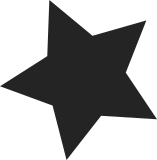
Based on 1 normalized pattern(s): this sctp implementation is free software you can redistribute it and or modify it under the terms of the gnu general public license as published by the free software foundation either version 2 or at your option any later version this sctp implementation is distributed in the hope that it will be useful but without any warranty without even the implied warranty of merchantability or fitness for a particular purpose see the gnu general public license for more details you should have received a copy of the gnu general public license along with gnu cc see the file copying if not see http www gnu org licenses extracted by the scancode license scanner the SPDX license identifier GPL-2.0-or-later has been chosen to replace the boilerplate/reference in 42 file(s). Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Reviewed-by: Kate Stewart <kstewart@linuxfoundation.org> Reviewed-by: Richard Fontana <rfontana@redhat.com> Reviewed-by: Allison Randal <allison@lohutok.net> Cc: linux-spdx@vger.kernel.org Link: https://lkml.kernel.org/r/20190523091649.683323110@linutronix.de Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
106 lines
2.8 KiB
C
106 lines
2.8 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/* SCTP kernel implementation
|
|
* (C) Copyright IBM Corp. 2001, 2004
|
|
*
|
|
* This file is part of the SCTP kernel implementation
|
|
*
|
|
* Support for memory object debugging. This allows one to monitor the
|
|
* object allocations/deallocations for types instrumented for this
|
|
* via the proc fs.
|
|
*
|
|
* Please send any bug reports or fixes you make to the
|
|
* email address(es):
|
|
* lksctp developers <linux-sctp@vger.kernel.org>
|
|
*
|
|
* Written or modified by:
|
|
* Jon Grimm <jgrimm@us.ibm.com>
|
|
*/
|
|
|
|
#define pr_fmt(fmt) KBUILD_MODNAME ": " fmt
|
|
|
|
#include <linux/kernel.h>
|
|
#include <net/sctp/sctp.h>
|
|
|
|
/*
|
|
* Global counters to count raw object allocation counts.
|
|
* To add new counters, choose a unique suffix for the variable
|
|
* name as the helper macros key off this suffix to make
|
|
* life easier for the programmer.
|
|
*/
|
|
|
|
SCTP_DBG_OBJCNT(sock);
|
|
SCTP_DBG_OBJCNT(ep);
|
|
SCTP_DBG_OBJCNT(transport);
|
|
SCTP_DBG_OBJCNT(assoc);
|
|
SCTP_DBG_OBJCNT(bind_addr);
|
|
SCTP_DBG_OBJCNT(bind_bucket);
|
|
SCTP_DBG_OBJCNT(chunk);
|
|
SCTP_DBG_OBJCNT(addr);
|
|
SCTP_DBG_OBJCNT(datamsg);
|
|
SCTP_DBG_OBJCNT(keys);
|
|
|
|
/* An array to make it easy to pretty print the debug information
|
|
* to the proc fs.
|
|
*/
|
|
static struct sctp_dbg_objcnt_entry sctp_dbg_objcnt[] = {
|
|
SCTP_DBG_OBJCNT_ENTRY(sock),
|
|
SCTP_DBG_OBJCNT_ENTRY(ep),
|
|
SCTP_DBG_OBJCNT_ENTRY(assoc),
|
|
SCTP_DBG_OBJCNT_ENTRY(transport),
|
|
SCTP_DBG_OBJCNT_ENTRY(chunk),
|
|
SCTP_DBG_OBJCNT_ENTRY(bind_addr),
|
|
SCTP_DBG_OBJCNT_ENTRY(bind_bucket),
|
|
SCTP_DBG_OBJCNT_ENTRY(addr),
|
|
SCTP_DBG_OBJCNT_ENTRY(datamsg),
|
|
SCTP_DBG_OBJCNT_ENTRY(keys),
|
|
};
|
|
|
|
/* Callback from procfs to read out objcount information.
|
|
* Walk through the entries in the sctp_dbg_objcnt array, dumping
|
|
* the raw object counts for each monitored type.
|
|
*/
|
|
static int sctp_objcnt_seq_show(struct seq_file *seq, void *v)
|
|
{
|
|
int i;
|
|
|
|
i = (int)*(loff_t *)v;
|
|
seq_setwidth(seq, 127);
|
|
seq_printf(seq, "%s: %d", sctp_dbg_objcnt[i].label,
|
|
atomic_read(sctp_dbg_objcnt[i].counter));
|
|
seq_pad(seq, '\n');
|
|
return 0;
|
|
}
|
|
|
|
static void *sctp_objcnt_seq_start(struct seq_file *seq, loff_t *pos)
|
|
{
|
|
return (*pos >= ARRAY_SIZE(sctp_dbg_objcnt)) ? NULL : (void *)pos;
|
|
}
|
|
|
|
static void sctp_objcnt_seq_stop(struct seq_file *seq, void *v)
|
|
{
|
|
}
|
|
|
|
static void *sctp_objcnt_seq_next(struct seq_file *seq, void *v, loff_t *pos)
|
|
{
|
|
++*pos;
|
|
return (*pos >= ARRAY_SIZE(sctp_dbg_objcnt)) ? NULL : (void *)pos;
|
|
}
|
|
|
|
static const struct seq_operations sctp_objcnt_seq_ops = {
|
|
.start = sctp_objcnt_seq_start,
|
|
.next = sctp_objcnt_seq_next,
|
|
.stop = sctp_objcnt_seq_stop,
|
|
.show = sctp_objcnt_seq_show,
|
|
};
|
|
|
|
/* Initialize the objcount in the proc filesystem. */
|
|
void sctp_dbg_objcnt_init(struct net *net)
|
|
{
|
|
struct proc_dir_entry *ent;
|
|
|
|
ent = proc_create_seq("sctp_dbg_objcnt", 0,
|
|
net->sctp.proc_net_sctp, &sctp_objcnt_seq_ops);
|
|
if (!ent)
|
|
pr_warn("sctp_dbg_objcnt: Unable to create /proc entry.\n");
|
|
}
|