0, \
|
|
.id_free_cnt = 0, \
|
|
.lock = __SPIN_LOCK_UNLOCKED(name.lock), \
|
|
}
|
|
#define DEFINE_IDR(name) struct idr name = IDR_INIT(name)
|
|
|
|
/*
|
|
* This is what we export.
|
|
*/
|
|
|
|
void *idr_find(struct idr *idp, int id);
|
|
int idr_pre_get(struct idr *idp, gfp_t gfp_mask);
|
|
int idr_get_new(struct idr *idp, void *ptr, int *id);
|
|
int idr_get_new_above(struct idr *idp, void *ptr, int starting_id, int *id);
|
|
int idr_for_each(struct idr *idp,
|
|
int (*fn)(int id, void *p, void *data), void *data);
|
|
void *idr_replace(struct idr *idp, void *ptr, int id);
|
|
void idr_remove(struct idr *idp, int id);
|
|
void idr_destroy(struct idr *idp);
|
|
void idr_init(struct idr *idp);
|
|
|
|
|
|
/*
|
|
* IDA - IDR based id allocator, use when translation from id to
|
|
* pointer isn't necessary.
|
|
*/
|
|
#define IDA_CHUNK_SIZE 128 /* 128 bytes per chunk */
|
|
#define IDA_BITMAP_LONGS (128 / sizeof(long) - 1)
|
|
#define IDA_BITMAP_BITS (IDA_BITMAP_LONGS * sizeof(long) * 8)
|
|
|
|
struct ida_bitmap {
|
|
long nr_busy;
|
|
unsigned long bitmap[IDA_BITMAP_LONGS];
|
|
};
|
|
|
|
struct ida {
|
|
struct idr idr;
|
|
struct ida_bitmap *free_bitmap;
|
|
};
|
|
|
|
#define IDA_INIT(name) { .idr = IDR_INIT(name), .free_bitmap = NULL, }
|
|
#define DEFINE_IDA(name) struct ida name = IDA_INIT(name)
|
|
|
|
int ida_pre_get(struct ida *ida, gfp_t gfp_mask);
|
|
int ida_get_new_above(struct ida *ida, int starting_id, int *p_id);
|
|
int ida_get_new(struct ida *ida, int *p_id);
|
|
void ida_remove(struct ida *ida, int id);
|
|
void ida_destroy(struct ida *ida);
|
|
void ida_init(struct ida *ida);
|
|
|
|
#endif /* __IDR_H__ */
|