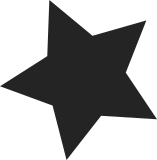
The iomux function declarations are in headers only accessible in this directory. Thus those can't be used in any module. None of the objects in this directory is tristate. Neither can the header be included in out-of-tree modules. Signed-off-by: Alexander Stein <alexander.stein@systec-electronic.com> Signed-off-by: Shawn Guo <shawn.guo@freescale.com>
78 lines
2.3 KiB
C
78 lines
2.3 KiB
C
/*
|
|
* Copyright 2004-2006 Freescale Semiconductor, Inc. All Rights Reserved.
|
|
* Copyright (C) 2008 by Sascha Hauer <kernel@pengutronix.de>
|
|
* Copyright (C) 2009 by Jan Weitzel Phytec Messtechnik GmbH,
|
|
* <armlinux@phytec.de>
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston,
|
|
* MA 02110-1301, USA.
|
|
*/
|
|
#include <linux/errno.h>
|
|
#include <linux/init.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/module.h>
|
|
#include <linux/string.h>
|
|
#include <linux/gpio.h>
|
|
|
|
#include <asm/mach/map.h>
|
|
|
|
#include "hardware.h"
|
|
#include "iomux-v3.h"
|
|
|
|
static void __iomem *base;
|
|
|
|
/*
|
|
* configures a single pad in the iomuxer
|
|
*/
|
|
int mxc_iomux_v3_setup_pad(iomux_v3_cfg_t pad)
|
|
{
|
|
u32 mux_ctrl_ofs = (pad & MUX_CTRL_OFS_MASK) >> MUX_CTRL_OFS_SHIFT;
|
|
u32 mux_mode = (pad & MUX_MODE_MASK) >> MUX_MODE_SHIFT;
|
|
u32 sel_input_ofs = (pad & MUX_SEL_INPUT_OFS_MASK) >> MUX_SEL_INPUT_OFS_SHIFT;
|
|
u32 sel_input = (pad & MUX_SEL_INPUT_MASK) >> MUX_SEL_INPUT_SHIFT;
|
|
u32 pad_ctrl_ofs = (pad & MUX_PAD_CTRL_OFS_MASK) >> MUX_PAD_CTRL_OFS_SHIFT;
|
|
u32 pad_ctrl = (pad & MUX_PAD_CTRL_MASK) >> MUX_PAD_CTRL_SHIFT;
|
|
|
|
if (mux_ctrl_ofs)
|
|
__raw_writel(mux_mode, base + mux_ctrl_ofs);
|
|
|
|
if (sel_input_ofs)
|
|
__raw_writel(sel_input, base + sel_input_ofs);
|
|
|
|
if (!(pad_ctrl & NO_PAD_CTRL) && pad_ctrl_ofs)
|
|
__raw_writel(pad_ctrl, base + pad_ctrl_ofs);
|
|
|
|
return 0;
|
|
}
|
|
|
|
int mxc_iomux_v3_setup_multiple_pads(iomux_v3_cfg_t *pad_list, unsigned count)
|
|
{
|
|
iomux_v3_cfg_t *p = pad_list;
|
|
int i;
|
|
int ret;
|
|
|
|
for (i = 0; i < count; i++) {
|
|
ret = mxc_iomux_v3_setup_pad(*p);
|
|
if (ret)
|
|
return ret;
|
|
p++;
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
void mxc_iomux_v3_init(void __iomem *iomux_v3_base)
|
|
{
|
|
base = iomux_v3_base;
|
|
}
|