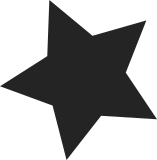
The current SMBus Host Notify implementation relies on .alert() to relay its notifications. However, the use cases where SMBus Host Notify is needed currently is to signal data ready on touchpads. This is closer to an IRQ than a custom API through .alert(). Given that the 2 touchpad manufacturers (Synaptics and Elan) that use SMBus Host Notify don't put any data in the SMBus payload, the concept actually matches one to one. Benefits are multiple: - simpler code and API: the client will just have an IRQ, and nothing needs to be added in the adapter beside internally enabling it. - no more specific workqueue, the threading is handled by IRQ core directly (when required) - no more races when removing the device (the drivers are already required to disable irq on remove) - simpler handling for drivers: use plain regular IRQs - no more dependency on i2c-smbus for i2c-i801 (and any other adapter) - the IRQ domain is created automatically when the adapter exports the Host Notify capability - the IRQ are assign only if ACPI, OF and the caller did not assign one already - the domain is automatically destroyed on remove - fewer lines of code (minus 20, yeah!) Signed-off-by: Benjamin Tissoires <benjamin.tissoires@redhat.com> Signed-off-by: Wolfram Sang <wsa@the-dreams.de>
54 lines
1.8 KiB
C
54 lines
1.8 KiB
C
/*
|
|
* i2c-smbus.h - SMBus extensions to the I2C protocol
|
|
*
|
|
* Copyright (C) 2010 Jean Delvare <jdelvare@suse.de>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston,
|
|
* MA 02110-1301 USA.
|
|
*/
|
|
|
|
#ifndef _LINUX_I2C_SMBUS_H
|
|
#define _LINUX_I2C_SMBUS_H
|
|
|
|
#include <linux/i2c.h>
|
|
#include <linux/spinlock.h>
|
|
#include <linux/workqueue.h>
|
|
|
|
|
|
/**
|
|
* i2c_smbus_alert_setup - platform data for the smbus_alert i2c client
|
|
* @alert_edge_triggered: whether the alert interrupt is edge (1) or level (0)
|
|
* triggered
|
|
* @irq: IRQ number, if the smbus_alert driver should take care of interrupt
|
|
* handling
|
|
*
|
|
* If irq is not specified, the smbus_alert driver doesn't take care of
|
|
* interrupt handling. In that case it is up to the I2C bus driver to either
|
|
* handle the interrupts or to poll for alerts.
|
|
*
|
|
* If irq is specified then it it crucial that alert_edge_triggered is
|
|
* properly set.
|
|
*/
|
|
struct i2c_smbus_alert_setup {
|
|
unsigned int alert_edge_triggered:1;
|
|
int irq;
|
|
};
|
|
|
|
struct i2c_client *i2c_setup_smbus_alert(struct i2c_adapter *adapter,
|
|
struct i2c_smbus_alert_setup *setup);
|
|
int i2c_handle_smbus_alert(struct i2c_client *ara);
|
|
|
|
#endif /* _LINUX_I2C_SMBUS_H */
|