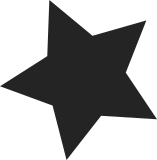
The cgroup filesystem is in the same boat as sysfs. No one ever permits executables of any kind on the cgroup filesystem, and there is no reasonable future case to support executables in the future. Therefore move the setting of SB_I_NOEXEC which makes the code proof against future mistakes of accidentally creating executables from sysfs to kernfs itself. Making the code simpler and covering the sysfs, cgroup, and cgroup2 filesystems. Acked-by: Seth Forshee <seth.forshee@canonical.com> Signed-off-by: "Eric W. Biederman" <ebiederm@xmission.com>
83 lines
1.7 KiB
C
83 lines
1.7 KiB
C
/*
|
|
* fs/sysfs/symlink.c - operations for initializing and mounting sysfs
|
|
*
|
|
* Copyright (c) 2001-3 Patrick Mochel
|
|
* Copyright (c) 2007 SUSE Linux Products GmbH
|
|
* Copyright (c) 2007 Tejun Heo <teheo@suse.de>
|
|
*
|
|
* This file is released under the GPLv2.
|
|
*
|
|
* Please see Documentation/filesystems/sysfs.txt for more information.
|
|
*/
|
|
|
|
#define DEBUG
|
|
|
|
#include <linux/fs.h>
|
|
#include <linux/magic.h>
|
|
#include <linux/mount.h>
|
|
#include <linux/init.h>
|
|
#include <linux/user_namespace.h>
|
|
|
|
#include "sysfs.h"
|
|
|
|
static struct kernfs_root *sysfs_root;
|
|
struct kernfs_node *sysfs_root_kn;
|
|
|
|
static struct dentry *sysfs_mount(struct file_system_type *fs_type,
|
|
int flags, const char *dev_name, void *data)
|
|
{
|
|
struct dentry *root;
|
|
void *ns;
|
|
bool new_sb;
|
|
|
|
if (!(flags & MS_KERNMOUNT)) {
|
|
if (!kobj_ns_current_may_mount(KOBJ_NS_TYPE_NET))
|
|
return ERR_PTR(-EPERM);
|
|
}
|
|
|
|
ns = kobj_ns_grab_current(KOBJ_NS_TYPE_NET);
|
|
root = kernfs_mount_ns(fs_type, flags, sysfs_root,
|
|
SYSFS_MAGIC, &new_sb, ns);
|
|
if (IS_ERR(root) || !new_sb)
|
|
kobj_ns_drop(KOBJ_NS_TYPE_NET, ns);
|
|
else if (new_sb)
|
|
root->d_sb->s_iflags |= SB_I_USERNS_VISIBLE;
|
|
|
|
return root;
|
|
}
|
|
|
|
static void sysfs_kill_sb(struct super_block *sb)
|
|
{
|
|
void *ns = (void *)kernfs_super_ns(sb);
|
|
|
|
kernfs_kill_sb(sb);
|
|
kobj_ns_drop(KOBJ_NS_TYPE_NET, ns);
|
|
}
|
|
|
|
static struct file_system_type sysfs_fs_type = {
|
|
.name = "sysfs",
|
|
.mount = sysfs_mount,
|
|
.kill_sb = sysfs_kill_sb,
|
|
.fs_flags = FS_USERNS_MOUNT,
|
|
};
|
|
|
|
int __init sysfs_init(void)
|
|
{
|
|
int err;
|
|
|
|
sysfs_root = kernfs_create_root(NULL, KERNFS_ROOT_EXTRA_OPEN_PERM_CHECK,
|
|
NULL);
|
|
if (IS_ERR(sysfs_root))
|
|
return PTR_ERR(sysfs_root);
|
|
|
|
sysfs_root_kn = sysfs_root->kn;
|
|
|
|
err = register_filesystem(&sysfs_fs_type);
|
|
if (err) {
|
|
kernfs_destroy_root(sysfs_root);
|
|
return err;
|
|
}
|
|
|
|
return 0;
|
|
}
|