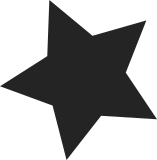
When using device trees on the ARM platform, it is not certain at compile time whether or not the system will have a RTC. If one enables CONFIG_HCTOSYS just in case the system booted has a RTC, and it turns out not to be, this will result in a big fat "unable to open rtc device" error being printed to console, even when "quiet" is set in the kernel cmdline. Fix this by outputting the message with loglevel info instead. Signed-off-by: Floris Bos <bos@je-eigen-domein.nl> Cc: Alessandro Zummo <a.zummo@towertech.it> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
69 lines
1.8 KiB
C
69 lines
1.8 KiB
C
/*
|
|
* RTC subsystem, initialize system time on startup
|
|
*
|
|
* Copyright (C) 2005 Tower Technologies
|
|
* Author: Alessandro Zummo <a.zummo@towertech.it>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
|
|
#include <linux/rtc.h>
|
|
|
|
/* IMPORTANT: the RTC only stores whole seconds. It is arbitrary
|
|
* whether it stores the most close value or the value with partial
|
|
* seconds truncated. However, it is important that we use it to store
|
|
* the truncated value. This is because otherwise it is necessary,
|
|
* in an rtc sync function, to read both xtime.tv_sec and
|
|
* xtime.tv_nsec. On some processors (i.e. ARM), an atomic read
|
|
* of >32bits is not possible. So storing the most close value would
|
|
* slow down the sync API. So here we have the truncated value and
|
|
* the best guess is to add 0.5s.
|
|
*/
|
|
|
|
static int __init rtc_hctosys(void)
|
|
{
|
|
int err = -ENODEV;
|
|
struct rtc_time tm;
|
|
struct timespec64 tv64 = {
|
|
.tv_nsec = NSEC_PER_SEC >> 1,
|
|
};
|
|
struct rtc_device *rtc = rtc_class_open(CONFIG_RTC_HCTOSYS_DEVICE);
|
|
|
|
if (rtc == NULL) {
|
|
pr_info("%s: unable to open rtc device (%s)\n",
|
|
__FILE__, CONFIG_RTC_HCTOSYS_DEVICE);
|
|
goto err_open;
|
|
}
|
|
|
|
err = rtc_read_time(rtc, &tm);
|
|
if (err) {
|
|
dev_err(rtc->dev.parent,
|
|
"hctosys: unable to read the hardware clock\n");
|
|
goto err_read;
|
|
|
|
}
|
|
|
|
tv64.tv_sec = rtc_tm_to_time64(&tm);
|
|
|
|
err = do_settimeofday64(&tv64);
|
|
|
|
dev_info(rtc->dev.parent,
|
|
"setting system clock to "
|
|
"%d-%02d-%02d %02d:%02d:%02d UTC (%lld)\n",
|
|
tm.tm_year + 1900, tm.tm_mon + 1, tm.tm_mday,
|
|
tm.tm_hour, tm.tm_min, tm.tm_sec,
|
|
(long long) tv64.tv_sec);
|
|
|
|
err_read:
|
|
rtc_class_close(rtc);
|
|
|
|
err_open:
|
|
rtc_hctosys_ret = err;
|
|
|
|
return err;
|
|
}
|
|
|
|
late_initcall(rtc_hctosys);
|