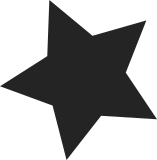
This option can be set to verify the full conversion to the new chip functions. Fix the fallout of the patch rework, so the core code compiles and works with it. Signed-off-by: Thomas Gleixner <tglx@linutronix.de>
69 lines
1.3 KiB
C
69 lines
1.3 KiB
C
/*
|
|
* Copyright (C) 1992, 1998-2006 Linus Torvalds, Ingo Molnar
|
|
* Copyright (C) 2005-2006, Thomas Gleixner, Russell King
|
|
*
|
|
* This file contains the dummy interrupt chip implementation
|
|
*/
|
|
#include <linux/interrupt.h>
|
|
#include <linux/irq.h>
|
|
|
|
#include "internals.h"
|
|
|
|
/*
|
|
* What should we do if we get a hw irq event on an illegal vector?
|
|
* Each architecture has to answer this themself.
|
|
*/
|
|
static void ack_bad(struct irq_data *data)
|
|
{
|
|
struct irq_desc *desc = irq_data_to_desc(data);
|
|
|
|
print_irq_desc(data->irq, desc);
|
|
ack_bad_irq(data->irq);
|
|
}
|
|
|
|
/*
|
|
* NOP functions
|
|
*/
|
|
static void noop(struct irq_data *data) { }
|
|
|
|
static unsigned int noop_ret(struct irq_data *data)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
#ifndef CONFIG_GENERIC_HARDIRQS_NO_DEPRECATED
|
|
static void compat_noop(unsigned int irq) { }
|
|
#define END_INIT .end = compat_noop
|
|
#else
|
|
#define END_INIT
|
|
#endif
|
|
|
|
/*
|
|
* Generic no controller implementation
|
|
*/
|
|
struct irq_chip no_irq_chip = {
|
|
.name = "none",
|
|
.irq_startup = noop_ret,
|
|
.irq_shutdown = noop,
|
|
.irq_enable = noop,
|
|
.irq_disable = noop,
|
|
.irq_ack = ack_bad,
|
|
END_INIT
|
|
};
|
|
|
|
/*
|
|
* Generic dummy implementation which can be used for
|
|
* real dumb interrupt sources
|
|
*/
|
|
struct irq_chip dummy_irq_chip = {
|
|
.name = "dummy",
|
|
.irq_startup = noop_ret,
|
|
.irq_shutdown = noop,
|
|
.irq_enable = noop,
|
|
.irq_disable = noop,
|
|
.irq_ack = noop,
|
|
.irq_mask = noop,
|
|
.irq_unmask = noop,
|
|
END_INIT
|
|
};
|