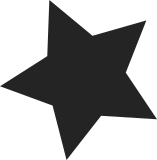
When a client authenticates with a service, an authorizer is sent with a nonce to the service (ceph_x_authorize_[ab]) and the service responds with a mutation of that nonce (ceph_x_authorize_reply). This lets the client verify the service is who it says it is but it doesn't protect against a replay: someone can trivially capture the exchange and reuse the same authorizer to authenticate themselves. Allow the service to reject an initial authorizer with a random challenge (ceph_x_authorize_challenge). The client then has to respond with an updated authorizer proving they are able to decrypt the service's challenge and that the new authorizer was produced for this specific connection instance. The accepting side requires this challenge and response unconditionally if the client side advertises they have CEPHX_V2 feature bit. This addresses CVE-2018-1128. Link: http://tracker.ceph.com/issues/24836 Signed-off-by: Ilya Dryomov <idryomov@gmail.com> Reviewed-by: Sage Weil <sage@redhat.com>
99 lines
1.9 KiB
C
99 lines
1.9 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef __FS_CEPH_AUTH_X_PROTOCOL
|
|
#define __FS_CEPH_AUTH_X_PROTOCOL
|
|
|
|
#define CEPHX_GET_AUTH_SESSION_KEY 0x0100
|
|
#define CEPHX_GET_PRINCIPAL_SESSION_KEY 0x0200
|
|
#define CEPHX_GET_ROTATING_KEY 0x0400
|
|
|
|
/* common bits */
|
|
struct ceph_x_ticket_blob {
|
|
__u8 struct_v;
|
|
__le64 secret_id;
|
|
__le32 blob_len;
|
|
char blob[];
|
|
} __attribute__ ((packed));
|
|
|
|
|
|
/* common request/reply headers */
|
|
struct ceph_x_request_header {
|
|
__le16 op;
|
|
} __attribute__ ((packed));
|
|
|
|
struct ceph_x_reply_header {
|
|
__le16 op;
|
|
__le32 result;
|
|
} __attribute__ ((packed));
|
|
|
|
|
|
/* authenticate handshake */
|
|
|
|
/* initial hello (no reply header) */
|
|
struct ceph_x_server_challenge {
|
|
__u8 struct_v;
|
|
__le64 server_challenge;
|
|
} __attribute__ ((packed));
|
|
|
|
struct ceph_x_authenticate {
|
|
__u8 struct_v;
|
|
__le64 client_challenge;
|
|
__le64 key;
|
|
/* ticket blob */
|
|
} __attribute__ ((packed));
|
|
|
|
struct ceph_x_service_ticket_request {
|
|
__u8 struct_v;
|
|
__le32 keys;
|
|
} __attribute__ ((packed));
|
|
|
|
struct ceph_x_challenge_blob {
|
|
__le64 server_challenge;
|
|
__le64 client_challenge;
|
|
} __attribute__ ((packed));
|
|
|
|
|
|
|
|
/* authorize handshake */
|
|
|
|
/*
|
|
* The authorizer consists of two pieces:
|
|
* a - service id, ticket blob
|
|
* b - encrypted with session key
|
|
*/
|
|
struct ceph_x_authorize_a {
|
|
__u8 struct_v;
|
|
__le64 global_id;
|
|
__le32 service_id;
|
|
struct ceph_x_ticket_blob ticket_blob;
|
|
} __attribute__ ((packed));
|
|
|
|
struct ceph_x_authorize_b {
|
|
__u8 struct_v;
|
|
__le64 nonce;
|
|
__u8 have_challenge;
|
|
__le64 server_challenge_plus_one;
|
|
} __attribute__ ((packed));
|
|
|
|
struct ceph_x_authorize_challenge {
|
|
__u8 struct_v;
|
|
__le64 server_challenge;
|
|
} __attribute__ ((packed));
|
|
|
|
struct ceph_x_authorize_reply {
|
|
__u8 struct_v;
|
|
__le64 nonce_plus_one;
|
|
} __attribute__ ((packed));
|
|
|
|
|
|
/*
|
|
* encyption bundle
|
|
*/
|
|
#define CEPHX_ENC_MAGIC 0xff009cad8826aa55ull
|
|
|
|
struct ceph_x_encrypt_header {
|
|
__u8 struct_v;
|
|
__le64 magic;
|
|
} __attribute__ ((packed));
|
|
|
|
#endif
|