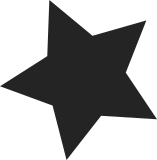
Here is the big char/misc driver update for 4.4-rc1. Lots of different driver and subsystem updates, hwtracing being the largest with the addition of some new platforms that are now supported. Full details in the shortlog. All of these have been in linux-next for a long time with no reported issues. Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org> -----BEGIN PGP SIGNATURE----- Version: GnuPG v2 iEYEABECAAYFAlY6d/oACgkQMUfUDdst+yl93ACcCf91y+ufwU3cmcnq5LpwHPfx VbkAn08Cn6Wu6IcihoEpR4hqGgIOtjqW =1a3d -----END PGP SIGNATURE----- Merge tag 'char-misc-4.4-rc1' of git://git.kernel.org/pub/scm/linux/kernel/git/gregkh/char-misc Pull char/misc driver updates from Greg KH: "Here is the big char/misc driver update for 4.4-rc1. Lots of different driver and subsystem updates, hwtracing being the largest with the addition of some new platforms that are now supported. Full details in the shortlog. All of these have been in linux-next for a long time with no reported issues" * tag 'char-misc-4.4-rc1' of git://git.kernel.org/pub/scm/linux/kernel/git/gregkh/char-misc: (181 commits) fpga: socfpga: Fix check of return value of devm_request_irq lkdtm: fix ACCESS_USERSPACE test mcb: Destroy IDA on module unload mcb: Do not return zero on error path in mcb_pci_probe() mei: bus: set the device name before running fixup mei: bus: use correct lock ordering mei: Fix debugfs filename in error output char: ipmi: ipmi_ssif: Replace timeval with timespec64 fpga: zynq-fpga: Fix issue with drvdata being overwritten. fpga manager: remove unnecessary null pointer checks fpga manager: ensure lifetime with of_fpga_mgr_get fpga: zynq-fpga: Change fw format to handle bin instead of bit. fpga: zynq-fpga: Fix unbalanced clock handling misc: sram: partition base address belongs to __iomem space coresight: etm3x: adding documentation for sysFS's cpu interface vme: 8-bit status/id takes 256 values, not 255 fpga manager: Adding FPGA Manager support for Xilinx Zynq 7000 ARM: zynq: dt: Updated devicetree for Zynq 7000 platform. ARM: dt: fpga: Added binding docs for Xilinx Zynq FPGA manager. ver_linux: proc/modules, limit text processing to 'sed' ...
188 lines
5 KiB
C
188 lines
5 KiB
C
#ifndef _VME_BRIDGE_H_
|
|
#define _VME_BRIDGE_H_
|
|
|
|
#include <linux/vme.h>
|
|
|
|
#define VME_CRCSR_BUF_SIZE (508*1024)
|
|
/*
|
|
* Resource structures
|
|
*/
|
|
struct vme_master_resource {
|
|
struct list_head list;
|
|
struct vme_bridge *parent;
|
|
/*
|
|
* We are likely to need to access the VME bus in interrupt context, so
|
|
* protect master routines with a spinlock rather than a mutex.
|
|
*/
|
|
spinlock_t lock;
|
|
int locked;
|
|
int number;
|
|
u32 address_attr;
|
|
u32 cycle_attr;
|
|
u32 width_attr;
|
|
struct resource bus_resource;
|
|
void __iomem *kern_base;
|
|
};
|
|
|
|
struct vme_slave_resource {
|
|
struct list_head list;
|
|
struct vme_bridge *parent;
|
|
struct mutex mtx;
|
|
int locked;
|
|
int number;
|
|
u32 address_attr;
|
|
u32 cycle_attr;
|
|
};
|
|
|
|
struct vme_dma_pattern {
|
|
u32 pattern;
|
|
u32 type;
|
|
};
|
|
|
|
struct vme_dma_pci {
|
|
dma_addr_t address;
|
|
};
|
|
|
|
struct vme_dma_vme {
|
|
unsigned long long address;
|
|
u32 aspace;
|
|
u32 cycle;
|
|
u32 dwidth;
|
|
};
|
|
|
|
struct vme_dma_list {
|
|
struct list_head list;
|
|
struct vme_dma_resource *parent;
|
|
struct list_head entries;
|
|
struct mutex mtx;
|
|
};
|
|
|
|
struct vme_dma_resource {
|
|
struct list_head list;
|
|
struct vme_bridge *parent;
|
|
struct mutex mtx;
|
|
int locked;
|
|
int number;
|
|
struct list_head pending;
|
|
struct list_head running;
|
|
u32 route_attr;
|
|
};
|
|
|
|
struct vme_lm_resource {
|
|
struct list_head list;
|
|
struct vme_bridge *parent;
|
|
struct mutex mtx;
|
|
int locked;
|
|
int number;
|
|
int monitors;
|
|
};
|
|
|
|
struct vme_error_handler {
|
|
struct list_head list;
|
|
unsigned long long start; /* Beginning of error window */
|
|
unsigned long long end; /* End of error window */
|
|
unsigned long long first_error; /* Address of the first error */
|
|
u32 aspace; /* Address space of error window*/
|
|
unsigned num_errors; /* Number of errors */
|
|
};
|
|
|
|
struct vme_callback {
|
|
void (*func)(int, int, void*);
|
|
void *priv_data;
|
|
};
|
|
|
|
struct vme_irq {
|
|
int count;
|
|
struct vme_callback callback[VME_NUM_STATUSID];
|
|
};
|
|
|
|
/* Allow 16 characters for name (including null character) */
|
|
#define VMENAMSIZ 16
|
|
|
|
/* This structure stores all the information about one bridge
|
|
* The structure should be dynamically allocated by the driver and one instance
|
|
* of the structure should be present for each VME chip present in the system.
