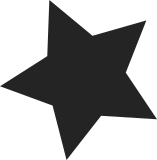
When refactoring and breaking out the includes for the machine-specific GPIO configuration, two files were created in <linux/platform_data/gpio-samsung-s3c[24|64]xx.h>, but as that namespace shall be used for defining data exchanged between machines and drivers, using it for these broad macros and config settings is wrong. Move the headers back into the machine-local <mach/gpio-samsung.h> file and think about the next step. Reported-by: Arnd Bergmann <arnd@arndb.de> Cc: Tomasz Figa <tomasz.figa@gmail.com> Cc: Sylwester Nawrocki <sylvester.nawrocki@gmail.com> Cc: Ben Dooks <ben-linux@fluff.org> Cc: Kukjin Kim <kgene.kim@samsung.com> Cc: linux-samsung-soc@vger.kernel.org Acked-by: Mark Brown <broonie@linaro.org> Acked-by: Arnd Bergmann <arnd@arndb.de> Acked-by: Heiko Stuebner <heiko@sntech.de> Signed-off-by: Linus Walleij <linus.walleij@linaro.org>
72 lines
1.5 KiB
C
72 lines
1.5 KiB
C
/*
|
|
* Copyright (C) 2012 Sylwester Nawrocki <sylvester.nawrocki@gmail.com>
|
|
*
|
|
* Helper functions for S3C24XX/S3C64XX SoC series CAMIF driver
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
|
|
#include <linux/gpio.h>
|
|
#include <plat/gpio-cfg.h>
|
|
#include <mach/gpio-samsung.h>
|
|
|
|
/* Number of camera port pins, without FIELD */
|
|
#define S3C_CAMIF_NUM_GPIOS 13
|
|
|
|
/* Default camera port configuration helpers. */
|
|
|
|
static void camif_get_gpios(int *gpio_start, int *gpio_reset)
|
|
{
|
|
#ifdef CONFIG_ARCH_S3C24XX
|
|
*gpio_start = S3C2410_GPJ(0);
|
|
*gpio_reset = S3C2410_GPJ(12);
|
|
#else
|
|
/* s3c64xx */
|
|
*gpio_start = S3C64XX_GPF(0);
|
|
*gpio_reset = S3C64XX_GPF(3);
|
|
#endif
|
|
}
|
|
|
|
int s3c_camif_gpio_get(void)
|
|
{
|
|
int gpio_start, gpio_reset;
|
|
int ret, i;
|
|
|
|
camif_get_gpios(&gpio_start, &gpio_reset);
|
|
|
|
for (i = 0; i < S3C_CAMIF_NUM_GPIOS; i++) {
|
|
int gpio = gpio_start + i;
|
|
|
|
if (gpio == gpio_reset)
|
|
continue;
|
|
|
|
ret = gpio_request(gpio, "camif");
|
|
if (!ret)
|
|
ret = s3c_gpio_cfgpin(gpio, S3C_GPIO_SFN(2));
|
|
if (ret) {
|
|
pr_err("failed to configure GPIO %d\n", gpio);
|
|
for (--i; i >= 0; i--)
|
|
gpio_free(gpio--);
|
|
return ret;
|
|
}
|
|
s3c_gpio_setpull(gpio, S3C_GPIO_PULL_NONE);
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
void s3c_camif_gpio_put(void)
|
|
{
|
|
int i, gpio_start, gpio_reset;
|
|
|
|
camif_get_gpios(&gpio_start, &gpio_reset);
|
|
|
|
for (i = 0; i < S3C_CAMIF_NUM_GPIOS; i++) {
|
|
int gpio = gpio_start + i;
|
|
if (gpio != gpio_reset)
|
|
gpio_free(gpio);
|
|
}
|
|
}
|