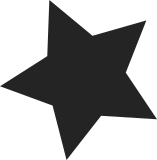
commitbbb03029a8
("strparser: Generalize strparser") added more function pointers to 'struct strp_callbacks'; however, kcm_attach() was not updated to initialize them. This could cause the ->lock() and/or ->unlock() function pointers to be set to garbage values, causing a crash in strp_work(). Fix the bug by moving the callback structs into static memory, so unspecified members are zeroed. Also constify them while we're at it. This bug was found by syzkaller, which encountered the following splat: IP: 0x55 PGD 3b1ca067 P4D 3b1ca067 PUD 3b12f067 PMD 0 Oops: 0010 [#1] SMP KASAN Dumping ftrace buffer: (ftrace buffer empty) Modules linked in: CPU: 2 PID: 1194 Comm: kworker/u8:1 Not tainted 4.13.0-rc4-next-20170811 #2 Hardware name: QEMU Standard PC (i440FX + PIIX, 1996), BIOS Bochs 01/01/2011 Workqueue: kstrp strp_work task: ffff88006bb0e480 task.stack: ffff88006bb10000 RIP: 0010:0x55 RSP: 0018:ffff88006bb17540 EFLAGS: 00010246 RAX: dffffc0000000000 RBX: ffff88006ce4bd60 RCX: 0000000000000000 RDX: 1ffff1000d9c97bd RSI: 0000000000000000 RDI: ffff88006ce4bc48 RBP: ffff88006bb17558 R08: ffffffff81467ab2 R09: 0000000000000000 R10: ffff88006bb17438 R11: ffff88006bb17940 R12: ffff88006ce4bc48 R13: ffff88003c683018 R14: ffff88006bb17980 R15: ffff88003c683000 FS: 0000000000000000(0000) GS:ffff88006de00000(0000) knlGS:0000000000000000 CS: 0010 DS: 0000 ES: 0000 CR0: 0000000080050033 CR2: 0000000000000055 CR3: 000000003c145000 CR4: 00000000000006e0 DR0: 0000000000000000 DR1: 0000000000000000 DR2: 0000000000000000 DR3: 0000000000000000 DR6: 00000000fffe0ff0 DR7: 0000000000000400 Call Trace: process_one_work+0xbf3/0x1bc0 kernel/workqueue.c:2098 worker_thread+0x223/0x1860 kernel/workqueue.c:2233 kthread+0x35e/0x430 kernel/kthread.c:231 ret_from_fork+0x2a/0x40 arch/x86/entry/entry_64.S:431 Code: Bad RIP value. RIP: 0x55 RSP: ffff88006bb17540 CR2: 0000000000000055 ---[ end trace f0e4920047069cee ]--- Here is a C reproducer (requires CONFIG_BPF_SYSCALL=y and CONFIG_AF_KCM=y): #include <linux/bpf.h> #include <linux/kcm.h> #include <linux/types.h> #include <stdint.h> #include <sys/ioctl.h> #include <sys/socket.h> #include <sys/syscall.h> #include <unistd.h> static const struct bpf_insn bpf_insns[3] = { { .code = 0xb7 }, /* BPF_MOV64_IMM(0, 0) */ { .code = 0x95 }, /* BPF_EXIT_INSN() */ }; static const union bpf_attr bpf_attr = { .prog_type = 1, .insn_cnt = 2, .insns = (uintptr_t)&bpf_insns, .license = (uintptr_t)"", }; int main(void) { int bpf_fd = syscall(__NR_bpf, BPF_PROG_LOAD, &bpf_attr, sizeof(bpf_attr)); int inet_fd = socket(AF_INET, SOCK_STREAM, 0); int kcm_fd = socket(AF_KCM, SOCK_DGRAM, 0); ioctl(kcm_fd, SIOCKCMATTACH, &(struct kcm_attach) { .fd = inet_fd, .bpf_fd = bpf_fd }); } Fixes:bbb03029a8
("strparser: Generalize strparser") Cc: Dmitry Vyukov <dvyukov@google.com> Cc: Tom Herbert <tom@quantonium.net> Signed-off-by: Eric Biggers <ebiggers@google.com> Signed-off-by: David S. Miller <davem@davemloft.net>
571 lines
13 KiB
C
571 lines
13 KiB
C
/*
|
|
* Stream Parser
|
|
*
|
|
* Copyright (c) 2016 Tom Herbert <tom@herbertland.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2
|
|
* as published by the Free Software Foundation.
