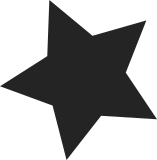
Pull vfs updates from Al Viro: "Assorted patches from Miklos. An interesting part here is /proc/mounts stuff..." The "/proc/mounts stuff" is using a cursor for keeeping the location data while traversing the mount listing. Also probably worth noting is the addition of faccessat2(), which takes an additional set of flags to specify how the lookup is done (AT_EACCESS, AT_SYMLINK_NOFOLLOW, AT_EMPTY_PATH). * 'from-miklos' of git://git.kernel.org/pub/scm/linux/kernel/git/viro/vfs: vfs: add faccessat2 syscall vfs: don't parse "silent" option vfs: don't parse "posixacl" option vfs: don't parse forbidden flags statx: add mount_root statx: add mount ID statx: don't clear STATX_ATIME on SB_RDONLY uapi: deprecate STATX_ALL utimensat: AT_EMPTY_PATH support vfs: split out access_override_creds() proc/mounts: add cursor aio: fix async fsync creds vfs: allow unprivileged whiteout creation
68 lines
1.6 KiB
C
68 lines
1.6 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#include <linux/fs.h>
|
|
|
|
#define DEVCG_ACC_MKNOD 1
|
|
#define DEVCG_ACC_READ 2
|
|
#define DEVCG_ACC_WRITE 4
|
|
#define DEVCG_ACC_MASK (DEVCG_ACC_MKNOD | DEVCG_ACC_READ | DEVCG_ACC_WRITE)
|
|
|
|
#define DEVCG_DEV_BLOCK 1
|
|
#define DEVCG_DEV_CHAR 2
|
|
#define DEVCG_DEV_ALL 4 /* this represents all devices */
|
|
|
|
|
|
#if defined(CONFIG_CGROUP_DEVICE) || defined(CONFIG_CGROUP_BPF)
|
|
int devcgroup_check_permission(short type, u32 major, u32 minor,
|
|
short access);
|
|
static inline int devcgroup_inode_permission(struct inode *inode, int mask)
|
|
{
|
|
short type, access = 0;
|
|
|
|
if (likely(!inode->i_rdev))
|
|
return 0;
|
|
|
|
if (S_ISBLK(inode->i_mode))
|
|
type = DEVCG_DEV_BLOCK;
|
|
else if (S_ISCHR(inode->i_mode))
|
|
type = DEVCG_DEV_CHAR;
|
|
else
|
|
return 0;
|
|
|
|
if (mask & MAY_WRITE)
|
|
access |= DEVCG_ACC_WRITE;
|
|
if (mask & MAY_READ)
|
|
access |= DEVCG_ACC_READ;
|
|
|
|
return devcgroup_check_permission(type, imajor(inode), iminor(inode),
|
|
access);
|
|
}
|
|
|
|
static inline int devcgroup_inode_mknod(int mode, dev_t dev)
|
|
{
|
|
short type;
|
|
|
|
if (!S_ISBLK(mode) && !S_ISCHR(mode))
|
|
return 0;
|
|
|
|
if (S_ISCHR(mode) && dev == WHITEOUT_DEV)
|
|
return 0;
|
|
|
|
if (S_ISBLK(mode))
|
|
type = DEVCG_DEV_BLOCK;
|
|
else
|
|
type = DEVCG_DEV_CHAR;
|
|
|
|
return devcgroup_check_permission(type, MAJOR(dev), MINOR(dev),
|
|
DEVCG_ACC_MKNOD);
|
|
}
|
|
|
|
#else
|
|
static inline int devcgroup_check_permission(short type, u32 major, u32 minor,
|
|
short access)
|
|
{ return 0; }
|
|
static inline int devcgroup_inode_permission(struct inode *inode, int mask)
|
|
{ return 0; }
|
|
static inline int devcgroup_inode_mknod(int mode, dev_t dev)
|
|
{ return 0; }
|
|
#endif
|