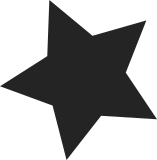
dma_addr_t and phys_addr_t are distinct types and must not be
mixed, as both the values and the size of the type may be
different depending on what the remote device uses.
In this driver the compiler warns when the two types are different:
drivers/remoteproc/mtk_scp.c: In function 'scp_map_memory_region':
drivers/remoteproc/mtk_scp.c:454:9: error: passing argument 3 of 'dma_alloc_coherent' from incompatible pointer type [-Werror=incompatible-pointer-types]
454 | &scp->phys_addr, GFP_KERNEL);
| ^~~~~~~~~~~~~~~
| |
| phys_addr_t * {aka unsigned int *}
In file included from drivers/remoteproc/mtk_scp.c:7:
include/linux/dma-mapping.h:642:15: note: expected 'dma_addr_t *' {aka 'long long unsigned int *'} but argument is of type 'phys_addr_t *' {aka 'unsigned int *'}
642 | dma_addr_t *dma_handle, gfp_t gfp)
Change the phys_addr member to be typed and named according
to how it is allocated.
Fixes: 63c13d61ea
("remoteproc/mediatek: add SCP support for mt8183")
Signed-off-by: Arnd Bergmann <arnd@arndb.de>
Link: https://lore.kernel.org/r/20200408155450.2186471-1-arnd@arndb.de
Signed-off-by: Bjorn Andersson <bjorn.andersson@linaro.org>
95 lines
2.2 KiB
C
95 lines
2.2 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/*
|
|
* Copyright (c) 2019 MediaTek Inc.
|
|
*/
|
|
|
|
#ifndef __RPROC_MTK_COMMON_H
|
|
#define __RPROC_MTK_COMMON_H
|
|
|
|
#include <linux/interrupt.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/platform_device.h>
|
|
#include <linux/remoteproc.h>
|
|
#include <linux/remoteproc/mtk_scp.h>
|
|
|
|
#define MT8183_SW_RSTN 0x0
|
|
#define MT8183_SW_RSTN_BIT BIT(0)
|
|
#define MT8183_SCP_TO_HOST 0x1C
|
|
#define MT8183_SCP_IPC_INT_BIT BIT(0)
|
|
#define MT8183_SCP_WDT_INT_BIT BIT(8)
|
|
#define MT8183_HOST_TO_SCP 0x28
|
|
#define MT8183_HOST_IPC_INT_BIT BIT(0)
|
|
#define MT8183_WDT_CFG 0x84
|
|
#define MT8183_SCP_CLK_SW_SEL 0x4000
|
|
#define MT8183_SCP_CLK_DIV_SEL 0x4024
|
|
#define MT8183_SCP_SRAM_PDN 0x402C
|
|
#define MT8183_SCP_L1_SRAM_PD 0x4080
|
|
#define MT8183_SCP_TCM_TAIL_SRAM_PD 0x4094
|
|
|
|
#define MT8183_SCP_CACHE_SEL(x) (0x14000 + (x) * 0x3000)
|
|
#define MT8183_SCP_CACHE_CON MT8183_SCP_CACHE_SEL(0)
|
|
#define MT8183_SCP_DCACHE_CON MT8183_SCP_CACHE_SEL(1)
|
|
#define MT8183_SCP_CACHESIZE_8KB BIT(8)
|
|
#define MT8183_SCP_CACHE_CON_WAYEN BIT(10)
|
|
|
|
#define SCP_FW_VER_LEN 32
|
|
#define SCP_SHARE_BUFFER_SIZE 288
|
|
|
|
struct scp_run {
|
|
u32 signaled;
|
|
s8 fw_ver[SCP_FW_VER_LEN];
|
|
u32 dec_capability;
|
|
u32 enc_capability;
|
|
wait_queue_head_t wq;
|
|
};
|
|
|
|
struct scp_ipi_desc {
|
|
/* For protecting handler. */
|
|
struct mutex lock;
|
|
scp_ipi_handler_t handler;
|
|
void *priv;
|
|
};
|
|
|
|
struct mtk_scp {
|
|
struct device *dev;
|
|
struct rproc *rproc;
|
|
struct clk *clk;
|
|
void __iomem *reg_base;
|
|
void __iomem *sram_base;
|
|
size_t sram_size;
|
|
|
|
struct mtk_share_obj __iomem *recv_buf;
|
|
struct mtk_share_obj __iomem *send_buf;
|
|
struct scp_run run;
|
|
/* To prevent multiple ipi_send run concurrently. */
|
|
struct mutex send_lock;
|
|
struct scp_ipi_desc ipi_desc[SCP_IPI_MAX];
|
|
bool ipi_id_ack[SCP_IPI_MAX];
|
|
wait_queue_head_t ack_wq;
|
|
|
|
void __iomem *cpu_addr;
|
|
dma_addr_t dma_addr;
|
|
size_t dram_size;
|
|
|
|
struct rproc_subdev *rpmsg_subdev;
|
|
};
|
|
|
|
/**
|
|
* struct mtk_share_obj - SRAM buffer shared with AP and SCP
|
|
*
|
|
* @id: IPI id
|
|
* @len: share buffer length
|
|
* @share_buf: share buffer data
|
|
*/
|
|
struct mtk_share_obj {
|
|
u32 id;
|
|
u32 len;
|
|
u8 share_buf[SCP_SHARE_BUFFER_SIZE];
|
|
};
|
|
|
|
void scp_memcpy_aligned(void __iomem *dst, const void *src, unsigned int len);
|
|
void scp_ipi_lock(struct mtk_scp *scp, u32 id);
|
|
void scp_ipi_unlock(struct mtk_scp *scp, u32 id);
|
|
|
|
#endif
|