|
|
*/
|
|
|
|
#include <linux/bpf.h>
|
|
#include <linux/errno.h>
|
|
#include <linux/errqueue.h>
|
|
#include <linux/file.h>
|
|
#include <linux/in.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/module.h>
|
|
#include <linux/net.h>
|
|
#include <linux/netdevice.h>
|
|
#include <linux/poll.h>
|
|
#include <linux/rculist.h>
|
|
#include <linux/skbuff.h>
|
|
#include <linux/socket.h>
|
|
#include <linux/uaccess.h>
|
|
#include <linux/workqueue.h>
|
|
#include <net/strparser.h>
|
|
#include <net/netns/generic.h>
|
|
#include <net/sock.h>
|
|
|
|
static struct workqueue_struct *strp_wq;
|
|
|
|
struct _strp_msg {
|
|
/* Internal cb structure. struct strp_msg must be first for passing
|
|
* to upper layer.
|
|
*/
|
|
struct strp_msg strp;
|
|
int accum_len;
|
|
int early_eaten;
|
|
};
|
|
|
|
static inline struct _strp_msg *_strp_msg(struct sk_buff *skb)
|
|
{
|
|
return (struct _strp_msg *)((void *)skb->cb +
|
|
offsetof(struct qdisc_skb_cb, data));
|
|
}
|
|
|
|
/* Lower lock held */
|
|
static void strp_abort_strp(struct strparser *strp, int err)
|
|
{
|
|
/* Unrecoverable error in receive */
|
|
|
|
del_timer(&strp->msg_timer);
|
|
|
|
if (strp->stopped)
|
|
return;
|
|
|
|
strp->stopped = 1;
|
|
|
|
if (strp->sk) {
|
|
struct sock *sk = strp->sk;
|
|
|
|
/* Report an error on the lower socket */
|
|
sk->sk_err = err;
|
|
sk->sk_error_report(sk);
|
|
}
|
|
}
|
|
|
|
static void strp_start_timer(struct strparser *strp, long timeo)
|
|
{
|
|
if (timeo)
|
|
mod_timer(&strp->msg_timer, timeo);
|
|
}
|
|
|
|
/* Lower lock held */
|
|
static void strp_parser_err(struct strparser *strp, int err,
|
|
read_descriptor_t *desc)
|
|
{
|
|
desc->error = err;
|
|
kfree_skb(strp->skb_head);
|
|
strp->skb_head = NULL;
|
|
strp->cb.abort_parser(strp, err);
|
|
}
|
|
|
|
static inline int strp_peek_len(struct strparser *strp)
|
|
{
|
|
if (strp->sk) {
|
|
struct socket *sock = strp->sk->sk_socket;
|
|
|
|
return sock->ops->peek_len(sock);
|
|
}
|
|
|
|
/* If we don't have an associated socket there's nothing to peek.
|
|
* Return int max to avoid stopping the strparser.
|
|
*/
|
|
|
|
return INT_MAX;
|
|
}
|
|
|
|
/* Lower socket lock held */
|
|
static int __strp_recv(read_descriptor_t *desc, struct sk_buff *orig_skb,
|
|
unsigned int orig_offset, size_t orig_len,
|
|
size_t max_msg_size, long timeo)
|
|
{
|
|
struct strparser *strp = (struct strparser *)desc->arg.data;
|
|
struct _strp_msg *stm;
|
|
struct sk_buff *head, *skb;
|
|
size_t eaten = 0, cand_len;
|
|
ssize_t extra;
|
|
int err;
|
|
bool cloned_orig = false;
|
|
|
|
if (strp->paused)
|
|
return 0;
|
|
|
|
head = strp->skb_head;
|
|
if (head) {
|
|
/* Message already in progress */
|
|
|
|
stm = _strp_msg(head);
|
|
if (unlikely(stm->early_eaten)) {
|
|
/* Already some number of bytes on the receive sock
|
|
* data saved in skb_head, just indicate they
|
|
* are consumed.
