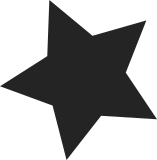
Initialize the callchain radix tree root correctly. When we walk through the parents, we must stop after the root, but since it wasn't well initialized, its parent pointer was random. Also the number of hits was random because uninitialized, hence it was part of the callchain while the root doesn't contain anything. This fixes segfaults and percentages followed by empty callchains while running: perf report -g flat Reported-by: Ingo Molnar <mingo@elte.hu> Signed-off-by: Frederic Weisbecker <fweisbec@gmail.com> Cc: Peter Zijlstra <a.p.zijlstra@chello.nl> Cc: Arnaldo Carvalho de Melo <acme@redhat.com> Cc: Paul Mackerras <paulus@samba.org> Cc: 2.6.31.x-2.6.34.x <stable@kernel.org>
68 lines
1.4 KiB
C
68 lines
1.4 KiB
C
#ifndef __PERF_CALLCHAIN_H
|
|
#define __PERF_CALLCHAIN_H
|
|
|
|
#include "../perf.h"
|
|
#include <linux/list.h>
|
|
#include <linux/rbtree.h>
|
|
#include "event.h"
|
|
#include "symbol.h"
|
|
|
|
enum chain_mode {
|
|
CHAIN_NONE,
|
|
CHAIN_FLAT,
|
|
CHAIN_GRAPH_ABS,
|
|
CHAIN_GRAPH_REL
|
|
};
|
|
|
|
struct callchain_node {
|
|
struct callchain_node *parent;
|
|
struct list_head brothers;
|
|
struct list_head children;
|
|
struct list_head val;
|
|
struct rb_node rb_node; /* to sort nodes in an rbtree */
|
|
struct rb_root rb_root; /* sorted tree of children */
|
|
unsigned int val_nr;
|
|
u64 hit;
|
|
u64 children_hit;
|
|
};
|
|
|
|
struct callchain_param;
|
|
|
|
typedef void (*sort_chain_func_t)(struct rb_root *, struct callchain_node *,
|
|
u64, struct callchain_param *);
|
|
|
|
struct callchain_param {
|
|
enum chain_mode mode;
|
|
u32 print_limit;
|
|
double min_percent;
|
|
sort_chain_func_t sort;
|
|
};
|
|
|
|
struct callchain_list {
|
|
u64 ip;
|
|
struct map_symbol ms;
|
|
struct list_head list;
|
|
};
|
|
|
|
static inline void callchain_init(struct callchain_node *node)
|
|
{
|
|
INIT_LIST_HEAD(&node->brothers);
|
|
INIT_LIST_HEAD(&node->children);
|
|
INIT_LIST_HEAD(&node->val);
|
|
|
|
node->parent = NULL;
|
|
node->hit = 0;
|
|
}
|
|
|
|
static inline u64 cumul_hits(struct callchain_node *node)
|
|
{
|
|
return node->hit + node->children_hit;
|
|
}
|
|
|
|
int register_callchain_param(struct callchain_param *param);
|
|
int append_chain(struct callchain_node *root, struct ip_callchain *chain,
|
|
struct map_symbol *syms);
|
|
|
|
bool ip_callchain__valid(struct ip_callchain *chain, event_t *event);
|
|
#endif /* __PERF_CALLCHAIN_H */
|