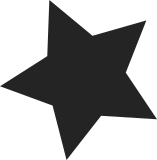
Currently, ARM32 and ARM64 uses different data structures to represent their cpu topologies. Since, we are moving the ARM64 topology to common code to be used by other architectures, we can reuse that for ARM32 as well. Take this opprtunity to remove the redundant functions from ARM32 and reuse the common code instead. To: Russell King <linux@armlinux.org.uk> Signed-off-by: Atish Patra <atish.patra@wdc.com> Tested-by: Sudeep Holla <sudeep.holla@arm.com> (on TC2) Reviewed-by: Sudeep Holla <sudeep.holla@arm.com> Signed-off-by: Paul Walmsley <paul.walmsley@sifive.com>
63 lines
1.6 KiB
C
63 lines
1.6 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/*
|
|
* include/linux/arch_topology.h - arch specific cpu topology information
|
|
*/
|
|
#ifndef _LINUX_ARCH_TOPOLOGY_H_
|
|
#define _LINUX_ARCH_TOPOLOGY_H_
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/percpu.h>
|
|
|
|
void topology_normalize_cpu_scale(void);
|
|
int topology_update_cpu_topology(void);
|
|
|
|
struct device_node;
|
|
bool topology_parse_cpu_capacity(struct device_node *cpu_node, int cpu);
|
|
|
|
DECLARE_PER_CPU(unsigned long, cpu_scale);
|
|
|
|
struct sched_domain;
|
|
static inline
|
|
unsigned long topology_get_cpu_scale(int cpu)
|
|
{
|
|
return per_cpu(cpu_scale, cpu);
|
|
}
|
|
|
|
void topology_set_cpu_scale(unsigned int cpu, unsigned long capacity);
|
|
|
|
DECLARE_PER_CPU(unsigned long, freq_scale);
|
|
|
|
static inline
|
|
unsigned long topology_get_freq_scale(int cpu)
|
|
{
|
|
return per_cpu(freq_scale, cpu);
|
|
}
|
|
|
|
struct cpu_topology {
|
|
int thread_id;
|
|
int core_id;
|
|
int package_id;
|
|
int llc_id;
|
|
cpumask_t thread_sibling;
|
|
cpumask_t core_sibling;
|
|
cpumask_t llc_sibling;
|
|
};
|
|
|
|
#ifdef CONFIG_GENERIC_ARCH_TOPOLOGY
|
|
extern struct cpu_topology cpu_topology[NR_CPUS];
|
|
|
|
#define topology_physical_package_id(cpu) (cpu_topology[cpu].package_id)
|
|
#define topology_core_id(cpu) (cpu_topology[cpu].core_id)
|
|
#define topology_core_cpumask(cpu) (&cpu_topology[cpu].core_sibling)
|
|
#define topology_sibling_cpumask(cpu) (&cpu_topology[cpu].thread_sibling)
|
|
#define topology_llc_cpumask(cpu) (&cpu_topology[cpu].llc_sibling)
|
|
void init_cpu_topology(void);
|
|
void store_cpu_topology(unsigned int cpuid);
|
|
const struct cpumask *cpu_coregroup_mask(int cpu);
|
|
void update_siblings_masks(unsigned int cpu);
|
|
void remove_cpu_topology(unsigned int cpuid);
|
|
void reset_cpu_topology(void);
|
|
#endif
|
|
|
|
#endif /* _LINUX_ARCH_TOPOLOGY_H_ */
|