|
|
*/
|
|
eaten = orig_len <= stm->early_eaten ?
|
|
orig_len : stm->early_eaten;
|
|
stm->early_eaten -= eaten;
|
|
|
|
return eaten;
|
|
}
|
|
|
|
if (unlikely(orig_offset)) {
|
|
/* Getting data with a non-zero offset when a message is
|
|
* in progress is not expected. If it does happen, we
|
|
* need to clone and pull since we can't deal with
|
|
* offsets in the skbs for a message expect in the head.
|
|
*/
|
|
orig_skb = skb_clone(orig_skb, GFP_ATOMIC);
|
|
if (!orig_skb) {
|
|
STRP_STATS_INCR(strp->stats.mem_fail);
|
|
desc->error = -ENOMEM;
|
|
return 0;
|
|
}
|
|
if (!pskb_pull(orig_skb, orig_offset)) {
|
|
STRP_STATS_INCR(strp->stats.mem_fail);
|
|
kfree_skb(orig_skb);
|
|
desc->error = -ENOMEM;
|
|
return 0;
|
|
}
|
|
cloned_orig = true;
|
|
orig_offset = 0;
|
|
}
|
|
|
|
if (!strp->skb_nextp) {
|
|
/* We are going to append to the frags_list of head.
|
|
* Need to unshare the frag_list.
|
|
*/
|
|
err = skb_unclone(head, GFP_ATOMIC);
|
|
if (err) {
|
|
STRP_STATS_INCR(strp->stats.mem_fail);
|
|
desc->error = err;
|
|
return 0;
|
|
}
|
|
|
|
if (unlikely(skb_shinfo(head)->frag_list)) {
|
|
/* We can't append to an sk_buff that already
|
|
* has a frag_list. We create a new head, point
|
|
* the frag_list of that to the old head, and
|
|
* then are able to use the old head->next for
|
|
* appending to the message.
|
|
*/
|
|
if (WARN_ON(head->next)) {
|
|
desc->error = -EINVAL;
|
|
return 0;
|
|
}
|
|
|
|
skb = alloc_skb(0, GFP_ATOMIC);
|
|
if (!skb) {
|
|
STRP_STATS_INCR(strp->stats.mem_fail);
|
|
desc->error = -ENOMEM;
|
|
return 0;
|
|
}
|
|
skb->len = head->len;
|
|
skb->data_len = head->len;
|
|
skb->truesize = head->truesize;
|
|
*_strp_msg(skb) = *_strp_msg(head);
|
|
strp->skb_nextp = &head->next;
|
|
skb_shinfo(skb)->frag_list = head;
|
|
strp->skb_head = skb;
|
|
head = skb;
|
|
} else {
|
|
strp->skb_nextp =
|
|
&skb_shinfo(head)->frag_list;
|
|
}
|
|
}
|
|
}
|
|
|
|
while (eaten < orig_len) {
|
|
/* Always clone since we will consume something */
|
|
skb = skb_clone(orig_skb, GFP_ATOMIC);
|
|
if (!skb) {
|
|
STRP_STATS_INCR(strp->stats.mem_fail);
|
|
desc->error = -ENOMEM;
|
|
break;
|
|
}
|
|
|
|
cand_len = orig_len - eaten;
|
|
|
|
head = strp->skb_head;
|
|
if (!head) {
|
|
head = skb;
|
|
strp->skb_head = head;
|
|
/* Will set skb_nextp on next packet if needed */
|
|
strp->skb_nextp = NULL;
|
|
stm = _strp_msg(head);
|
|
memset(stm, 0, sizeof(*stm));
|
|
stm->strp.offset = orig_offset + eaten;
|
|
} else {
|
|
/* Unclone since we may be appending to an skb that we
|
|
* already share a frag_list with.
