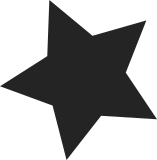
Instead of receiving the num_crts as a parameter, we can read it directly from the mode_config structure. I audited the drivers that invoke this helper and I believe all of them initialize the mode_config struct accordingly, prior to calling the fb_helper. I used the following coccinelle hack to make this transformation, except for the function headers and comment updates. The first and second rules are split because I couldn't find a way to remove the unused temporary variables at the same time I removed the parameter. // <smpl> @r@ expression A,B,D,E; identifier C; @@ ( - drm_fb_helper_init(A,B,C,D) + drm_fb_helper_init(A,B,D) | - drm_fbdev_cma_init_with_funcs(A,B,C,D,E) + drm_fbdev_cma_init_with_funcs(A,B,D,E) | - drm_fbdev_cma_init(A,B,C,D) + drm_fbdev_cma_init(A,B,D) ) @@ expression A,B,C,D,E; @@ ( - drm_fb_helper_init(A,B,C,D) + drm_fb_helper_init(A,B,D) | - drm_fbdev_cma_init_with_funcs(A,B,C,D,E) + drm_fbdev_cma_init_with_funcs(A,B,D,E) | - drm_fbdev_cma_init(A,B,C,D) + drm_fbdev_cma_init(A,B,D) ) @@ identifier r.C; type T; expression V; @@ - T C; <... when != C - C = V; ...> // </smpl> Changes since v1: - Rebased on top of the tip of drm-misc-next. - Remove mention to sti since a proper fix got merged. Suggested-by: Daniel Vetter <daniel.vetter@intel.com> Signed-off-by: Gabriel Krisman Bertazi <krisman@collabora.co.uk> Reviewed-by: Eric Anholt <eric@anholt.net> Signed-off-by: Daniel Vetter <daniel.vetter@ffwll.ch> Link: http://patchwork.freedesktop.org/patch/msgid/20170202162640.27261-1-krisman@collabora.co.uk
53 lines
1.4 KiB
C
53 lines
1.4 KiB
C
/*
|
|
* Copyright (C) 2015 Free Electrons
|
|
* Copyright (C) 2015 NextThing Co
|
|
*
|
|
* Maxime Ripard <maxime.ripard@free-electrons.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of
|
|
* the License, or (at your option) any later version.
|
|
*/
|
|
|
|
#include <drm/drm_atomic_helper.h>
|
|
#include <drm/drm_fb_cma_helper.h>
|
|
#include <drm/drmP.h>
|
|
|
|
#include "sun4i_drv.h"
|
|
#include "sun4i_framebuffer.h"
|
|
|
|
static void sun4i_de_output_poll_changed(struct drm_device *drm)
|
|
{
|
|
struct sun4i_drv *drv = drm->dev_private;
|
|
|
|
drm_fbdev_cma_hotplug_event(drv->fbdev);
|
|
}
|
|
|
|
static const struct drm_mode_config_funcs sun4i_de_mode_config_funcs = {
|
|
.output_poll_changed = sun4i_de_output_poll_changed,
|
|
.atomic_check = drm_atomic_helper_check,
|
|
.atomic_commit = drm_atomic_helper_commit,
|
|
.fb_create = drm_fb_cma_create,
|
|
};
|
|
|
|
struct drm_fbdev_cma *sun4i_framebuffer_init(struct drm_device *drm)
|
|
{
|
|
drm_mode_config_reset(drm);
|
|
|
|
drm->mode_config.max_width = 8192;
|
|
drm->mode_config.max_height = 8192;
|
|
|
|
drm->mode_config.funcs = &sun4i_de_mode_config_funcs;
|
|
|
|
return drm_fbdev_cma_init(drm, 32, drm->mode_config.num_connector);
|
|
}
|
|
|
|
void sun4i_framebuffer_free(struct drm_device *drm)
|
|
{
|
|
struct sun4i_drv *drv = drm->dev_private;
|
|
|
|
drm_fbdev_cma_fini(drv->fbdev);
|
|
drm_mode_config_cleanup(drm);
|
|
}
|