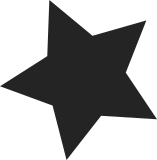
This reverts commit14f0aa3593
. That commit was needed earlier because system call restarting for OABI (compat) required an executable stack and thus had problems with NX. Sinceab72b00734
("ARM: Fix signal restart issues with NX and OABI compat") has reworked the code to not require an executable stack anymore, we can re-enable NX support for kernels with OABI (compat) support. Signed-off-by: Rabin Vincent <rabin@rab.in> Signed-off-by: Russell King <rmk+kernel@arm.linux.org.uk>
84 lines
2 KiB
C
84 lines
2 KiB
C
#include <linux/module.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/personality.h>
|
|
#include <linux/binfmts.h>
|
|
#include <linux/elf.h>
|
|
|
|
int elf_check_arch(const struct elf32_hdr *x)
|
|
{
|
|
unsigned int eflags;
|
|
|
|
/* Make sure it's an ARM executable */
|
|
if (x->e_machine != EM_ARM)
|
|
return 0;
|
|
|
|
/* Make sure the entry address is reasonable */
|
|
if (x->e_entry & 1) {
|
|
if (!(elf_hwcap & HWCAP_THUMB))
|
|
return 0;
|
|
} else if (x->e_entry & 3)
|
|
return 0;
|
|
|
|
eflags = x->e_flags;
|
|
if ((eflags & EF_ARM_EABI_MASK) == EF_ARM_EABI_UNKNOWN) {
|
|
unsigned int flt_fmt;
|
|
|
|
/* APCS26 is only allowed if the CPU supports it */
|
|
if ((eflags & EF_ARM_APCS_26) && !(elf_hwcap & HWCAP_26BIT))
|
|
return 0;
|
|
|
|
flt_fmt = eflags & (EF_ARM_VFP_FLOAT | EF_ARM_SOFT_FLOAT);
|
|
|
|
/* VFP requires the supporting code */
|
|
if (flt_fmt == EF_ARM_VFP_FLOAT && !(elf_hwcap & HWCAP_VFP))
|
|
return 0;
|
|
}
|
|
return 1;
|
|
}
|
|
EXPORT_SYMBOL(elf_check_arch);
|
|
|
|
void elf_set_personality(const struct elf32_hdr *x)
|
|
{
|
|
unsigned int eflags = x->e_flags;
|
|
unsigned int personality = PER_LINUX_32BIT;
|
|
|
|
/*
|
|
* APCS-26 is only valid for OABI executables
|
|
*/
|
|
if ((eflags & EF_ARM_EABI_MASK) == EF_ARM_EABI_UNKNOWN) {
|
|
if (eflags & EF_ARM_APCS_26)
|
|
personality = PER_LINUX;
|
|
}
|
|
|
|
set_personality(personality);
|
|
|
|
/*
|
|
* Since the FPA coprocessor uses CP1 and CP2, and iWMMXt uses CP0
|
|
* and CP1, we only enable access to the iWMMXt coprocessor if the
|
|
* binary is EABI or softfloat (and thus, guaranteed not to use
|
|
* FPA instructions.)
|
|
*/
|
|
if (elf_hwcap & HWCAP_IWMMXT &&
|
|
eflags & (EF_ARM_EABI_MASK | EF_ARM_SOFT_FLOAT)) {
|
|
set_thread_flag(TIF_USING_IWMMXT);
|
|
} else {
|
|
clear_thread_flag(TIF_USING_IWMMXT);
|
|
}
|
|
}
|
|
EXPORT_SYMBOL(elf_set_personality);
|
|
|
|
/*
|
|
* Set READ_IMPLIES_EXEC if:
|
|
* - the binary requires an executable stack
|
|
* - we're running on a CPU which doesn't support NX.
|
|
*/
|
|
int arm_elf_read_implies_exec(const struct elf32_hdr *x, int executable_stack)
|
|
{
|
|
if (executable_stack != EXSTACK_DISABLE_X)
|
|
return 1;
|
|
if (cpu_architecture() < CPU_ARCH_ARMv6)
|
|
return 1;
|
|
return 0;
|
|
}
|
|
EXPORT_SYMBOL(arm_elf_read_implies_exec);
|