|
|
*/
|
|
err = skb_unclone(skb, GFP_ATOMIC);
|
|
if (err) {
|
|
STRP_STATS_INCR(strp->stats.mem_fail);
|
|
desc->error = err;
|
|
break;
|
|
}
|
|
|
|
stm = _strp_msg(head);
|
|
*strp->skb_nextp = skb;
|
|
strp->skb_nextp = &skb->next;
|
|
head->data_len += skb->len;
|
|
head->len += skb->len;
|
|
head->truesize += skb->truesize;
|
|
}
|
|
|
|
if (!stm->strp.full_len) {
|
|
ssize_t len;
|
|
|
|
len = (*strp->cb.parse_msg)(strp, head);
|
|
|
|
if (!len) {
|
|
/* Need more header to determine length */
|
|
if (!stm->accum_len) {
|
|
/* Start RX timer for new message */
|
|
strp_start_timer(strp, timeo);
|
|
}
|
|
stm->accum_len += cand_len;
|
|
eaten += cand_len;
|
|
STRP_STATS_INCR(strp->stats.need_more_hdr);
|
|
WARN_ON(eaten != orig_len);
|
|
break;
|
|
} else if (len < 0) {
|
|
if (len == -ESTRPIPE && stm->accum_len) {
|
|
len = -ENODATA;
|
|
strp->unrecov_intr = 1;
|
|
} else {
|
|
strp->interrupted = 1;
|
|
}
|
|
strp_parser_err(strp, len, desc);
|
|
break;
|
|
} else if (len > max_msg_size) {
|
|
/* Message length exceeds maximum allowed */
|
|
STRP_STATS_INCR(strp->stats.msg_too_big);
|
|
strp_parser_err(strp, -EMSGSIZE, desc);
|
|
break;
|
|
} else if (len <= (ssize_t)head->len -
|
|
skb->len - stm->strp.offset) {
|
|
/* Length must be into new skb (and also
|
|
* greater than zero)
|
|
*/
|
|
STRP_STATS_INCR(strp->stats.bad_hdr_len);
|
|
strp_parser_err(strp, -EPROTO, desc);
|
|
break;
|
|
}
|
|
|
|
stm->strp.full_len = len;
|
|
}
|
|
|
|
extra = (ssize_t)(stm->accum_len + cand_len) -
|
|
stm->strp.full_len;
|
|
|
|
if (extra < 0) {
|
|
/* Message not complete yet. */
|
|
if (stm->strp.full_len - stm->accum_len >
|
|
strp_peek_len(strp)) {
|
|
/* Don't have the whole message in the socket
|
|
* buffer. Set strp->need_bytes to wait for
|
|
* the rest of the message. Also, set "early
|
|
* eaten" since we've already buffered the skb
|
|
* but don't consume yet per strp_read_sock.
|
|
*/
|
|
|
|
if (!stm->accum_len) {
|
|
/* Start RX timer for new message */
|
|
strp_start_timer(strp, timeo);
|
|
}
|
|
|
|
strp->need_bytes = stm->strp.full_len -
|
|
stm->accum_len;
|
|
stm->accum_len += cand_len;
|
|
stm->early_eaten = cand_len;
|
|
STRP_STATS_ADD(strp->stats.bytes, cand_len);
|
|
desc->count = 0; /* Stop reading socket */
|
|
break;
|
|
}
|
|
stm->accum_len += cand_len;
|
|
eaten += cand_len;
|
|
WARN_ON(eaten != orig_len);
|
|
break;
|
|
}
|
|
|
|
/* Positive extra indicates ore bytes than needed for the
|
|
* message
|
|
*/
|
|
|
|
WARN_ON(extra > cand_len);
|
|
|
|
eaten += (cand_len - extra);
|
|
|
|
/* Hurray, we have a new message! */
|
|
del_timer(&strp->msg_timer);
|
|
strp->skb_head = NULL;
|
|
STRP_STATS_INCR(strp->stats.msgs);
|
|
|
|
/* Give skb to upper layer */
|
|
strp->cb.rcv_msg(strp, head);
|
|
|
|
if (unlikely(strp->paused)) {
|
|
/* Upper layer paused strp */
|
|
break;
|
|
}
|
|
}
|
|
|
|
if (cloned_orig)
|
|
kfree_skb(orig_skb);
|
|
|
|
STRP_STATS_ADD(strp->stats.bytes, eaten);
|
|
|
|
return eaten;
|
|
}
|
|
|
|
int strp_process(struct strparser *strp, struct sk_buff *orig_skb,
|
|
unsigned int orig_offset, size_t orig_len,
|
|
size_t max_msg_size, long timeo)
|
|
{
|
|
read_descriptor_t desc; /* Dummy arg to strp_recv */
|
|
|
|
desc.arg.data = strp;
|
|
|
|
return __strp_recv(&desc, orig_skb, orig_offset, orig_len,
|
|
max_msg_size, timeo);
|
|
}
|
|
