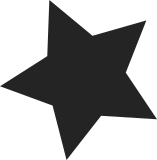
As discussed at Linux Plumbers Conference 2018 in Vancouver [1] this is the implementation of binderfs. /* Abstract */ binderfs is a backwards-compatible filesystem for Android's binder ipc mechanism. Each ipc namespace will mount a new binderfs instance. Mounting binderfs multiple times at different locations in the same ipc namespace will not cause a new super block to be allocated and hence it will be the same filesystem instance. Each new binderfs mount will have its own set of binder devices only visible in the ipc namespace it has been mounted in. All devices in a new binderfs mount will follow the scheme binder%d and numbering will always start at 0. /* Backwards compatibility */ Devices requested in the Kconfig via CONFIG_ANDROID_BINDER_DEVICES for the initial ipc namespace will work as before. They will be registered via misc_register() and appear in the devtmpfs mount. Specifically, the standard devices binder, hwbinder, and vndbinder will all appear in their standard locations in /dev. Mounting or unmounting the binderfs mount in the initial ipc namespace will have no effect on these devices, i.e. they will neither show up in the binderfs mount nor will they disappear when the binderfs mount is gone. /* binder-control */ Each new binderfs instance comes with a binder-control device. No other devices will be present at first. The binder-control device can be used to dynamically allocate binder devices. All requests operate on the binderfs mount the binder-control device resides in. Assuming a new instance of binderfs has been mounted at /dev/binderfs via mount -t binderfs binderfs /dev/binderfs. Then a request to create a new binder device can be made as illustrated in [2]. Binderfs devices can simply be removed via unlink(). /* Implementation details */ - dynamic major number allocation: When binderfs is registered as a new filesystem it will dynamically allocate a new major number. The allocated major number will be returned in struct binderfs_device when a new binder device is allocated. - global minor number tracking: Minor are tracked in a global idr struct that is capped at BINDERFS_MAX_MINOR. The minor number tracker is protected by a global mutex. This is the only point of contention between binderfs mounts. - struct binderfs_info: Each binderfs super block has its own struct binderfs_info that tracks specific details about a binderfs instance: - ipc namespace - dentry of the binder-control device - root uid and root gid of the user namespace the binderfs instance was mounted in - mountable by user namespace root: binderfs can be mounted by user namespace root in a non-initial user namespace. The devices will be owned by user namespace root. - binderfs binder devices without misc infrastructure: New binder devices associated with a binderfs mount do not use the full misc_register() infrastructure. The misc_register() infrastructure can only create new devices in the host's devtmpfs mount. binderfs does however only make devices appear under its own mountpoint and thus allocates new character device nodes from the inode of the root dentry of the super block. This will have the side-effect that binderfs specific device nodes do not appear in sysfs. This behavior is similar to devpts allocated pts devices and has no effect on the functionality of the ipc mechanism itself. [1]: https://goo.gl/JL2tfX [2]: program to allocate a new binderfs binder device: #define _GNU_SOURCE #include <errno.h> #include <fcntl.h> #include <stdio.h> #include <stdlib.h> #include <string.h> #include <sys/ioctl.h> #include <sys/stat.h> #include <sys/types.h> #include <unistd.h> #include <linux/android/binder_ctl.h> int main(int argc, char *argv[]) { int fd, ret, saved_errno; size_t len; struct binderfs_device device = { 0 }; if (argc < 2) exit(EXIT_FAILURE); len = strlen(argv[1]); if (len > BINDERFS_MAX_NAME) exit(EXIT_FAILURE); memcpy(device.name, argv[1], len); fd = open("/dev/binderfs/binder-control", O_RDONLY | O_CLOEXEC); if (fd < 0) { printf("%s - Failed to open binder-control device\n", strerror(errno)); exit(EXIT_FAILURE); } ret = ioctl(fd, BINDER_CTL_ADD, &device); saved_errno = errno; close(fd); errno = saved_errno; if (ret < 0) { printf("%s - Failed to allocate new binder device\n", strerror(errno)); exit(EXIT_FAILURE); } printf("Allocated new binder device with major %d, minor %d, and " "name %s\n", device.major, device.minor, device.name); exit(EXIT_SUCCESS); } Cc: Martijn Coenen <maco@android.com> Cc: Greg Kroah-Hartman <gregkh@linuxfoundation.org> Signed-off-by: Christian Brauner <christian.brauner@ubuntu.com> Acked-by: Todd Kjos <tkjos@google.com> Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
