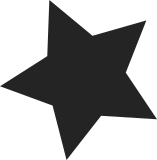
Domain transition so far have been largely blocked by no new privs, unless the transition has been provably a subset of the previous confinement. There was a couple problems with the previous implementations, - transitions that weren't explicitly a stack but resulted in a subset of confinement were disallowed - confinement subsets were only calculated from the previous confinement instead of the confinement being enforced at the time of no new privs, so transitions would have to get progressively tighter. Fix this by detecting and storing a reference to the task's confinement at the "time" no new privs is set. This reference is then used to determine whether a transition is a subsystem of the confinement at the time no new privs was set. Unfortunately the implementation is less than ideal in that we have to detect no new privs after the fact when a task attempts a domain transition. This is adequate for the currently but will not work in a stacking situation where no new privs could be conceivably be set in both the "host" and in the container. Signed-off-by: John Johansen <john.johansen@canonical.com>
95 lines
2.4 KiB
C
95 lines
2.4 KiB
C
/*
|
|
* AppArmor security module
|
|
*
|
|
* This file contains AppArmor task related definitions and mediation
|
|
*
|
|
* Copyright 2017 Canonical Ltd.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation, version 2 of the
|
|
* License.
|
|
*/
|
|
|
|
#ifndef __AA_TASK_H
|
|
#define __AA_TASK_H
|
|
|
|
#define task_ctx(X) ((X)->security)
|
|
|
|
/*
|
|
* struct aa_task_ctx - information for current task label change
|
|
* @nnp: snapshot of label at time of no_new_privs
|
|
* @onexec: profile to transition to on next exec (MAY BE NULL)
|
|
* @previous: profile the task may return to (MAY BE NULL)
|
|
* @token: magic value the task must know for returning to @previous_profile
|
|
*/
|
|
struct aa_task_ctx {
|
|
struct aa_label *nnp;
|
|
struct aa_label *onexec;
|
|
struct aa_label *previous;
|
|
u64 token;
|
|
};
|
|
|
|
int aa_replace_current_label(struct aa_label *label);
|
|
int aa_set_current_onexec(struct aa_label *label, bool stack);
|
|
int aa_set_current_hat(struct aa_label *label, u64 token);
|
|
int aa_restore_previous_label(u64 cookie);
|
|
struct aa_label *aa_get_task_label(struct task_struct *task);
|
|
|
|
/**
|
|
* aa_alloc_task_ctx - allocate a new task_ctx
|
|
* @flags: gfp flags for allocation
|
|
*
|
|
* Returns: allocated buffer or NULL on failure
|
|
*/
|
|
static inline struct aa_task_ctx *aa_alloc_task_ctx(gfp_t flags)
|
|
{
|
|
return kzalloc(sizeof(struct aa_task_ctx), flags);
|
|
}
|
|
|
|
/**
|
|
* aa_free_task_ctx - free a task_ctx
|
|
* @ctx: task_ctx to free (MAYBE NULL)
|
|
*/
|
|
static inline void aa_free_task_ctx(struct aa_task_ctx *ctx)
|
|
{
|
|
if (ctx) {
|
|
aa_put_label(ctx->nnp);
|
|
aa_put_label(ctx->previous);
|
|
aa_put_label(ctx->onexec);
|
|
|
|
kzfree(ctx);
|
|
}
|
|
}
|
|
|
|
/**
|
|
* aa_dup_task_ctx - duplicate a task context, incrementing reference counts
|
|
* @new: a blank task context (NOT NULL)
|
|
* @old: the task context to copy (NOT NULL)
|
|
*/
|
|
static inline void aa_dup_task_ctx(struct aa_task_ctx *new,
|
|
const struct aa_task_ctx *old)
|
|
{
|
|
*new = *old;
|
|
aa_get_label(new->nnp);
|
|
aa_get_label(new->previous);
|
|
aa_get_label(new->onexec);
|
|
}
|
|
|
|
/**
|
|
* aa_clear_task_ctx_trans - clear transition tracking info from the ctx
|
|
* @ctx: task context to clear (NOT NULL)
|
|
*/
|
|
static inline void aa_clear_task_ctx_trans(struct aa_task_ctx *ctx)
|
|
{
|
|
AA_BUG(!ctx);
|
|
|
|
aa_put_label(ctx->previous);
|
|
aa_put_label(ctx->onexec);
|
|
ctx->previous = NULL;
|
|
ctx->onexec = NULL;
|
|
ctx->token = 0;
|
|
}
|
|
|
|
#endif /* __AA_TASK_H */
|