EXPORT_SYMBOL_GPL(strp_process);
|
|
|
|
static int strp_recv(read_descriptor_t *desc, struct sk_buff *orig_skb,
|
|
unsigned int orig_offset, size_t orig_len)
|
|
{
|
|
struct strparser *strp = (struct strparser *)desc->arg.data;
|
|
|
|
return __strp_recv(desc, orig_skb, orig_offset, orig_len,
|
|
strp->sk->sk_rcvbuf, strp->sk->sk_rcvtimeo);
|
|
}
|
|
|
|
static int default_read_sock_done(struct strparser *strp, int err)
|
|
{
|
|
return err;
|
|
}
|
|
|
|
/* Called with lock held on lower socket */
|
|
static int strp_read_sock(struct strparser *strp)
|
|
{
|
|
struct socket *sock = strp->sk->sk_socket;
|
|
read_descriptor_t desc;
|
|
|
|
if (unlikely(!sock || !sock->ops || !sock->ops->read_sock))
|
|
return -EBUSY;
|
|
|
|
desc.arg.data = strp;
|
|
desc.error = 0;
|
|
desc.count = 1; /* give more than one skb per call */
|
|
|
|
/* sk should be locked here, so okay to do read_sock */
|
|
sock->ops->read_sock(strp->sk, &desc, strp_recv);
|
|
|
|
desc.error = strp->cb.read_sock_done(strp, desc.error);
|
|
|
|
return desc.error;
|
|
}
|
|
|
|
/* Lower sock lock held */
|
|
void strp_data_ready(struct strparser *strp)
|
|
{
|
|
if (unlikely(strp->stopped))
|
|
return;
|
|
|
|
/* This check is needed to synchronize with do_strp_work.
|
|
* do_strp_work acquires a process lock (lock_sock) whereas
|
|
* the lock held here is bh_lock_sock. The two locks can be
|
|
* held by different threads at the same time, but bh_lock_sock
|
|
* allows a thread in BH context to safely check if the process
|
|
* lock is held. In this case, if the lock is held, queue work.
|
|
*/
|
|
if (sock_owned_by_user(strp->sk)) {
|
|
queue_work(strp_wq, &strp->work);
|
|
return;
|
|
}
|
|
|
|
if (strp->paused)
|
|
return;
|
|
|
|
if (strp->need_bytes) {
|
|
if (strp_peek_len(strp) >= strp->need_bytes)
|
|
strp->need_bytes = 0;
|
|
else
|
|
return;
|
|
}
|
|
|
|
if (strp_read_sock(strp) == -ENOMEM)
|
|
queue_work(strp_wq, &strp->work);
|
|
}
|
|
EXPORT_SYMBOL_GPL(strp_data_ready);
|
|
|
|
static void do_strp_work(struct strparser *strp)
|
|
{
|
|
read_descriptor_t rd_desc;
|
|
|
|
/* We need the read lock to synchronize with strp_data_ready. We
|
|
* need the socket lock for calling strp_read_sock.
|
|
*/
|
|
strp->cb.lock(strp);
|
|
|
|
if (unlikely(strp->stopped))
|
|
goto out;
|
|
|
|
if (strp->paused)
|
|
goto out;
|
|
|
|
rd_desc.arg.data = strp;
|
|
|
|
if (strp_read_sock(strp) == -ENOMEM)
|
|
queue_work(strp_wq, &strp->work);
|
|
|
|
out:
|
|
strp->cb.unlock(strp);
|
|
}
|
|
|
|
static void strp_work(struct work_struct *w)
|
|
{
|
|
do_strp_work(container_of(w, struct strparser, work));
|
|
}
|
|
|
|
static void strp_msg_timeout(unsigned long arg)
|
|
{
|
|
struct strparser *strp = (struct strparser *)arg;
|
|
|
|
/* Message assembly timed out */
|
|
STRP_STATS_INCR(strp->stats.msg_timeouts);
|
|
strp->cb.lock(strp);
|
|
strp->cb.abort_parser(strp, ETIMEDOUT);
|
|
strp->cb.unlock(strp);
|
|
}
|
|
|
|
static void strp_sock_lock(struct strparser *strp)
|
|
{
|
|
lock_sock(strp->sk);
|
|
}
|
|
|
|
static void strp_sock_unlock(struct strparser *strp)
|
|
{
|
|
release_sock(strp->sk);
|
|
}
|
|
|
|
int strp_init(struct strparser *strp, struct sock *sk,
|
|
const struct strp_callbacks *cb)
|
|
{
|
|
|
|
if (!cb || !cb->rcv_msg || !cb->parse_msg)
|
|
return -EINVAL;
|
|
|
|
/* The sk (sock) arg determines the mode of the stream parser.