|
|
*/
|
|
struct vme_bridge {
|
|
char name[VMENAMSIZ];
|
|
int num;
|
|
struct list_head master_resources;
|
|
struct list_head slave_resources;
|
|
struct list_head dma_resources;
|
|
struct list_head lm_resources;
|
|
|
|
/* List for registered errors handlers */
|
|
struct list_head vme_error_handlers;
|
|
/* List of devices on this bridge */
|
|
struct list_head devices;
|
|
|
|
/* Bridge Info - XXX Move to private structure? */
|
|
struct device *parent; /* Parent device (eg. pdev->dev for PCI) */
|
|
void *driver_priv; /* Private pointer for the bridge driver */
|
|
struct list_head bus_list; /* list of VME buses */
|
|
|
|
/* Interrupt callbacks */
|
|
struct vme_irq irq[7];
|
|
/* Locking for VME irq callback configuration */
|
|
struct mutex irq_mtx;
|
|
|
|
/* Slave Functions */
|
|
int (*slave_get) (struct vme_slave_resource *, int *,
|
|
unsigned long long *, unsigned long long *, dma_addr_t *,
|
|
u32 *, u32 *);
|
|
int (*slave_set) (struct vme_slave_resource *, int, unsigned long long,
|
|
unsigned long long, dma_addr_t, u32, u32);
|
|
|
|
/* Master Functions */
|
|
int (*master_get) (struct vme_master_resource *, int *,
|
|
unsigned long long *, unsigned long long *, u32 *, u32 *,
|
|
u32 *);
|
|
int (*master_set) (struct vme_master_resource *, int,
|
|
unsigned long long, unsigned long long, u32, u32, u32);
|
|
ssize_t (*master_read) (struct vme_master_resource *, void *, size_t,
|
|
loff_t);
|
|
ssize_t (*master_write) (struct vme_master_resource *, void *, size_t,
|
|
loff_t);
|
|
unsigned int (*master_rmw) (struct vme_master_resource *, unsigned int,
|
|
unsigned int, unsigned int, loff_t);
|
|
|
|
/* DMA Functions */
|
|
int (*dma_list_add) (struct vme_dma_list *, struct vme_dma_attr *,
|
|
struct vme_dma_attr *, size_t);
|
|
int (*dma_list_exec) (struct vme_dma_list *);
|
|
int (*dma_list_empty) (struct vme_dma_list *);
|
|
|
|
/* Interrupt Functions */
|
|
void (*irq_set) (struct vme_bridge *, int, int, int);
|
|
int (*irq_generate) (struct vme_bridge *, int, int);
|
|
|
|
/* Location monitor functions */
|
|
int (*lm_set) (struct vme_lm_resource *, unsigned long long, u32, u32);
|
|
int (*lm_get) (struct vme_lm_resource *, unsigned long long *, u32 *,
|
|
u32 *);
|
|
int (*lm_attach) (struct vme_lm_resource *, int, void (*callback)(int));
|
|
int (*lm_detach) (struct vme_lm_resource *, int);
|
|
|
|
/* CR/CSR space functions */
|
|
int (*slot_get) (struct vme_bridge *);
|
|
|
|
/* Bridge parent interface */
|
|
void *(*alloc_consistent)(struct device *dev, size_t size,
|
|
dma_addr_t *dma);
|
|
void (*free_consistent)(struct device *dev, size_t size,
|
|
void *vaddr, dma_addr_t dma);
|
|
};
|
|
|
|
void vme_bus_error_handler(struct vme_bridge *bridge,
|
|
unsigned long long address, int am);
|
|
void vme_irq_handler(struct vme_bridge *, int, int);
|
|
|
|
int vme_register_bridge(struct vme_bridge *);
|
|
void vme_unregister_bridge(struct vme_bridge *);
|
|
struct vme_error_handler *vme_register_error_handler(
|
|
struct vme_bridge *bridge, u32 aspace,
|
|
unsigned long long address, size_t len);
|
|
void vme_unregister_error_handler(struct vme_error_handler *handler);
|
|
|
|
#endif /* _VME_BRIDGE_H_ */
|