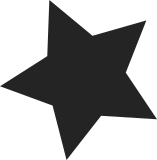
Rename div64_64 to div64_u64 to make it consistent with the other divide functions, so it clearly includes the type of the divide. Move its definition to math64.h as currently no architecture overrides the generic implementation. They can still override it of course, but the duplicated declarations are avoided. Signed-off-by: Roman Zippel <zippel@linux-m68k.org> Cc: Avi Kivity <avi@qumranet.com> Cc: Russell King <rmk@arm.linux.org.uk> Cc: Geert Uytterhoeven <geert@linux-m68k.org> Cc: Ralf Baechle <ralf@linux-mips.org> Cc: David Howells <dhowells@redhat.com> Cc: Jeff Dike <jdike@addtoit.com> Cc: Ingo Molnar <mingo@elte.hu> Cc: "David S. Miller" <davem@davemloft.net> Cc: Patrick McHardy <kaber@trash.net> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
101 lines
2 KiB
C
101 lines
2 KiB
C
/*
|
|
* Copyright (C) 2003 Bernardo Innocenti <bernie@develer.com>
|
|
*
|
|
* Based on former do_div() implementation from asm-parisc/div64.h:
|
|
* Copyright (C) 1999 Hewlett-Packard Co
|
|
* Copyright (C) 1999 David Mosberger-Tang <davidm@hpl.hp.com>
|
|
*
|
|
*
|
|
* Generic C version of 64bit/32bit division and modulo, with
|
|
* 64bit result and 32bit remainder.
|
|
*
|
|
* The fast case for (n>>32 == 0) is handled inline by do_div().
|
|
*
|
|
* Code generated for this function might be very inefficient
|
|
* for some CPUs. __div64_32() can be overridden by linking arch-specific
|
|
* assembly versions such as arch/ppc/lib/div64.S and arch/sh/lib/div64.S.
|
|
*/
|
|
|
|
#include <linux/module.h>
|
|
#include <linux/math64.h>
|
|
|
|
/* Not needed on 64bit architectures */
|
|
#if BITS_PER_LONG == 32
|
|
|
|
uint32_t __attribute__((weak)) __div64_32(uint64_t *n, uint32_t base)
|
|
{
|
|
uint64_t rem = *n;
|
|
uint64_t b = base;
|
|
uint64_t res, d = 1;
|
|
uint32_t high = rem >> 32;
|
|
|
|
/* Reduce the thing a bit first */
|
|
res = 0;
|
|
if (high >= base) {
|
|
high /= base;
|
|
res = (uint64_t) high << 32;
|
|
rem -= (uint64_t) (high*base) << 32;
|
|
}
|
|
|
|
while ((int64_t)b > 0 && b < rem) {
|
|
b = b+b;
|
|
d = d+d;
|
|
}
|
|
|
|
do {
|
|
if (rem >= b) {
|
|
rem -= b;
|
|
res += d;
|
|
}
|
|
b >>= 1;
|
|
d >>= 1;
|
|
} while (d);
|
|
|
|
*n = res;
|
|
return rem;
|
|
}
|
|
|
|
EXPORT_SYMBOL(__div64_32);
|
|
|
|
#ifndef div_s64_rem
|
|
s64 div_s64_rem(s64 dividend, s32 divisor, s32 *remainder)
|
|
{
|
|
u64 quotient;
|
|
|
|
if (dividend < 0) {
|
|
quotient = div_u64_rem(-dividend, abs(divisor), (u32 *)remainder);
|
|
*remainder = -*remainder;
|
|
if (divisor > 0)
|
|
quotient = -quotient;
|
|
} else {
|
|
quotient = div_u64_rem(dividend, abs(divisor), (u32 *)remainder);
|
|
if (divisor < 0)
|
|
quotient = -quotient;
|
|
}
|
|
return quotient;
|
|
}
|
|
EXPORT_SYMBOL(div_s64_rem);
|
|
#endif
|
|
|
|
/* 64bit divisor, dividend and result. dynamic precision */
|
|
#ifndef div64_u64
|
|
u64 div64_u64(u64 dividend, u64 divisor)
|
|
{
|
|
u32 high, d;
|
|
|
|
high = divisor >> 32;
|
|
if (high) {
|
|
unsigned int shift = fls(high);
|
|
|
|
d = divisor >> shift;
|
|
dividend >>= shift;
|
|
} else
|
|
d = divisor;
|
|
|
|
return div_u64(dividend, d);
|
|
}
|
|
EXPORT_SYMBOL(div64_u64);
|
|
#endif
|
|
|
|
#endif /* BITS_PER_LONG == 32 */
|