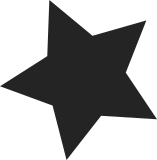
The x86 CPU feature modalias handling existed before it was reimplemented
generically. This patch aligns the x86 handling so that it
(a) reuses some more code that is now generic;
(b) uses the generic format for the modalias module metadata entry, i.e., it
now uses 'cpu:type:x86,venVVVVfamFFFFmodMMMM:feature:,XXXX,YYYY' instead of
the 'x86cpu:vendor:VVVV👪FFFF:model:MMMM:feature:,XXXX,YYYY' that was
used before.
Signed-off-by: Ard Biesheuvel <ard.biesheuvel@linaro.org>
Acked-by: H. Peter Anvin <hpa@linux.intel.com>
Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
50 lines
1.6 KiB
C
50 lines
1.6 KiB
C
#include <asm/cpu_device_id.h>
|
|
#include <asm/processor.h>
|
|
#include <linux/cpu.h>
|
|
#include <linux/module.h>
|
|
#include <linux/slab.h>
|
|
|
|
/**
|
|
* x86_match_cpu - match current CPU again an array of x86_cpu_ids
|
|
* @match: Pointer to array of x86_cpu_ids. Last entry terminated with
|
|
* {}.
|
|
*
|
|
* Return the entry if the current CPU matches the entries in the
|
|
* passed x86_cpu_id match table. Otherwise NULL. The match table
|
|
* contains vendor (X86_VENDOR_*), family, model and feature bits or
|
|
* respective wildcard entries.
|
|
*
|
|
* A typical table entry would be to match a specific CPU
|
|
* { X86_VENDOR_INTEL, 6, 0x12 }
|
|
* or to match a specific CPU feature
|
|
* { X86_FEATURE_MATCH(X86_FEATURE_FOOBAR) }
|
|
*
|
|
* Fields can be wildcarded with %X86_VENDOR_ANY, %X86_FAMILY_ANY,
|
|
* %X86_MODEL_ANY, %X86_FEATURE_ANY or 0 (except for vendor)
|
|
*
|
|
* Arrays used to match for this should also be declared using
|
|
* MODULE_DEVICE_TABLE(x86cpu, ...)
|
|
*
|
|
* This always matches against the boot cpu, assuming models and features are
|
|
* consistent over all CPUs.
|
|
*/
|
|
const struct x86_cpu_id *x86_match_cpu(const struct x86_cpu_id *match)
|
|
{
|
|
const struct x86_cpu_id *m;
|
|
struct cpuinfo_x86 *c = &boot_cpu_data;
|
|
|
|
for (m = match; m->vendor | m->family | m->model | m->feature; m++) {
|
|
if (m->vendor != X86_VENDOR_ANY && c->x86_vendor != m->vendor)
|
|
continue;
|
|
if (m->family != X86_FAMILY_ANY && c->x86 != m->family)
|
|
continue;
|
|
if (m->model != X86_MODEL_ANY && c->x86_model != m->model)
|
|
continue;
|
|
if (m->feature != X86_FEATURE_ANY && !cpu_has(c, m->feature))
|
|
continue;
|
|
return m;
|
|
}
|
|
return NULL;
|
|
}
|
|
EXPORT_SYMBOL(x86_match_cpu);
|