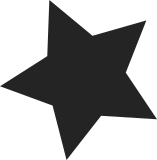
Both esp4 and esp6 used to assume that the SKB payload is encrypted and therefore the inner_network and inner_transport offsets are not relevant. When doing crypto offload in the NIC, this is no longer the case and the NIC driver needs these offsets so it can do TX TCP checksum offloading. This patch sets the inner_network and inner_transport members of the SKB, as well as encapsulation, to reflect the actual positions of these headers, and removes them only once encryption is done on the payload. Signed-off-by: Ilan Tayari <ilant@mellanox.com> Signed-off-by: Steffen Klassert <steffen.klassert@secunet.com>
117 lines
3.1 KiB
C
117 lines
3.1 KiB
C
/*
|
|
* xfrm4_mode_transport.c - Transport mode encapsulation for IPv4.
|
|
*
|
|
* Copyright (c) 2004-2006 Herbert Xu <herbert@gondor.apana.org.au>
|
|
*/
|
|
|
|
#include <linux/init.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/module.h>
|
|
#include <linux/skbuff.h>
|
|
#include <linux/stringify.h>
|
|
#include <net/dst.h>
|
|
#include <net/ip.h>
|
|
#include <net/xfrm.h>
|
|
#include <net/protocol.h>
|
|
|
|
/* Add encapsulation header.
|
|
*
|
|
* The IP header will be moved forward to make space for the encapsulation
|
|
* header.
|
|
*/
|
|
static int xfrm4_transport_output(struct xfrm_state *x, struct sk_buff *skb)
|
|
{
|
|
struct iphdr *iph = ip_hdr(skb);
|
|
int ihl = iph->ihl * 4;
|
|
|
|
skb_set_inner_transport_header(skb, skb_transport_offset(skb));
|
|
|
|
skb_set_network_header(skb, -x->props.header_len);
|
|
skb->mac_header = skb->network_header +
|
|
offsetof(struct iphdr, protocol);
|
|
skb->transport_header = skb->network_header + ihl;
|
|
__skb_pull(skb, ihl);
|
|
memmove(skb_network_header(skb), iph, ihl);
|
|
return 0;
|
|
}
|
|
|
|
/* Remove encapsulation header.
|
|
*
|
|
* The IP header will be moved over the top of the encapsulation header.
|
|
*
|
|
* On entry, skb->h shall point to where the IP header should be and skb->nh
|
|
* shall be set to where the IP header currently is. skb->data shall point
|
|
* to the start of the payload.
|
|
*/
|
|
static int xfrm4_transport_input(struct xfrm_state *x, struct sk_buff *skb)
|
|
{
|
|
int ihl = skb->data - skb_transport_header(skb);
|
|
struct xfrm_offload *xo = xfrm_offload(skb);
|
|
|
|
if (skb->transport_header != skb->network_header) {
|
|
memmove(skb_transport_header(skb),
|
|
skb_network_header(skb), ihl);
|
|
skb->network_header = skb->transport_header;
|
|
}
|
|
ip_hdr(skb)->tot_len = htons(skb->len + ihl);
|
|
if (!xo || !(xo->flags & XFRM_GRO))
|
|
skb_reset_transport_header(skb);
|
|
return 0;
|
|
}
|
|
|
|
static struct sk_buff *xfrm4_transport_gso_segment(struct xfrm_state *x,
|
|
struct sk_buff *skb,
|
|
netdev_features_t features)
|
|
{
|
|
const struct net_offload *ops;
|
|
struct sk_buff *segs = ERR_PTR(-EINVAL);
|
|
struct xfrm_offload *xo = xfrm_offload(skb);
|
|
|
|
skb->transport_header += x->props.header_len;
|
|
ops = rcu_dereference(inet_offloads[xo->proto]);
|
|
if (likely(ops && ops->callbacks.gso_segment))
|
|
segs = ops->callbacks.gso_segment(skb, features);
|
|
|
|
return segs;
|
|
}
|
|
|
|
static void xfrm4_transport_xmit(struct xfrm_state *x, struct sk_buff *skb)
|
|
{
|
|
struct xfrm_offload *xo = xfrm_offload(skb);
|
|
|
|
skb_reset_mac_len(skb);
|
|
pskb_pull(skb, skb->mac_len + sizeof(struct iphdr) + x->props.header_len);
|
|
|
|
if (xo->flags & XFRM_GSO_SEGMENT) {
|
|
skb_reset_transport_header(skb);
|
|
skb->transport_header -= x->props.header_len;
|
|
}
|
|
}
|
|
|
|
static struct xfrm_mode xfrm4_transport_mode = {
|
|
.input = xfrm4_transport_input,
|
|
.output = xfrm4_transport_output,
|
|
.gso_segment = xfrm4_transport_gso_segment,
|
|
.xmit = xfrm4_transport_xmit,
|
|
.owner = THIS_MODULE,
|
|
.encap = XFRM_MODE_TRANSPORT,
|
|
};
|
|
|
|
static int __init xfrm4_transport_init(void)
|
|
{
|
|
return xfrm_register_mode(&xfrm4_transport_mode, AF_INET);
|
|
}
|
|
|
|
static void __exit xfrm4_transport_exit(void)
|
|
{
|
|
int err;
|
|
|
|
err = xfrm_unregister_mode(&xfrm4_transport_mode, AF_INET);
|
|
BUG_ON(err);
|
|
}
|
|
|
|
module_init(xfrm4_transport_init);
|
|
module_exit(xfrm4_transport_exit);
|
|
MODULE_LICENSE("GPL");
|
|
MODULE_ALIAS_XFRM_MODE(AF_INET, XFRM_MODE_TRANSPORT);
|