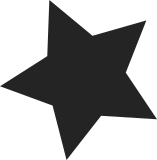
Use the new typedef for interrupt handler function pointers rather than actually spelling out the full thing each time. This was scripted with the following small shell script: #!/bin/sh egrep -nHrl -e 'irqreturn_t[ ]*[(][*]' $* | while read i do echo $i perl -pi -e 's/irqreturn_t\s*[(]\s*[*]\s*([_a-zA-Z0-9]*)\s*[)]\s*[(]\s*int\s*,\s*void\s*[*]\s*[)]/irq_handler_t \1/g' $i || exit $? done Signed-Off-By: David Howells <dhowells@redhat.com>
104 lines
2.1 KiB
C
104 lines
2.1 KiB
C
/*
|
|
* linux/arch/m68k/sun3x/time.c
|
|
*
|
|
* Sun3x-specific time handling
|
|
*/
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/kd.h>
|
|
#include <linux/init.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/kernel_stat.h>
|
|
#include <linux/interrupt.h>
|
|
#include <linux/rtc.h>
|
|
#include <linux/bcd.h>
|
|
|
|
#include <asm/irq.h>
|
|
#include <asm/io.h>
|
|
#include <asm/system.h>
|
|
#include <asm/traps.h>
|
|
#include <asm/sun3x.h>
|
|
#include <asm/sun3ints.h>
|
|
#include <asm/rtc.h>
|
|
|
|
#include "time.h"
|
|
|
|
#define M_CONTROL 0xf8
|
|
#define M_SEC 0xf9
|
|
#define M_MIN 0xfa
|
|
#define M_HOUR 0xfb
|
|
#define M_DAY 0xfc
|
|
#define M_DATE 0xfd
|
|
#define M_MONTH 0xfe
|
|
#define M_YEAR 0xff
|
|
|
|
#define C_WRITE 0x80
|
|
#define C_READ 0x40
|
|
#define C_SIGN 0x20
|
|
#define C_CALIB 0x1f
|
|
|
|
int sun3x_hwclk(int set, struct rtc_time *t)
|
|
{
|
|
volatile struct mostek_dt *h =
|
|
(struct mostek_dt *)(SUN3X_EEPROM+M_CONTROL);
|
|
unsigned long flags;
|
|
|
|
local_irq_save(flags);
|
|
|
|
if(set) {
|
|
h->csr |= C_WRITE;
|
|
h->sec = BIN2BCD(t->tm_sec);
|
|
h->min = BIN2BCD(t->tm_min);
|
|
h->hour = BIN2BCD(t->tm_hour);
|
|
h->wday = BIN2BCD(t->tm_wday);
|
|
h->mday = BIN2BCD(t->tm_mday);
|
|
h->month = BIN2BCD(t->tm_mon);
|
|
h->year = BIN2BCD(t->tm_year);
|
|
h->csr &= ~C_WRITE;
|
|
} else {
|
|
h->csr |= C_READ;
|
|
t->tm_sec = BCD2BIN(h->sec);
|
|
t->tm_min = BCD2BIN(h->min);
|
|
t->tm_hour = BCD2BIN(h->hour);
|
|
t->tm_wday = BCD2BIN(h->wday);
|
|
t->tm_mday = BCD2BIN(h->mday);
|
|
t->tm_mon = BCD2BIN(h->month);
|
|
t->tm_year = BCD2BIN(h->year);
|
|
h->csr &= ~C_READ;
|
|
}
|
|
|
|
local_irq_restore(flags);
|
|
|
|
return 0;
|
|
}
|
|
/* Not much we can do here */
|
|
unsigned long sun3x_gettimeoffset (void)
|
|
{
|
|
return 0L;
|
|
}
|
|
|
|
#if 0
|
|
static void sun3x_timer_tick(int irq, void *dev_id, struct pt_regs *regs)
|
|
{
|
|
void (*vector)(int, void *, struct pt_regs *) = dev_id;
|
|
|
|
/* Clear the pending interrupt - pulse the enable line low */
|
|
disable_irq(5);
|
|
enable_irq(5);
|
|
|
|
vector(irq, NULL, regs);
|
|
}
|
|
#endif
|
|
|
|
void __init sun3x_sched_init(irq_handler_t vector)
|
|
{
|
|
|
|
sun3_disable_interrupts();
|
|
|
|
|
|
/* Pulse enable low to get the clock started */
|
|
sun3_disable_irq(5);
|
|
sun3_enable_irq(5);
|
|
sun3_enable_interrupts();
|
|
}
|