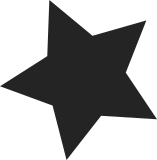
This patch is hdmi display support for exynos drm driver. There is already v4l2 based exynos hdmi driver in drivers/media/video/s5p-tv and some low level code is already in s5p-tv and even headers for register define are almost same. but in this patch, we decide not to consider separated common code with s5p-tv. Exynos HDMI is composed of 5 blocks, mixer, vp, hdmi, hdmiphy and ddc. 1. mixer. The piece of hardware responsible for mixing and blending multiple data inputs before passing it to an output device. The mixer is capable of handling up to three image layers. One is the output of VP. Other two are images in RGB format. The blending factor, and layers' priority are controlled by mixer's registers. The output is passed to HDMI. 2. vp (video processor). It is used for processing of NV12/NV21 data. An image stored in RAM is accessed by DMA. The output in YCbCr444 format is send to mixer. 3. hdmi. The piece of HW responsible for generation of HDMI packets. It takes pixel data from mixer and transforms it into data frames. The output is send to HDMIPHY interface. 4. hdmiphy. Physical interface for HDMI. Its duties are sending HDMI packets to HDMI connector. Basically, it contains a PLL that produces source clock for mixer, vp and hdmi. 5. ddc (display data channel). It is dedicated i2c channel to exchange display information as edid with display monitor. With plane support, exynos hdmi driver fully supports two mixer layes and vp layer. Also vp layer supports multi buffer plane pixel formats having non contigus memory spaces. In exynos drm driver, common drm_hdmi driver to interface with drm framework has opertion pointers for mixer and hdmi. this drm_hdmi driver is registered as sub driver of exynos_drm. hdmi has hdmiphy and ddc i2c clients and controls them. mixer controls all overlay layers in both mixer and vp. Vblank interrupts for hdmi are handled by mixer internally because drm framework cannot support multiple irq id. And pipe number is used to check which display device irq happens. History v2: this version - drm plane feature support to handle overlay layers. - multi buffer plane pixel format support for vp layer. - vp layer support RFCv1: original - at https://lkml.org/lkml/2011/11/4/164 Signed-off-by: Seung-Woo Kim <sw0312.kim@samsung.com> Signed-off-by: Inki Dae <inki.dae@samsung.com> Signed-off-by: Joonyoung Shim <jy0922.shim@samsung.com> Signed-off-by: Kyungmin Park <kyungmin.park@samsung.com>
142 lines
4.5 KiB
C
142 lines
4.5 KiB
C
/*
|
|
*
|
|
* Cloned from drivers/media/video/s5p-tv/regs-mixer.h
|
|
*
|
|
* Copyright (c) 2010-2011 Samsung Electronics Co., Ltd.
