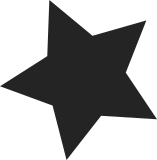
Based on 1 normalized pattern(s): this program is free software you can redistribute it and or modify it under the terms of the gnu general public license as published by the free software foundation either version 2 of the license or at your option any later version this program is distributed in the hope that it will be useful but without any warranty without even the implied warranty of merchantability or fitness for a particular purpose see the gnu general public license for more details you should have received a copy of the gnu general public license along with this program if not write to the free software foundation inc 59 temple place suite 330 boston ma 02111 1307 usa extracted by the scancode license scanner the SPDX license identifier GPL-2.0-or-later has been chosen to replace the boilerplate/reference in 1334 file(s). Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Reviewed-by: Allison Randal <allison@lohutok.net> Reviewed-by: Richard Fontana <rfontana@redhat.com> Cc: linux-spdx@vger.kernel.org Link: https://lkml.kernel.org/r/20190527070033.113240726@linutronix.de Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
128 lines
2.9 KiB
C
128 lines
2.9 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/*
|
|
* Copyright (C) Nokia Corporation
|
|
*
|
|
* Written by Timo Kokkonen <timo.t.kokkonen at nokia.com>
|
|
*/
|
|
|
|
#include <linux/module.h>
|
|
#include <linux/types.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/watchdog.h>
|
|
#include <linux/platform_device.h>
|
|
#include <linux/mfd/twl.h>
|
|
|
|
#define TWL4030_WATCHDOG_CFG_REG_OFFS 0x3
|
|
|
|
static bool nowayout = WATCHDOG_NOWAYOUT;
|
|
module_param(nowayout, bool, 0);
|
|
MODULE_PARM_DESC(nowayout, "Watchdog cannot be stopped once started "
|
|
"(default=" __MODULE_STRING(WATCHDOG_NOWAYOUT) ")");
|
|
|
|
static int twl4030_wdt_write(unsigned char val)
|
|
{
|
|
return twl_i2c_write_u8(TWL_MODULE_PM_RECEIVER, val,
|
|
TWL4030_WATCHDOG_CFG_REG_OFFS);
|
|
}
|
|
|
|
static int twl4030_wdt_start(struct watchdog_device *wdt)
|
|
{
|
|
return twl4030_wdt_write(wdt->timeout + 1);
|
|
}
|
|
|
|
static int twl4030_wdt_stop(struct watchdog_device *wdt)
|
|
{
|
|
return twl4030_wdt_write(0);
|
|
}
|
|
|
|
static int twl4030_wdt_set_timeout(struct watchdog_device *wdt,
|
|
unsigned int timeout)
|
|
{
|
|
wdt->timeout = timeout;
|
|
return 0;
|
|
}
|
|
|
|
static const struct watchdog_info twl4030_wdt_info = {
|
|
.options = WDIOF_SETTIMEOUT | WDIOF_MAGICCLOSE | WDIOF_KEEPALIVEPING,
|
|
.identity = "TWL4030 Watchdog",
|
|
};
|
|
|
|
static const struct watchdog_ops twl4030_wdt_ops = {
|
|
.owner = THIS_MODULE,
|
|
.start = twl4030_wdt_start,
|
|
.stop = twl4030_wdt_stop,
|
|
.set_timeout = twl4030_wdt_set_timeout,
|
|
};
|
|
|
|
static int twl4030_wdt_probe(struct platform_device *pdev)
|
|
{
|
|
struct device *dev = &pdev->dev;
|
|
struct watchdog_device *wdt;
|
|
|
|
wdt = devm_kzalloc(dev, sizeof(*wdt), GFP_KERNEL);
|
|
if (!wdt)
|
|
return -ENOMEM;
|
|
|
|
wdt->info = &twl4030_wdt_info;
|
|
wdt->ops = &twl4030_wdt_ops;
|
|
wdt->status = 0;
|
|
wdt->timeout = 30;
|
|
wdt->min_timeout = 1;
|
|
wdt->max_timeout = 30;
|
|
wdt->parent = dev;
|
|
|
|
watchdog_set_nowayout(wdt, nowayout);
|
|
platform_set_drvdata(pdev, wdt);
|
|
|
|
twl4030_wdt_stop(wdt);
|
|
|
|
return devm_watchdog_register_device(dev, wdt);
|
|
}
|
|
|
|
#ifdef CONFIG_PM
|
|
static int twl4030_wdt_suspend(struct platform_device *pdev, pm_message_t state)
|
|
{
|
|
struct watchdog_device *wdt = platform_get_drvdata(pdev);
|
|
if (watchdog_active(wdt))
|
|
return twl4030_wdt_stop(wdt);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int twl4030_wdt_resume(struct platform_device *pdev)
|
|
{
|
|
struct watchdog_device *wdt = platform_get_drvdata(pdev);
|
|
if (watchdog_active(wdt))
|
|
return twl4030_wdt_start(wdt);
|
|
|
|
return 0;
|
|
}
|
|
#else
|
|
#define twl4030_wdt_suspend NULL
|
|
#define twl4030_wdt_resume NULL
|
|
#endif
|
|
|
|
static const struct of_device_id twl_wdt_of_match[] = {
|
|
{ .compatible = "ti,twl4030-wdt", },
|
|
{ },
|
|
};
|
|
MODULE_DEVICE_TABLE(of, twl_wdt_of_match);
|
|
|
|
static struct platform_driver twl4030_wdt_driver = {
|
|
.probe = twl4030_wdt_probe,
|
|
.suspend = twl4030_wdt_suspend,
|
|
.resume = twl4030_wdt_resume,
|
|
.driver = {
|
|
.name = "twl4030_wdt",
|
|
.of_match_table = twl_wdt_of_match,
|
|
},
|
|
};
|
|
|
|
module_platform_driver(twl4030_wdt_driver);
|
|
|
|
MODULE_AUTHOR("Nokia Corporation");
|
|
MODULE_LICENSE("GPL");
|
|
MODULE_ALIAS("platform:twl4030_wdt");
|
|
|