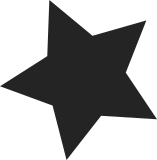
Remove the verbose license text from XFS files and replace them with SPDX tags. This does not change the license of any of the code, merely refers to the common, up-to-date license files in LICENSES/ This change was mostly scripted. fs/xfs/Makefile and fs/xfs/libxfs/xfs_fs.h were modified by hand, the rest were detected and modified by the following command: for f in `git grep -l "GNU General" fs/xfs/` ; do echo $f cat $f | awk -f hdr.awk > $f.new mv -f $f.new $f done And the hdr.awk script that did the modification (including detecting the difference between GPL-2.0 and GPL-2.0+ licenses) is as follows: $ cat hdr.awk BEGIN { hdr = 1.0 tag = "GPL-2.0" str = "" } /^ \* This program is free software/ { hdr = 2.0; next } /any later version./ { tag = "GPL-2.0+" next } /^ \*\// { if (hdr > 0.0) { print "// SPDX-License-Identifier: " tag print str print $0 str="" hdr = 0.0 next } print $0 next } /^ \* / { if (hdr > 1.0) next if (hdr > 0.0) { if (str != "") str = str "\n" str = str $0 next } print $0 next } /^ \*/ { if (hdr > 0.0) next print $0 next } // { if (hdr > 0.0) { if (str != "") str = str "\n" str = str $0 next } print $0 } END { } $ Signed-off-by: Dave Chinner <dchinner@redhat.com> Reviewed-by: Darrick J. Wong <darrick.wong@oracle.com> Signed-off-by: Darrick J. Wong <darrick.wong@oracle.com>
69 lines
1.9 KiB
C
69 lines
1.9 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Copyright (c) 2000-2002,2005 Silicon Graphics, Inc.
|
|
* Copyright (c) 2010 David Chinner.
|
|
* Copyright (c) 2011 Christoph Hellwig.
|
|
* All Rights Reserved.
|
|
*/
|
|
#ifndef __XFS_EXTENT_BUSY_H__
|
|
#define __XFS_EXTENT_BUSY_H__
|
|
|
|
struct xfs_mount;
|
|
struct xfs_trans;
|
|
struct xfs_alloc_arg;
|
|
|
|
/*
|
|
* Busy block/extent entry. Indexed by a rbtree in perag to mark blocks that
|
|
* have been freed but whose transactions aren't committed to disk yet.
|
|
*
|
|
* Note that we use the transaction ID to record the transaction, not the
|
|
* transaction structure itself. See xfs_extent_busy_insert() for details.
|
|
*/
|
|
struct xfs_extent_busy {
|
|
struct rb_node rb_node; /* ag by-bno indexed search tree */
|
|
struct list_head list; /* transaction busy extent list */
|
|
xfs_agnumber_t agno;
|
|
xfs_agblock_t bno;
|
|
xfs_extlen_t length;
|
|
unsigned int flags;
|
|
#define XFS_EXTENT_BUSY_DISCARDED 0x01 /* undergoing a discard op. */
|
|
#define XFS_EXTENT_BUSY_SKIP_DISCARD 0x02 /* do not discard */
|
|
};
|
|
|
|
void
|
|
xfs_extent_busy_insert(struct xfs_trans *tp, xfs_agnumber_t agno,
|
|
xfs_agblock_t bno, xfs_extlen_t len, unsigned int flags);
|
|
|
|
void
|
|
xfs_extent_busy_clear(struct xfs_mount *mp, struct list_head *list,
|
|
bool do_discard);
|
|
|
|
int
|
|
xfs_extent_busy_search(struct xfs_mount *mp, xfs_agnumber_t agno,
|
|
xfs_agblock_t bno, xfs_extlen_t len);
|
|
|
|
void
|
|
xfs_extent_busy_reuse(struct xfs_mount *mp, xfs_agnumber_t agno,
|
|
xfs_agblock_t fbno, xfs_extlen_t flen, bool userdata);
|
|
|
|
bool
|
|
xfs_extent_busy_trim(struct xfs_alloc_arg *args, xfs_agblock_t *bno,
|
|
xfs_extlen_t *len, unsigned *busy_gen);
|
|
|
|
void
|
|
xfs_extent_busy_flush(struct xfs_mount *mp, struct xfs_perag *pag,
|
|
unsigned busy_gen);
|
|
|
|
void
|
|
xfs_extent_busy_wait_all(struct xfs_mount *mp);
|
|
|
|
int
|
|
xfs_extent_busy_ag_cmp(void *priv, struct list_head *a, struct list_head *b);
|
|
|
|
static inline void xfs_extent_busy_sort(struct list_head *list)
|
|
{
|
|
list_sort(NULL, list, xfs_extent_busy_ag_cmp);
|
|
}
|
|
|
|
#endif /* __XFS_EXTENT_BUSY_H__ */
|