|
|
* http://www.samsung.com/
|
|
*
|
|
* Mixer register header file for Samsung Mixer driver
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
#ifndef SAMSUNG_REGS_MIXER_H
|
|
#define SAMSUNG_REGS_MIXER_H
|
|
|
|
/*
|
|
* Register part
|
|
*/
|
|
#define MXR_STATUS 0x0000
|
|
#define MXR_CFG 0x0004
|
|
#define MXR_INT_EN 0x0008
|
|
#define MXR_INT_STATUS 0x000C
|
|
#define MXR_LAYER_CFG 0x0010
|
|
#define MXR_VIDEO_CFG 0x0014
|
|
#define MXR_GRAPHIC0_CFG 0x0020
|
|
#define MXR_GRAPHIC0_BASE 0x0024
|
|
#define MXR_GRAPHIC0_SPAN 0x0028
|
|
#define MXR_GRAPHIC0_SXY 0x002C
|
|
#define MXR_GRAPHIC0_WH 0x0030
|
|
#define MXR_GRAPHIC0_DXY 0x0034
|
|
#define MXR_GRAPHIC0_BLANK 0x0038
|
|
#define MXR_GRAPHIC1_CFG 0x0040
|
|
#define MXR_GRAPHIC1_BASE 0x0044
|
|
#define MXR_GRAPHIC1_SPAN 0x0048
|
|
#define MXR_GRAPHIC1_SXY 0x004C
|
|
#define MXR_GRAPHIC1_WH 0x0050
|
|
#define MXR_GRAPHIC1_DXY 0x0054
|
|
#define MXR_GRAPHIC1_BLANK 0x0058
|
|
#define MXR_BG_CFG 0x0060
|
|
#define MXR_BG_COLOR0 0x0064
|
|
#define MXR_BG_COLOR1 0x0068
|
|
#define MXR_BG_COLOR2 0x006C
|
|
#define MXR_CM_COEFF_Y 0x0080
|
|
#define MXR_CM_COEFF_CB 0x0084
|
|
#define MXR_CM_COEFF_CR 0x0088
|
|
#define MXR_GRAPHIC0_BASE_S 0x2024
|
|
#define MXR_GRAPHIC1_BASE_S 0x2044
|
|
|
|
/* for parametrized access to layer registers */
|
|
#define MXR_GRAPHIC_CFG(i) (0x0020 + (i) * 0x20)
|
|
#define MXR_GRAPHIC_BASE(i) (0x0024 + (i) * 0x20)
|
|
#define MXR_GRAPHIC_SPAN(i) (0x0028 + (i) * 0x20)
|
|
#define MXR_GRAPHIC_SXY(i) (0x002C + (i) * 0x20)
|
|
#define MXR_GRAPHIC_WH(i) (0x0030 + (i) * 0x20)
|
|
#define MXR_GRAPHIC_DXY(i) (0x0034 + (i) * 0x20)
|
|
#define MXR_GRAPHIC_BLANK(i) (0x0038 + (i) * 0x20)
|
|
#define MXR_GRAPHIC_BASE_S(i) (0x2024 + (i) * 0x20)
|
|
|
|
/*
|
|
* Bit definition part
|
|
*/
|
|
|
|
/* generates mask for range of bits */
|
|
#define MXR_MASK(high_bit, low_bit) \
|
|
(((2 << ((high_bit) - (low_bit))) - 1) << (low_bit))
|
|
|
|
#define MXR_MASK_VAL(val, high_bit, low_bit) \
|
|
(((val) << (low_bit)) & MXR_MASK(high_bit, low_bit))
|
|
|
|
/* bits for MXR_STATUS */
|
|
#define MXR_STATUS_16_BURST (1 << 7)
|
|
#define MXR_STATUS_BURST_MASK (1 << 7)
|
|
#define MXR_STATUS_BIG_ENDIAN (1 << 3)
|
|
#define MXR_STATUS_ENDIAN_MASK (1 << 3)
|
|
#define MXR_STATUS_SYNC_ENABLE (1 << 2)
|
|
#define MXR_STATUS_REG_RUN (1 << 0)
|
|
|
|
/* bits for MXR_CFG */
|
|
