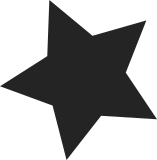
Michael Leun reported that running parallel opens on a fuse filesystem can trigger a "kernel BUG at mm/truncate.c:475" Gurudas Pai reported the same bug on NFS. The reason is, unmap_mapping_range() is not prepared for more than one concurrent invocation per inode. For example: thread1: going through a big range, stops in the middle of a vma and stores the restart address in vm_truncate_count. thread2: comes in with a small (e.g. single page) unmap request on the same vma, somewhere before restart_address, finds that the vma was already unmapped up to the restart address and happily returns without doing anything. Another scenario would be two big unmap requests, both having to restart the unmapping and each one setting vm_truncate_count to its own value. This could go on forever without any of them being able to finish. Truncate and hole punching already serialize with i_mutex. Other callers of unmap_mapping_range() do not, and it's difficult to get i_mutex protection for all callers. In particular ->d_revalidate(), which calls invalidate_inode_pages2_range() in fuse, may be called with or without i_mutex. This patch adds a new mutex to 'struct address_space' to prevent running multiple concurrent unmap_mapping_range() on the same mapping. [ We'll hopefully get rid of all this with the upcoming mm preemptibility series by Peter Zijlstra, the "mm: Remove i_mmap_mutex lockbreak" patch in particular. But that is for 2.6.39 ] Signed-off-by: Miklos Szeredi <mszeredi@suse.cz> Reported-by: Michael Leun <lkml20101129@newton.leun.net> Reported-by: Gurudas Pai <gurudas.pai@oracle.com> Tested-by: Gurudas Pai <gurudas.pai@oracle.com> Acked-by: Hugh Dickins <hughd@google.com> Cc: stable@kernel.org Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
58 lines
2 KiB
C
58 lines
2 KiB
C
/*
|
|
* btnode.h - NILFS B-tree node cache
|
|
*
|
|
* Copyright (C) 2005-2008 Nippon Telegraph and Telephone Corporation.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
|
|
*
|
|
* Written by Seiji Kihara <kihara@osrg.net>
|
|
* Revised by Ryusuke Konishi <ryusuke@osrg.net>
|
|
*/
|
|
|
|
#ifndef _NILFS_BTNODE_H
|
|
#define _NILFS_BTNODE_H
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/buffer_head.h>
|
|
#include <linux/fs.h>
|
|
#include <linux/backing-dev.h>
|
|
|
|
|
|
struct nilfs_btnode_chkey_ctxt {
|
|
__u64 oldkey;
|
|
__u64 newkey;
|
|
struct buffer_head *bh;
|
|
struct buffer_head *newbh;
|
|
};
|
|
|
|
void nilfs_btnode_cache_init(struct address_space *, struct backing_dev_info *);
|
|
void nilfs_btnode_cache_clear(struct address_space *);
|
|
struct buffer_head *nilfs_btnode_create_block(struct address_space *btnc,
|
|
__u64 blocknr);
|
|
int nilfs_btnode_submit_block(struct address_space *, __u64, sector_t, int,
|
|
struct buffer_head **, sector_t *);
|
|
void nilfs_btnode_delete(struct buffer_head *);
|
|
int nilfs_btnode_prepare_change_key(struct address_space *,
|
|
struct nilfs_btnode_chkey_ctxt *);
|
|
void nilfs_btnode_commit_change_key(struct address_space *,
|
|
struct nilfs_btnode_chkey_ctxt *);
|
|
void nilfs_btnode_abort_change_key(struct address_space *,
|
|
struct nilfs_btnode_chkey_ctxt *);
|
|
|
|
#define nilfs_btnode_mark_dirty(bh) nilfs_mark_buffer_dirty(bh)
|
|
|
|
|
|
#endif /* _NILFS_BTNODE_H */
|