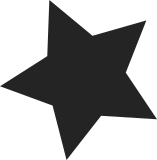
commit 3747069b25e419f6b51395f48127e9812abc3596 upstream.
The __cpuinit type of throwaway sections might have made sense
some time ago when RAM was more constrained, but now the savings
do not offset the cost and complications. For example, the fix in
commit 5e427ec2d0
("x86: Fix bit corruption at CPU resume time")
is a good example of the nasty type of bugs that can be created
with improper use of the various __init prefixes.
After a discussion on LKML[1] it was decided that cpuinit should go
the way of devinit and be phased out. Once all the users are gone,
we can then finally remove the macros themselves from linux/init.h.
Note that some harmless section mismatch warnings may result, since
notify_cpu_starting() and cpu_up() are arch independent (kernel/cpu.c)
and are flagged as __cpuinit -- so if we remove the __cpuinit from
the arch specific callers, we will also get section mismatch warnings.
As an intermediate step, we intend to turn the linux/init.h cpuinit
related content into no-ops as early as possible, since that will get
rid of these warnings. In any case, they are temporary and harmless.
Here, we remove all the MIPS __cpuinit from C code and __CPUINIT
from asm files. MIPS is interesting in this respect, because there
are also uasm users hiding behind their own renamed versions of the
__cpuinit macros.
[1] https://lkml.org/lkml/2013/5/20/589
[ralf@linux-mips.org: Folded in Paul's followup fix.]
Signed-off-by: Paul Gortmaker <paul.gortmaker@windriver.com>
Cc: linux-mips@linux-mips.org
Patchwork: https://patchwork.linux-mips.org/patch/5494/
Patchwork: https://patchwork.linux-mips.org/patch/5495/
Patchwork: https://patchwork.linux-mips.org/patch/5509/
Signed-off-by: Ralf Baechle <ralf@linux-mips.org>
189 lines
4.7 KiB
ArmAsm
189 lines
4.7 KiB
ArmAsm
/*
|
|
* This file is subject to the terms and conditions of the GNU General Public
|
|
* License. See the file "COPYING" in the main directory of this archive
|
|
* for more details.
|
|
*
|
|
* Copyright (C) 1994, 1995 Waldorf Electronics
|
|
* Written by Ralf Baechle and Andreas Busse
|
|
* Copyright (C) 1994 - 99, 2003, 06 Ralf Baechle
|
|
* Copyright (C) 1996 Paul M. Antoine
|
|
* Modified for DECStation and hence R3000 support by Paul M. Antoine
|
|
* Further modifications by David S. Miller and Harald Koerfgen
|
|
* Copyright (C) 1999 Silicon Graphics, Inc.
|
|
* Kevin Kissell, kevink@mips.com and Carsten Langgaard, carstenl@mips.com
|
|
* Copyright (C) 2000 MIPS Technologies, Inc. All rights reserved.
|
|
*/
|
|
#include <linux/init.h>
|
|
#include <linux/threads.h>
|
|
|
|
#include <asm/addrspace.h>
|
|
#include <asm/asm.h>
|
|
#include <asm/asmmacro.h>
|
|
#include <asm/irqflags.h>
|
|
#include <asm/regdef.h>
|
|
#include <asm/pgtable-bits.h>
|
|
#include <asm/mipsregs.h>
|
|
#include <asm/stackframe.h>
|
|
|
|
#include <kernel-entry-init.h>
|
|
|
|
/*
|
|
* For the moment disable interrupts, mark the kernel mode and
|
|
* set ST0_KX so that the CPU does not spit fire when using
|
|
* 64-bit addresses. A full initialization of the CPU's status
|
|
* register is done later in per_cpu_trap_init().
|
|
*/
|
|
.macro setup_c0_status set clr
|
|
.set push
|
|
#ifdef CONFIG_MIPS_MT_SMTC
|
|
/*
|
|
* For SMTC, we need to set privilege and disable interrupts only for
|
|
* the current TC, using the TCStatus register.
|
|
*/
|
|
mfc0 t0, CP0_TCSTATUS
|
|
/* Fortunately CU 0 is in the same place in both registers */
|
|
/* Set TCU0, TMX, TKSU (for later inversion) and IXMT */
|
|
li t1, ST0_CU0 | 0x08001c00
|
|
or t0, t1
|
|
/* Clear TKSU, leave IXMT */
|
|
xori t0, 0x00001800
|
|
mtc0 t0, CP0_TCSTATUS
|
|
_ehb
|
|
/* We need to leave the global IE bit set, but clear EXL...*/
|
|
mfc0 t0, CP0_STATUS
|
|
or t0, ST0_CU0 | ST0_EXL | ST0_ERL | \set | \clr
|
|
xor t0, ST0_EXL | ST0_ERL | \clr
|
|
mtc0 t0, CP0_STATUS
|
|
#else
|
|
mfc0 t0, CP0_STATUS
|
|
or t0, ST0_CU0|\set|0x1f|\clr
|
|
xor t0, 0x1f|\clr
|
|
mtc0 t0, CP0_STATUS
|
|
.set noreorder
|
|
sll zero,3 # ehb
|
|
#endif
|
|
.set pop
|
|
.endm
|
|
|
|
.macro setup_c0_status_pri
|
|
#ifdef CONFIG_64BIT
|
|
setup_c0_status ST0_KX 0
|
|
#else
|
|
setup_c0_status 0 0
|
|
#endif
|
|
.endm
|
|
|
|
.macro setup_c0_status_sec
|
|
#ifdef CONFIG_64BIT
|
|
setup_c0_status ST0_KX ST0_BEV
|
|
#else
|
|
setup_c0_status 0 ST0_BEV
|
|
#endif
|
|
.endm
|
|
|
|
#ifndef CONFIG_NO_EXCEPT_FILL
|
|
/*
|
|
* Reserved space for exception handlers.
