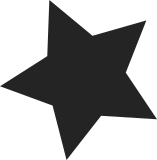
After Al Viro (finally) succeeded in removing the sched.h #include in module.h recently, it makes sense again to remove other superfluous sched.h includes. There are quite a lot of files which include it but don't actually need anything defined in there. Presumably these includes were once needed for macros that used to live in sched.h, but moved to other header files in the course of cleaning it up. To ease the pain, this time I did not fiddle with any header files and only removed #includes from .c-files, which tend to cause less trouble. Compile tested against 2.6.20-rc2 and 2.6.20-rc2-mm2 (with offsets) on alpha, arm, i386, ia64, mips, powerpc, and x86_64 with allnoconfig, defconfig, allmodconfig, and allyesconfig as well as a few randconfigs on x86_64 and all configs in arch/arm/configs on arm. I also checked that no new warnings were introduced by the patch (actually, some warnings are removed that were emitted by unnecessarily included header files). Signed-off-by: Tim Schmielau <tim@physik3.uni-rostock.de> Acked-by: Russell King <rmk+kernel@arm.linux.org.uk> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
143 lines
2.4 KiB
C
143 lines
2.4 KiB
C
/*
|
|
* linux/net/sunrpc/auth_null.c
|
|
*
|
|
* AUTH_NULL authentication. Really :-)
|
|
*
|
|
* Copyright (C) 1996, Olaf Kirch <okir@monad.swb.de>
|
|
*/
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/module.h>
|
|
#include <linux/utsname.h>
|
|
#include <linux/sunrpc/clnt.h>
|
|
|
|
#ifdef RPC_DEBUG
|
|
# define RPCDBG_FACILITY RPCDBG_AUTH
|
|
#endif
|
|
|
|
static struct rpc_auth null_auth;
|
|
static struct rpc_cred null_cred;
|
|
|
|
static struct rpc_auth *
|
|
nul_create(struct rpc_clnt *clnt, rpc_authflavor_t flavor)
|
|
{
|
|
atomic_inc(&null_auth.au_count);
|
|
return &null_auth;
|
|
}
|
|
|
|
static void
|
|
nul_destroy(struct rpc_auth *auth)
|
|
{
|
|
}
|
|
|
|
/*
|
|
* Lookup NULL creds for current process
|
|
*/
|
|
static struct rpc_cred *
|
|
nul_lookup_cred(struct rpc_auth *auth, struct auth_cred *acred, int flags)
|
|
{
|
|
return get_rpccred(&null_cred);
|
|
}
|
|
|
|
/*
|
|
* Destroy cred handle.
|
|
*/
|
|
static void
|
|
nul_destroy_cred(struct rpc_cred *cred)
|
|
{
|
|
}
|
|
|
|
/*
|
|
* Match cred handle against current process
|
|
*/
|
|
static int
|
|
nul_match(struct auth_cred *acred, struct rpc_cred *cred, int taskflags)
|
|
{
|
|
return 1;
|
|
}
|
|
|
|
/*
|
|
* Marshal credential.
|
|
*/
|
|
static __be32 *
|
|
nul_marshal(struct rpc_task *task, __be32 *p)
|
|
{
|
|
*p++ = htonl(RPC_AUTH_NULL);
|
|
*p++ = 0;
|
|
*p++ = htonl(RPC_AUTH_NULL);
|
|
*p++ = 0;
|
|
|
|
return p;
|
|
}
|
|
|
|
/*
|
|
* Refresh credential. This is a no-op for AUTH_NULL
|
|
*/
|
|
static int
|
|
nul_refresh(struct rpc_task *task)
|
|
{
|
|
task->tk_msg.rpc_cred->cr_flags |= RPCAUTH_CRED_UPTODATE;
|
|
return 0;
|
|
}
|
|
|
|
static __be32 *
|
|
nul_validate(struct rpc_task *task, __be32 *p)
|
|
{
|
|
rpc_authflavor_t flavor;
|
|
u32 size;
|
|
|
|
flavor = ntohl(*p++);
|
|
if (flavor != RPC_AUTH_NULL) {
|
|
printk("RPC: bad verf flavor: %u\n", flavor);
|
|
return NULL;
|
|
}
|
|
|
|
size = ntohl(*p++);
|
|
if (size != 0) {
|
|
printk("RPC: bad verf size: %u\n", size);
|
|
return NULL;
|
|
}
|
|
|
|
return p;
|
|
}
|
|
|
|
struct rpc_authops authnull_ops = {
|
|
.owner = THIS_MODULE,
|
|
.au_flavor = RPC_AUTH_NULL,
|
|
#ifdef RPC_DEBUG
|
|
.au_name = "NULL",
|
|
#endif
|
|
.create = nul_create,
|
|
.destroy = nul_destroy,
|
|
.lookup_cred = nul_lookup_cred,
|
|
};
|
|
|
|
static
|
|
struct rpc_auth null_auth = {
|
|
.au_cslack = 4,
|
|
.au_rslack = 2,
|
|
.au_ops = &authnull_ops,
|
|
.au_flavor = RPC_AUTH_NULL,
|
|
.au_count = ATOMIC_INIT(0),
|
|
};
|
|
|
|
static
|
|
struct rpc_credops null_credops = {
|
|
.cr_name = "AUTH_NULL",
|
|
.crdestroy = nul_destroy_cred,
|
|
.crmatch = nul_match,
|
|
.crmarshal = nul_marshal,
|
|
.crrefresh = nul_refresh,
|
|
.crvalidate = nul_validate,
|
|
};
|
|
|
|
static
|
|
struct rpc_cred null_cred = {
|
|
.cr_ops = &null_credops,
|
|
.cr_count = ATOMIC_INIT(1),
|
|
.cr_flags = RPCAUTH_CRED_UPTODATE,
|
|
#ifdef RPC_DEBUG
|
|
.cr_magic = RPCAUTH_CRED_MAGIC,
|
|
#endif
|
|
};
|