#define MXR_CFG_RGB601_0_255 (0 << 9)
|
|
#define MXR_CFG_RGB601_16_235 (1 << 9)
|
|
#define MXR_CFG_RGB709_0_255 (2 << 9)
|
|
#define MXR_CFG_RGB709_16_235 (3 << 9)
|
|
#define MXR_CFG_RGB_FMT_MASK 0x600
|
|
#define MXR_CFG_OUT_YUV444 (0 << 8)
|
|
#define MXR_CFG_OUT_RGB888 (1 << 8)
|
|
#define MXR_CFG_OUT_MASK (1 << 8)
|
|
#define MXR_CFG_DST_SDO (0 << 7)
|
|
#define MXR_CFG_DST_HDMI (1 << 7)
|
|
#define MXR_CFG_DST_MASK (1 << 7)
|
|
#define MXR_CFG_SCAN_HD_720 (0 << 6)
|
|
#define MXR_CFG_SCAN_HD_1080 (1 << 6)
|
|
#define MXR_CFG_GRP1_ENABLE (1 << 5)
|
|
#define MXR_CFG_GRP0_ENABLE (1 << 4)
|
|
#define MXR_CFG_VP_ENABLE (1 << 3)
|
|
#define MXR_CFG_SCAN_INTERLACE (0 << 2)
|
|
#define MXR_CFG_SCAN_PROGRASSIVE (1 << 2)
|
|
#define MXR_CFG_SCAN_NTSC (0 << 1)
|
|
#define MXR_CFG_SCAN_PAL (1 << 1)
|
|
#define MXR_CFG_SCAN_SD (0 << 0)
|
|
#define MXR_CFG_SCAN_HD (1 << 0)
|
|
#define MXR_CFG_SCAN_MASK 0x47
|
|
|
|
/* bits for MXR_GRAPHICn_CFG */
|
|
#define MXR_GRP_CFG_COLOR_KEY_DISABLE (1 << 21)
|
|
#define MXR_GRP_CFG_BLEND_PRE_MUL (1 << 20)
|
|
#define MXR_GRP_CFG_WIN_BLEND_EN (1 << 17)
|
|
#define MXR_GRP_CFG_PIXEL_BLEND_EN (1 << 16)
|
|
#define MXR_GRP_CFG_FORMAT_VAL(x) MXR_MASK_VAL(x, 11, 8)
|
|
#define MXR_GRP_CFG_FORMAT_MASK MXR_GRP_CFG_FORMAT_VAL(~0)
|
|
#define MXR_GRP_CFG_ALPHA_VAL(x) MXR_MASK_VAL(x, 7, 0)
|
|
|
|
/* bits for MXR_GRAPHICn_WH */
|
|
#define MXR_GRP_WH_H_SCALE(x) MXR_MASK_VAL(x, 28, 28)
|
|
#define MXR_GRP_WH_V_SCALE(x) MXR_MASK_VAL(x, 12, 12)
|
|
#define MXR_GRP_WH_WIDTH(x) MXR_MASK_VAL(x, 26, 16)
|
|
#define MXR_GRP_WH_HEIGHT(x) MXR_MASK_VAL(x, 10, 0)
|
|
|
|
/* bits for MXR_GRAPHICn_SXY */
|
|
#define MXR_GRP_SXY_SX(x) MXR_MASK_VAL(x, 26, 16)
|
|
#define MXR_GRP_SXY_SY(x) MXR_MASK_VAL(x, 10, 0)
|
|
|
|
/* bits for MXR_GRAPHICn_DXY */
|
|
#define MXR_GRP_DXY_DX(x) MXR_MASK_VAL(x, 26, 16)
|
|
#define MXR_GRP_DXY_DY(x) MXR_MASK_VAL(x, 10, 0)
|
|
|
|
/* bits for MXR_INT_EN */
|
|
#define MXR_INT_EN_VSYNC (1 << 11)
|
|
#define MXR_INT_EN_ALL (0x0f << 8)
|
|
|
|
/* bit for MXR_INT_STATUS */
|
|
#define MXR_INT_CLEAR_VSYNC (1 << 11)
|
|
#define MXR_INT_STATUS_VSYNC (1 << 0)
|
|
|
|
/* bit for MXR_LAYER_CFG */
|
|
#define MXR_LAYER_CFG_GRP1_VAL(x) MXR_MASK_VAL(x, 11, 8)
|
|
#define MXR_LAYER_CFG_GRP0_VAL(x) MXR_MASK_VAL(x, 7, 4)
|
|
#define MXR_LAYER_CFG_VP_VAL(x) MXR_MASK_VAL(x, 3, 0)
|
|
|
|
#endif /* SAMSUNG_REGS_MIXER_H */
|
|
|