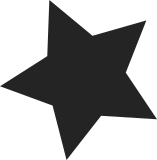
* Enable anatop, well bisa and RBC for suspend to optimize the power consumption a little bit * Clock changes for TVE, LDB, PATA, SRTC support * Add System Reset Controller (SRC) support for imx5 and imx6 * Add initial imx6dl support based on imx6q code * Kconfig for cpufreq-cpu0, defconfig updates and few other changes -----BEGIN PGP SIGNATURE----- Version: GnuPG v1.4.11 (GNU/Linux) iQEcBAABAgAGBQJRZ/viAAoJEFBXWFqHsHzOyqUH/2t7CCwlfTVUJYDCeo6PaE9x 41cV5zG6RvX1OfkRUmJ2N2klGn4zhwg6GHsCDQmvs+IODs4E7JeB9M92FAaPng71 NnuuwCQ01iIoaTtkz8z/n3tSet3fYB+xfNCMJoWIyS0edFFkCjOgnqRsA0pHRIOp G6ey1kU80D0f4+DjAUg1mkIvJrZxbRKDwmwqfDGJzQ4VU7cv70n027YuMKbeMyCC zdeKmpKSEl9AY+O/zeMrf03zEW+kdZ4eB6cTUFlpzYwPYY9o+XHx0U0535F7/x4+ KObUZ9Qg7liP5WO3ZzkVff5HJqPs6s/q99eOsU4/okF1x0fpq2mSgIHlSw4HpK8= =TuTx -----END PGP SIGNATURE----- Merge tag 'imx-soc-3.10' of git://git.linaro.org/people/shawnguo/linux-2.6 into next/soc2 From Shawn Guo: The imx soc changes for 3.10: * Enable anatop, well bisa and RBC for suspend to optimize the power consumption a little bit * Clock changes for TVE, LDB, PATA, SRTC support * Add System Reset Controller (SRC) support for imx5 and imx6 * Add initial imx6dl support based on imx6q code * Kconfig for cpufreq-cpu0, defconfig updates and few other changes * tag 'imx-soc-3.10' of git://git.linaro.org/people/shawnguo/linux-2.6: (275 commits) ARM i.MX53: set CLK_SET_RATE_PARENT flag on the tve_ext_sel clock ARM i.MX53: tve_di clock is not part of the CCM, but of TVE ARM i.MX53: make tve_ext_sel propagate rate change to PLL ARM i.MX53: Remove unused tve_gate clkdev entry ARM i.MX5: Remove tve_sel clock from i.MX53 clock tree ARM: i.MX5: Add PATA and SRTC clocks ARM: imx: do not bring up unavailable cores ARM: imx: add initial imx6dl support ARM: imx1: mm: add call to mxc_device_init ARM: imx_v4_v5_defconfig: Add CONFIG_GPIO_SYSFS ARM: imx_v6_v7_defconfig: Select CONFIG_PERF_EVENTS ARM: i.MX53 Add the cko1, cko2 clock outputs. staging: drm/imx: Use SRC to reset IPU ARM i.MX6q: Add GPU, VPU, IPU, and OpenVG resets to System Reset Controller (SRC) ARM: imx: do not use regmap_read for ANADIG_DIGPROG ARM i.MX6q: set the LDB serial clock parent to the video PLL ARM i.MX6q: Add audio/video PLL post dividers for i.MX6q rev 1.1 ARM i.MX6q: fix ldb di divider and selector clocks ARM i.MX53: fix ldb di divider and selector clocks ARM i.MX: Add imx_clk_divider_flags and imx_clk_mux_flags ... Signed-off-by: Olof Johansson <olof@lixom.net> Trivial change/change conflict in arch/arm/mach-imx/mach-imx6q.c resolved.
94 lines
2 KiB
C
94 lines
2 KiB
C
/*
|
|
* Copyright 2011 Freescale Semiconductor, Inc.
|
|
* Copyright 2011 Linaro Ltd.
|
|
*
|
|
* The code contained herein is licensed under the GNU General Public
|
|
* License. You may obtain a copy of the GNU General Public License
|
|
* Version 2 or later at the following locations:
|
|
*
|
|
* http://www.opensource.org/licenses/gpl-license.html
|
|
* http://www.gnu.org/copyleft/gpl.html
|
|
*/
|
|
|
|
#include <linux/init.h>
|
|
#include <linux/smp.h>
|
|
#include <asm/page.h>
|
|
#include <asm/smp_scu.h>
|
|
#include <asm/mach/map.h>
|
|
|
|
#include "common.h"
|
|
#include "hardware.h"
|
|
|
|
#define SCU_STANDBY_ENABLE (1 << 5)
|
|
|
|
static void __iomem *scu_base;
|
|
|
|
static struct map_desc scu_io_desc __initdata = {
|
|
/* .virtual and .pfn are run-time assigned */
|
|
.length = SZ_4K,
|
|
.type = MT_DEVICE,
|
|
};
|
|
|
|
void __init imx_scu_map_io(void)
|
|
{
|
|
unsigned long base;
|
|
|
|
/* Get SCU base */
|
|
asm("mrc p15, 4, %0, c15, c0, 0" : "=r" (base));
|
|
|
|
scu_io_desc.virtual = IMX_IO_P2V(base);
|
|
scu_io_desc.pfn = __phys_to_pfn(base);
|
|
iotable_init(&scu_io_desc, 1);
|
|
|
|
scu_base = IMX_IO_ADDRESS(base);
|
|
}
|
|
|
|
void imx_scu_standby_enable(void)
|
|
{
|
|
u32 val = readl_relaxed(scu_base);
|
|
|
|
val |= SCU_STANDBY_ENABLE;
|
|
writel_relaxed(val, scu_base);
|
|
}
|
|
|
|
static int __cpuinit imx_boot_secondary(unsigned int cpu, struct task_struct *idle)
|
|
{
|
|
imx_set_cpu_jump(cpu, v7_secondary_startup);
|
|
imx_enable_cpu(cpu, true);
|
|
return 0;
|
|
}
|
|
|
|
/*
|
|
* Initialise the CPU possible map early - this describes the CPUs
|
|
* which may be present or become present in the system.
|
|
*/
|
|
static void __init imx_smp_init_cpus(void)
|
|
{
|
|
int i, ncores;
|
|
|
|
ncores = scu_get_core_count(scu_base);
|
|
|
|
for (i = ncores; i < NR_CPUS; i++)
|
|
set_cpu_possible(i, false);
|
|
}
|
|
|
|
void imx_smp_prepare(void)
|
|
{
|
|
scu_enable(scu_base);
|
|
}
|
|
|
|
static void __init imx_smp_prepare_cpus(unsigned int max_cpus)
|
|
{
|
|
imx_smp_prepare();
|
|
}
|
|
|
|
struct smp_operations imx_smp_ops __initdata = {
|
|
.smp_init_cpus = imx_smp_init_cpus,
|
|
.smp_prepare_cpus = imx_smp_prepare_cpus,
|
|
.smp_boot_secondary = imx_boot_secondary,
|
|
#ifdef CONFIG_HOTPLUG_CPU
|
|
.cpu_die = imx_cpu_die,
|
|
.cpu_kill = imx_cpu_kill,
|
|
#endif
|
|
};
|