96 lines
3.4 KiB
C
96 lines
3.4 KiB
C
/* SPDX-License-Identifier: GPL-2.0 WITH Linux-syscall-note */
|
|
#ifndef __LINUX_MAGIC_H__
|
|
#define __LINUX_MAGIC_H__
|
|
|
|
#define ADFS_SUPER_MAGIC 0xadf5
|
|
#define AFFS_SUPER_MAGIC 0xadff
|
|
#define AFS_SUPER_MAGIC 0x5346414F
|
|
#define AUTOFS_SUPER_MAGIC 0x0187
|
|
#define CODA_SUPER_MAGIC 0x73757245
|
|
#define CRAMFS_MAGIC 0x28cd3d45 /* some random number */
|
|
#define CRAMFS_MAGIC_WEND 0x453dcd28 /* magic number with the wrong endianess */
|
|
#define DEBUGFS_MAGIC 0x64626720
|
|
#define SECURITYFS_MAGIC 0x73636673
|
|
#define SELINUX_MAGIC 0xf97cff8c
|
|
#define SMACK_MAGIC 0x43415d53 /* "SMAC" */
|
|
#define RAMFS_MAGIC 0x858458f6 /* some random number */
|
|
#define TMPFS_MAGIC 0x01021994
|
|
#define HUGETLBFS_MAGIC 0x958458f6 /* some random number */
|
|
#define SQUASHFS_MAGIC 0x73717368
|
|
#define ECRYPTFS_SUPER_MAGIC 0xf15f
|
|
#define EFS_SUPER_MAGIC 0x414A53
|
|
#define EXT2_SUPER_MAGIC 0xEF53
|
|
#define EXT3_SUPER_MAGIC 0xEF53
|
|
#define XENFS_SUPER_MAGIC 0xabba1974
|
|
#define EXT4_SUPER_MAGIC 0xEF53
|
|
#define BTRFS_SUPER_MAGIC 0x9123683E
|
|
#define NILFS_SUPER_MAGIC 0x3434
|
|
#define F2FS_SUPER_MAGIC 0xF2F52010
|
|
#define HPFS_SUPER_MAGIC 0xf995e849
|
|
#define ISOFS_SUPER_MAGIC 0x9660
|
|
#define JFFS2_SUPER_MAGIC 0x72b6
|
|
#define XFS_SUPER_MAGIC 0x58465342 /* "XFSB" */
|
|
#define PSTOREFS_MAGIC 0x6165676C
|
|
#define EFIVARFS_MAGIC 0xde5e81e4
|
|
#define HOSTFS_SUPER_MAGIC 0x00c0ffee
|
|
#define OVERLAYFS_SUPER_MAGIC 0x794c7630
|
|
|
|
#define MINIX_SUPER_MAGIC 0x137F /* minix v1 fs, 14 char names */
|
|
#define MINIX_SUPER_MAGIC2 0x138F /* minix v1 fs, 30 char names */
|
|
#define MINIX2_SUPER_MAGIC 0x2468 /* minix v2 fs, 14 char names */
|
|
#define MINIX2_SUPER_MAGIC2 0x2478 /* minix v2 fs, 30 char names */
|
|
#define MINIX3_SUPER_MAGIC 0x4d5a /* minix v3 fs, 60 char names */
|
|
|
|
#define MSDOS_SUPER_MAGIC 0x4d44 /* MD */
|
|
#define NCP_SUPER_MAGIC 0x564c /* Guess, what 0x564c is :-) */
|
|
#define NFS_SUPER_MAGIC 0x6969
|
|
#define OCFS2_SUPER_MAGIC 0x7461636f
|
|
#define OPENPROM_SUPER_MAGIC 0x9fa1
|
|
#define QNX4_SUPER_MAGIC 0x002f /* qnx4 fs detection */
|
|
#define QNX6_SUPER_MAGIC 0x68191122 /* qnx6 fs detection */
|
|
#define AFS_FS_MAGIC 0x6B414653
|
|
|
|
#define REISERFS_SUPER_MAGIC 0x52654973 /* used by gcc */
|
|
/* used by file system utilities that
|
|
look at the superblock, etc. */
|
|
#define REISERFS_SUPER_MAGIC_STRING "ReIsErFs"
|
|
#define REISER2FS_SUPER_MAGIC_STRING "ReIsEr2Fs"
|
|
#define REISER2FS_JR_SUPER_MAGIC_STRING "ReIsEr3Fs"
|
|
|
|
#define SMB_SUPER_MAGIC 0x517B
|
|
#define CGROUP_SUPER_MAGIC 0x27e0eb
|
|
#define CGROUP2_SUPER_MAGIC 0x63677270
|
|
|
|
#define RDTGROUP_SUPER_MAGIC 0x7655821
|
|
|
|
#define STACK_END_MAGIC 0x57AC6E9D
|
|
|
|
#define TRACEFS_MAGIC 0x74726163
|
|
|
|
#define V9FS_MAGIC 0x01021997
|
|
|
|
#define BDEVFS_MAGIC 0x62646576
|
|
#define DAXFS_MAGIC 0x64646178
|
|
#define BINFMTFS_MAGIC 0x42494e4d
|
|
#define DEVPTS_SUPER_MAGIC 0x1cd1
|
|
#define BINDERFS_SUPER_MAGIC 0x6c6f6f70
|
|
#define FUTEXFS_SUPER_MAGIC 0xBAD1DEA
|
|
#define PIPEFS_MAGIC 0x50495045
|
|
#define PROC_SUPER_MAGIC 0x9fa0
|
|
#define SOCKFS_MAGIC 0x534F434B
|
|
#define SYSFS_MAGIC 0x62656572
|
|
#define USBDEVICE_SUPER_MAGIC 0x9fa2
|
|
#define MTD_INODE_FS_MAGIC 0x11307854
|
|
#define ANON_INODE_FS_MAGIC 0x09041934
|
|
#define BTRFS_TEST_MAGIC 0x73727279
|
|
#define NSFS_MAGIC 0x6e736673
|
|
#define BPF_FS_MAGIC 0xcafe4a11
|
|
#define AAFS_MAGIC 0x5a3c69f0
|
|
|
|
/* Since UDF 2.01 is ISO 13346 based... */
|
|
#define UDF_SUPER_MAGIC 0x15013346
|
|
#define BALLOON_KVM_MAGIC 0x13661366
|
|
#define ZSMALLOC_MAGIC 0x58295829
|
|
|
|
#endif /* __LINUX_MAGIC_H__ */
|