|
|
*
|
|
* If the sock is set then the strparser is in receive callback mode.
|
|
* The upper layer calls strp_data_ready to kick receive processing
|
|
* and strparser calls the read_sock function on the socket to
|
|
* get packets.
|
|
*
|
|
* If the sock is not set then the strparser is in general mode.
|
|
* The upper layer calls strp_process for each skb to be parsed.
|
|
*/
|
|
|
|
if (!sk) {
|
|
if (!cb->lock || !cb->unlock)
|
|
return -EINVAL;
|
|
}
|
|
|
|
memset(strp, 0, sizeof(*strp));
|
|
|
|
strp->sk = sk;
|
|
|
|
strp->cb.lock = cb->lock ? : strp_sock_lock;
|
|
strp->cb.unlock = cb->unlock ? : strp_sock_unlock;
|
|
strp->cb.rcv_msg = cb->rcv_msg;
|
|
strp->cb.parse_msg = cb->parse_msg;
|
|
strp->cb.read_sock_done = cb->read_sock_done ? : default_read_sock_done;
|
|
strp->cb.abort_parser = cb->abort_parser ? : strp_abort_strp;
|
|
|
|
setup_timer(&strp->msg_timer, strp_msg_timeout,
|
|
(unsigned long)strp);
|
|
|
|
INIT_WORK(&strp->work, strp_work);
|
|
|
|
return 0;
|
|
}
|
|
EXPORT_SYMBOL_GPL(strp_init);
|
|
|
|
void strp_unpause(struct strparser *strp)
|
|
{
|
|
strp->paused = 0;
|
|
|
|
/* Sync setting paused with RX work */
|
|
smp_mb();
|
|
|
|
queue_work(strp_wq, &strp->work);
|
|
}
|
|
EXPORT_SYMBOL_GPL(strp_unpause);
|
|
|
|
/* strp must already be stopped so that strp_recv will no longer be called.
|
|
* Note that strp_done is not called with the lower socket held.
|
|
*/
|
|
void strp_done(struct strparser *strp)
|
|
{
|
|
WARN_ON(!strp->stopped);
|
|
|
|
del_timer_sync(&strp->msg_timer);
|
|
cancel_work_sync(&strp->work);
|
|
|
|
if (strp->skb_head) {
|
|
kfree_skb(strp->skb_head);
|
|
strp->skb_head = NULL;
|
|
}
|
|
}
|
|
EXPORT_SYMBOL_GPL(strp_done);
|
|
|
|
void strp_stop(struct strparser *strp)
|
|
{
|
|
strp->stopped = 1;
|
|
}
|
|
EXPORT_SYMBOL_GPL(strp_stop);
|
|
|
|
void strp_check_rcv(struct strparser *strp)
|
|
{
|
|
queue_work(strp_wq, &strp->work);
|
|
}
|
|
EXPORT_SYMBOL_GPL(strp_check_rcv);
|
|
|
|
static int __init strp_mod_init(void)
|
|
{
|
|
strp_wq = create_singlethread_workqueue("kstrp");
|
|
|
|
return 0;
|
|
}
|
|
|
|
static void __exit strp_mod_exit(void)
|
|
{
|
|
destroy_workqueue(strp_wq);
|
|
}
|
|
module_init(strp_mod_init);
|
|
module_exit(strp_mod_exit);
|
|
MODULE_LICENSE("GPL");
|