|
|
* Necessary for machines which link their kernels at KSEG0.
|
|
*/
|
|
.fill 0x400
|
|
#endif
|
|
|
|
EXPORT(_stext)
|
|
|
|
#ifdef CONFIG_BOOT_RAW
|
|
/*
|
|
* Give us a fighting chance of running if execution beings at the
|
|
* kernel load address. This is needed because this platform does
|
|
* not have a ELF loader yet.
|
|
*/
|
|
FEXPORT(__kernel_entry)
|
|
j kernel_entry
|
|
#endif
|
|
|
|
__REF
|
|
|
|
NESTED(kernel_entry, 16, sp) # kernel entry point
|
|
|
|
kernel_entry_setup # cpu specific setup
|
|
|
|
setup_c0_status_pri
|
|
|
|
/* We might not get launched at the address the kernel is linked to,
|
|
so we jump there. */
|
|
PTR_LA t0, 0f
|
|
jr t0
|
|
0:
|
|
|
|
#ifdef CONFIG_MIPS_MT_SMTC
|
|
/*
|
|
* In SMTC kernel, "CLI" is thread-specific, in TCStatus.
|
|
* We still need to enable interrupts globally in Status,
|
|
* and clear EXL/ERL.
|
|
*
|
|
* TCContext is used to track interrupt levels under
|
|
* service in SMTC kernel. Clear for boot TC before
|
|
* allowing any interrupts.
|
|
*/
|
|
mtc0 zero, CP0_TCCONTEXT
|
|
|
|
mfc0 t0, CP0_STATUS
|
|
ori t0, t0, 0xff1f
|
|
xori t0, t0, 0x001e
|
|
mtc0 t0, CP0_STATUS
|
|
#endif /* CONFIG_MIPS_MT_SMTC */
|
|
|
|
PTR_LA t0, __bss_start # clear .bss
|
|
LONG_S zero, (t0)
|
|
PTR_LA t1, __bss_stop - LONGSIZE
|
|
1:
|
|
PTR_ADDIU t0, LONGSIZE
|
|
LONG_S zero, (t0)
|
|
bne t0, t1, 1b
|
|
|
|
LONG_S a0, fw_arg0 # firmware arguments
|
|
LONG_S a1, fw_arg1
|
|
LONG_S a2, fw_arg2
|
|
LONG_S a3, fw_arg3
|
|
|
|
MTC0 zero, CP0_CONTEXT # clear context register
|
|
PTR_LA $28, init_thread_union
|
|
/* Set the SP after an empty pt_regs. */
|
|
PTR_LI sp, _THREAD_SIZE - 32 - PT_SIZE
|
|
PTR_ADDU sp, $28
|
|
back_to_back_c0_hazard
|
|
set_saved_sp sp, t0, t1
|
|
PTR_SUBU sp, 4 * SZREG # init stack pointer
|
|
|
|
j start_kernel
|
|
END(kernel_entry)
|
|
|
|
#ifdef CONFIG_SMP
|
|
/*
|
|
* SMP slave cpus entry point. Board specific code for bootstrap calls this
|
|
* function after setting up the stack and gp registers.
|
|
*/
|
|
NESTED(smp_bootstrap, 16, sp)
|
|
#ifdef CONFIG_MIPS_MT_SMTC
|
|
/*
|
|
* Read-modify-writes of Status must be atomic, and this
|
|
* is one case where CLI is invoked without EXL being
|
|
* necessarily set. The CLI and setup_c0_status will
|
|
* in fact be redundant for all but the first TC of
|
|
* each VPE being booted.
|
|
*/
|
|
DMT 10 # dmt t2 /* t0, t1 are used by CLI and setup_c0_status() */
|
|
jal mips_ihb
|
|
#endif /* CONFIG_MIPS_MT_SMTC */
|
|
setup_c0_status_sec
|
|
smp_slave_setup
|
|
#ifdef CONFIG_MIPS_MT_SMTC
|
|
andi t2, t2, VPECONTROL_TE
|
|
beqz t2, 2f
|
|
EMT # emt
|
|
2:
|
|
#endif /* CONFIG_MIPS_MT_SMTC */
|
|
j start_secondary
|
|
END(smp_bootstrap)
|
|
#endif /* CONFIG_SMP */
|