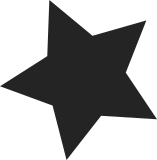
While implementing event__preprocess_sample, that will do all of the symbol lookup in one convenient function, I noticed that util/process_event.[ch] were not being used at all, then started looking if there were other functions that could be shared and... All those functions really don't need to receive offset + head, the only thing they did was common to all of them, so do it at one place instead. Stats about number of each type of event processed now is done in a central place. Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com> Cc: Frédéric Weisbecker <fweisbec@gmail.com> Cc: John Kacur <jkacur@redhat.com> Cc: Mike Galbraith <efault@gmx.de> Cc: Peter Zijlstra <a.p.zijlstra@chello.nl> Cc: Paul Mackerras <paulus@samba.org> LKML-Reference: <1259346563-12568-11-git-send-email-acme@infradead.org> Signed-off-by: Ingo Molnar <mingo@elte.hu>
141 lines
3 KiB
C
141 lines
3 KiB
C
#include "builtin.h"
|
|
|
|
#include "util/util.h"
|
|
#include "util/cache.h"
|
|
#include "util/symbol.h"
|
|
#include "util/thread.h"
|
|
#include "util/header.h"
|
|
|
|
#include "util/parse-options.h"
|
|
|
|
#include "perf.h"
|
|
#include "util/debug.h"
|
|
|
|
#include "util/trace-event.h"
|
|
#include "util/data_map.h"
|
|
|
|
static char const *input_name = "perf.data";
|
|
|
|
static struct perf_header *header;
|
|
static u64 sample_type;
|
|
|
|
static int process_sample_event(event_t *event)
|
|
{
|
|
u64 ip = event->ip.ip;
|
|
u64 timestamp = -1;
|
|
u32 cpu = -1;
|
|
u64 period = 1;
|
|
void *more_data = event->ip.__more_data;
|
|
struct thread *thread = threads__findnew(event->ip.pid);
|
|
|
|
if (sample_type & PERF_SAMPLE_TIME) {
|
|
timestamp = *(u64 *)more_data;
|
|
more_data += sizeof(u64);
|
|
}
|
|
|
|
if (sample_type & PERF_SAMPLE_CPU) {
|
|
cpu = *(u32 *)more_data;
|
|
more_data += sizeof(u32);
|
|
more_data += sizeof(u32); /* reserved */
|
|
}
|
|
|
|
if (sample_type & PERF_SAMPLE_PERIOD) {
|
|
period = *(u64 *)more_data;
|
|
more_data += sizeof(u64);
|
|
}
|
|
|
|
dump_printf("(IP, %d): %d/%d: %p period: %Ld\n",
|
|
event->header.misc,
|
|
event->ip.pid, event->ip.tid,
|
|
(void *)(long)ip,
|
|
(long long)period);
|
|
|
|
if (thread == NULL) {
|
|
pr_debug("problem processing %d event, skipping it.\n",
|
|
event->header.type);
|
|
return -1;
|
|
}
|
|
|
|
dump_printf(" ... thread: %s:%d\n", thread->comm, thread->pid);
|
|
|
|
if (sample_type & PERF_SAMPLE_RAW) {
|
|
struct {
|
|
u32 size;
|
|
char data[0];
|
|
} *raw = more_data;
|
|
|
|
/*
|
|
* FIXME: better resolve from pid from the struct trace_entry
|
|
* field, although it should be the same than this perf
|
|
* event pid
|
|
*/
|
|
print_event(cpu, raw->data, raw->size, timestamp, thread->comm);
|
|
}
|
|
event__stats.total += period;
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int sample_type_check(u64 type)
|
|
{
|
|
sample_type = type;
|
|
|
|
if (!(sample_type & PERF_SAMPLE_RAW)) {
|
|
fprintf(stderr,
|
|
"No trace sample to read. Did you call perf record "
|
|
"without -R?");
|
|
return -1;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
static struct perf_file_handler file_handler = {
|
|
.process_sample_event = process_sample_event,
|
|
.process_comm_event = event__process_comm,
|
|
.sample_type_check = sample_type_check,
|
|
};
|
|
|
|
static int __cmd_trace(void)
|
|
{
|
|
register_idle_thread();
|
|
register_perf_file_handler(&file_handler);
|
|
|
|
return mmap_dispatch_perf_file(&header, input_name,
|
|
0, 0, &event__cwdlen, &event__cwd);
|
|
}
|
|
|
|
static const char * const annotate_usage[] = {
|
|
"perf trace [<options>] <command>",
|
|
NULL
|
|
};
|
|
|
|
static const struct option options[] = {
|
|
OPT_BOOLEAN('D', "dump-raw-trace", &dump_trace,
|
|
"dump raw trace in ASCII"),
|
|
OPT_BOOLEAN('v', "verbose", &verbose,
|
|
"be more verbose (show symbol address, etc)"),
|
|
OPT_BOOLEAN('l', "latency", &latency_format,
|
|
"show latency attributes (irqs/preemption disabled, etc)"),
|
|
OPT_END()
|
|
};
|
|
|
|
int cmd_trace(int argc, const char **argv, const char *prefix __used)
|
|
{
|
|
symbol__init(0);
|
|
|
|
argc = parse_options(argc, argv, options, annotate_usage, 0);
|
|
if (argc) {
|
|
/*
|
|
* Special case: if there's an argument left then assume tha
|
|
* it's a symbol filter:
|
|
*/
|
|
if (argc > 1)
|
|
usage_with_options(annotate_usage, options);
|
|
}
|
|
|
|
setup_pager();
|
|
|
|
return __cmd_trace();
|
|
}
|